[C++] Write a program that dynamically allocates an array large enough to hold a user-defined number of test scores. Once all the scores are entered, the array should be passed to a function that sorts them in ascending order. Another function should be called that calculates the average score. The program should display the sorted list of scores and averages with appropriate headings. Use pointer notation rather than array notation whenever possible. Input Validation: Do not accept negative numbers for test scores.
[C++] Write a program that dynamically allocates an array large enough to hold a user-defined number of test scores. Once all the scores are entered, the array should be passed to a function that sorts them in ascending order. Another function should be called that calculates the average score. The program should display the sorted list of scores and averages with appropriate headings. Use pointer notation rather than array notation whenever possible. Input Validation: Do not accept negative numbers for test scores.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Complete the whole code using the all function!.
![int *numPtr = scores;
// Get number of scores
cout << "How many test scores do you have? ";
cin >> numScores;
// Dynamically allocate an array large enough
// to hold that many days of sales amounts
scores = new int[numScores]; // Allocate memory
// Get the sales figures for each day
for (int count = 0; count < numScores; count++)
{
cout << "Test Score #" << (count + 1) << ": ";
cin >> scores[count];
while (scores[count]<1)
{
cout << "Value must be one or greater: ";
cin >> scores[count];
}
}
cout << "The numbers in set are:\n";
for (int count = 0; count < numScores; count++)
cout << *(scores+count) << " "; // Display array
// Calculate the total sales
for (int count = 0; count < numScores; count++)
{
total + scores[count];
}
// Calculate the average
average total / numScores;
// Display the results
cout << "\nAverage Score: " << average;
// Free dynamically allocated memory
delete [] scores;
scores = 0;
return 0;
}
=](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6123bd4b-f483-495c-88a9-ba094fe0819b%2Fc97a1017-0ba4-4736-95fa-40e1190c7650%2F960xn57_processed.jpeg&w=3840&q=75)
Transcribed Image Text:int *numPtr = scores;
// Get number of scores
cout << "How many test scores do you have? ";
cin >> numScores;
// Dynamically allocate an array large enough
// to hold that many days of sales amounts
scores = new int[numScores]; // Allocate memory
// Get the sales figures for each day
for (int count = 0; count < numScores; count++)
{
cout << "Test Score #" << (count + 1) << ": ";
cin >> scores[count];
while (scores[count]<1)
{
cout << "Value must be one or greater: ";
cin >> scores[count];
}
}
cout << "The numbers in set are:\n";
for (int count = 0; count < numScores; count++)
cout << *(scores+count) << " "; // Display array
// Calculate the total sales
for (int count = 0; count < numScores; count++)
{
total + scores[count];
}
// Calculate the average
average total / numScores;
// Display the results
cout << "\nAverage Score: " << average;
// Free dynamically allocated memory
delete [] scores;
scores = 0;
return 0;
}
=
![[C++]
Write a program that dynamically allocates an array
large enough to hold a user-defined number of test
scores. Once all the scores are entered, the array should
be passed to a function that sorts them in ascending
order. Another function should be called that calculates
the average score. The program should display the
sorted list of scores and averages with appropriate
headings. Use pointer notation rather than array
notation whenever possible. Input Validation: Do not
accept negative numbers for test scores.
<<Please help to add code to sort test scores in
ascendent order for "The numbers in set are: \n">>
(Current Code)
#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
int *scores, // To dynamically allocate an array
total = 0.0, // Accumulator
average; // To hold average scores
int numScores; // To hold number of scores
int *numPtr = scores;
// Get number of scores
cout << "How many test scores do you have? ";
cin >> numScores;
// Dynamically allocate an array large enough
// to hold that many days of sales amounts
scores = new int[numScores]; // Allocate memory
// Get the sales figures for each day
for (int count = 0; count < numScores; count++)
{
cout << "Test Score #" << (count + 1) << ": ";
cin >> scores[count];
while (scores[count]<1)
{
cout << "Value must be one or greater: ";](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6123bd4b-f483-495c-88a9-ba094fe0819b%2Fc97a1017-0ba4-4736-95fa-40e1190c7650%2Fq1ny1nv_processed.jpeg&w=3840&q=75)
Transcribed Image Text:[C++]
Write a program that dynamically allocates an array
large enough to hold a user-defined number of test
scores. Once all the scores are entered, the array should
be passed to a function that sorts them in ascending
order. Another function should be called that calculates
the average score. The program should display the
sorted list of scores and averages with appropriate
headings. Use pointer notation rather than array
notation whenever possible. Input Validation: Do not
accept negative numbers for test scores.
<<Please help to add code to sort test scores in
ascendent order for "The numbers in set are: \n">>
(Current Code)
#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
int *scores, // To dynamically allocate an array
total = 0.0, // Accumulator
average; // To hold average scores
int numScores; // To hold number of scores
int *numPtr = scores;
// Get number of scores
cout << "How many test scores do you have? ";
cin >> numScores;
// Dynamically allocate an array large enough
// to hold that many days of sales amounts
scores = new int[numScores]; // Allocate memory
// Get the sales figures for each day
for (int count = 0; count < numScores; count++)
{
cout << "Test Score #" << (count + 1) << ": ";
cin >> scores[count];
while (scores[count]<1)
{
cout << "Value must be one or greater: ";
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Recommended textbooks for you
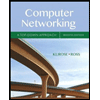
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
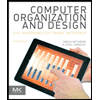
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
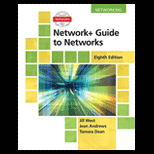
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
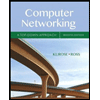
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
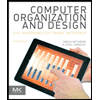
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
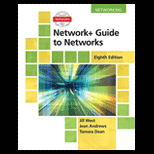
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
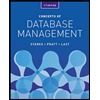
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
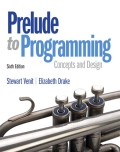
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
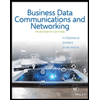
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY