Caesar's Cipher is an algorithm that substitutes each letter of the alphabet by shifting the letter by a given key value. For example, a key of 3 would replace A with D, B with E, and so on. As described in https://www.youtube.com/watch?v=6-JiHa-qLPk, it is fairly easy to break an encrypted message if all letters are shifted by the same number. An improved approach is to use a multiple-digit key that would shift each letter in a document by a different amount. The following screenshot from the video shows how a 10-digit key 5182273847 can encrypt "FREE PIZZA IN THE CAFETERIA" into "KSMG RPCHE PS UPG EHIMXLWJI". The first letter F is shifted by 5 and replaced with K. The second letter R is shifted by 1 and replaced with S. The third letter E is shifted by 8 and replaced with M. And so on. Note that the video and the following screenshot from the video did not convert the last three letters properly. The result should be "WJI" instead of "SJB". . IQKE RLF KISMIG L T NIH MIUIO 1 +5 +1 +8 +2 PNAFC NQ QOBG D O R PCHE P SQD 1 F Q T TRE JG RU +7 +5 +2 +7 +3 +8 +4 As we know, a string is a sequence (array) of characters. The circular effect for this improved Caesar's Cipher comes in two forms. O The first circular form applies to a string of digits as the key. In the above example, as we move down the message, we move down the key string. That is, we use 5 as the key for encripting the first letter 'F', 1 as the key for encripting the second letter 'R', and so on. When we get to the 10th letter 'I', 7 is used as a key. After that, for the 11th letter 'N', we circle back to the beginning of the key string and use '5' as the key to encript. The second circular form applies to a string of all alphabets. const string CaesarCipher::ALPHABET = When the "shifting" does not go past the last letter, no circular effect. For example, ● If the letter to be encripted is F and the key is 5, the encripted letter would be K. If the letter to be encripted is E and the key is 2, the encripted letter would be G. ● ● SNE O F U P G V H WR +1 +8 +2 . When the "shifting" go past the last letter, it circular back to the beginning of the alphabet. For example, ● CF|GK|VJ|QH|Z D GHLWKR 1 A E H 1 MIXIL S J B F 1 J N Y M TKIC GJKOZNUL +2 +7 +3 +8 +4 +7+5 +1 +8 5182273847 ● In this lab, you are asked to implement and test the Ciper and Decipher member functions of the CaesarCipher class. We will focus on only the UPPERCASE LETTERS. "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; string cipher (const string&) const; This function takes a string as its parameter and returns an encrypted string by repeatedly applying the appropriate shifting according to the key. For example, in the following code segment, "CJVYB" would be returned by the cipher function. CaesarCipher demo ("35"); cout << demo.cipher("ZESTY") << endl; If the letter to be encripted is T and the key is 7, the encripted letter would be A. If the letter to be encripted is Z and the key is 3, the encripted letter would be C. Z would be encripted into a letter in ALPHABET that is 3 spaces forward, which is C. Note the circular effect of starting from the letter A in the shifting process. E would be encripted into by a letter in ALPHABET that is 5 spaces forward, which is J. S would be encripted into by a letter in ALPHABET that is 3 spaces forward, which is V. Note the circular effect of using 3 as the key after both 3 and 5 have been used. T would be encripted into by a letter in ALPHABET that is 5 spaces forward, which is Y.
Caesar's Cipher is an algorithm that substitutes each letter of the alphabet by shifting the letter by a given key value. For example, a key of 3 would replace A with D, B with E, and so on. As described in https://www.youtube.com/watch?v=6-JiHa-qLPk, it is fairly easy to break an encrypted message if all letters are shifted by the same number. An improved approach is to use a multiple-digit key that would shift each letter in a document by a different amount. The following screenshot from the video shows how a 10-digit key 5182273847 can encrypt "FREE PIZZA IN THE CAFETERIA" into "KSMG RPCHE PS UPG EHIMXLWJI". The first letter F is shifted by 5 and replaced with K. The second letter R is shifted by 1 and replaced with S. The third letter E is shifted by 8 and replaced with M. And so on. Note that the video and the following screenshot from the video did not convert the last three letters properly. The result should be "WJI" instead of "SJB". . IQKE RLF KISMIG L T NIH MIUIO 1 +5 +1 +8 +2 PNAFC NQ QOBG D O R PCHE P SQD 1 F Q T TRE JG RU +7 +5 +2 +7 +3 +8 +4 As we know, a string is a sequence (array) of characters. The circular effect for this improved Caesar's Cipher comes in two forms. O The first circular form applies to a string of digits as the key. In the above example, as we move down the message, we move down the key string. That is, we use 5 as the key for encripting the first letter 'F', 1 as the key for encripting the second letter 'R', and so on. When we get to the 10th letter 'I', 7 is used as a key. After that, for the 11th letter 'N', we circle back to the beginning of the key string and use '5' as the key to encript. The second circular form applies to a string of all alphabets. const string CaesarCipher::ALPHABET = When the "shifting" does not go past the last letter, no circular effect. For example, ● If the letter to be encripted is F and the key is 5, the encripted letter would be K. If the letter to be encripted is E and the key is 2, the encripted letter would be G. ● ● SNE O F U P G V H WR +1 +8 +2 . When the "shifting" go past the last letter, it circular back to the beginning of the alphabet. For example, ● CF|GK|VJ|QH|Z D GHLWKR 1 A E H 1 MIXIL S J B F 1 J N Y M TKIC GJKOZNUL +2 +7 +3 +8 +4 +7+5 +1 +8 5182273847 ● In this lab, you are asked to implement and test the Ciper and Decipher member functions of the CaesarCipher class. We will focus on only the UPPERCASE LETTERS. "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; string cipher (const string&) const; This function takes a string as its parameter and returns an encrypted string by repeatedly applying the appropriate shifting according to the key. For example, in the following code segment, "CJVYB" would be returned by the cipher function. CaesarCipher demo ("35"); cout << demo.cipher("ZESTY") << endl; If the letter to be encripted is T and the key is 7, the encripted letter would be A. If the letter to be encripted is Z and the key is 3, the encripted letter would be C. Z would be encripted into a letter in ALPHABET that is 3 spaces forward, which is C. Note the circular effect of starting from the letter A in the shifting process. E would be encripted into by a letter in ALPHABET that is 5 spaces forward, which is J. S would be encripted into by a letter in ALPHABET that is 3 spaces forward, which is V. Note the circular effect of using 3 as the key after both 3 and 5 have been used. T would be encripted into by a letter in ALPHABET that is 5 spaces forward, which is Y.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Please help me in C++ I just need help with my ceasar cipher and decipher function please help
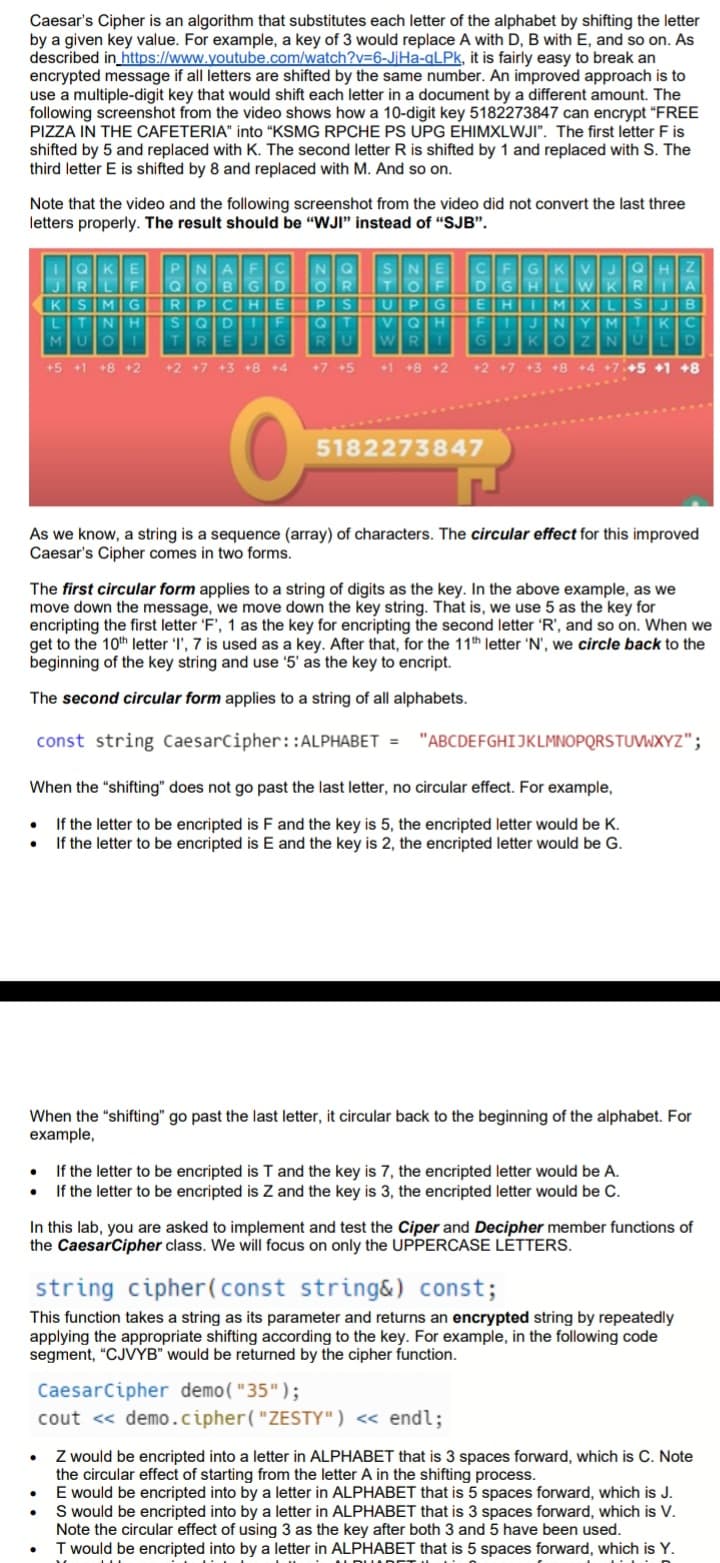
Transcribed Image Text:Caesar's Cipher is an algorithm that substitutes each letter of the alphabet by shifting the letter
by a given key value. For example, a key of 3 would replace A with D, B with E, and so on. As
described in https://www.youtube.com/watch?v=6-JjHa-qLPk, it is fairly easy to break an
encrypted message if all letters are shifted by the same number. An improved approach is to
use a multiple-digit key that would shift each letter in a document by a different amount. The
following screenshot from the video shows how a 10-digit key 5182273847 can encrypt "FREE
PIZZA IN THE CAFETERIA" into "KSMG RPCHE PS UPG EHIMXLWJI". The first letter F is
shifted by 5 and replaced with K. The second letter R is shifted by 1 and replaced with S. The
third letter E is shifted by 8 and replaced with M. And so on.
Note that the video and the following screenshot from the video did not convert the last three
letters properly. The result should be "WJI" instead of "SJB".
●
IQKE
RL
KSMG
L T ΝΤΗ
MUO
UOI
+5 +1 +8 +2
PNAFC NQ SNE
QOBGD OR
RPCHE
SQD 1 F
TREJG
+2 +7 +3 +8 +4
●
P S
Q
RU
+7 +5
CFGKVJQHZ
DGH
WKR
E HII MIXILIS
F 1 J N YIM
GJKOZNUL
WR
+1 +8 +2 +2 +7 +3 +8 +4 +7+5 +1 +8
O
.
●
U P G
Q H
As we know, a string is a sequence (array) of characters. The circular effect for this improved
Caesar's Cipher comes in two forms.
●
5182273847
The first circular form applies to a string of digits as the key. In the above example, as we
move down the message, we move down the key string. That is, we use 5 as the key for
encripting the first letter 'F', 1 as the key for encripting the second letter 'R', and so on. When we
get to the 10th letter 'I', 7 is used as a key. After that, for the 11th letter 'N', we circle back to the
beginning of the key string and use '5' as the key to encript.
The second circular form applies to a string of all alphabets.
const string CaesarCipher::ALPHABET =
When the "shifting" does not go past the last letter, no circular effect. For example,
● If the letter to be encripted is F and the key is 5, the encripted letter would be K.
• If the letter to be encripted is E and the key is 2, the encripted letter would be G.
GHLW
When the "shifting" go past the last letter, it circular back to the beginning of the alphabet. For
example,
LISIJIB
MTKC
In this lab, you are asked to implement and test the Ciper and Decipher member functions of
the Caesar Cipher class. We will focus on only the UPPERCASE LETTERS.
string cipher (const string&) const;
This function takes a string as its parameter and returns an encrypted string by repeatedly
applying the appropriate shifting according to the key. For example, in the following code
segment, "CJVYB" would be returned by the cipher function.
CaesarCipher demo("35");
cout << demo.cipher("ZESTY") << endl;
A
"ABCDEFGHIJKLMNOPQRSTUVWXYZ";
If the letter to be encripted is T and the key is 7, the encripted letter would be A.
If the letter to be encripted is Z and the key is 3, the encripted letter would be C.
Z would be encripted into a letter in ALPHABET that is 3 spaces forward, which is C. Note
the circular effect of starting from the letter A in the shifting process.
E would be encripted into by a letter in ALPHABET that is 5 spaces forward, which is J.
S would be encripted into by a letter in ALPHABET that is 3 spaces forward, which is V.
Note the circular effect of using 3 as the key after both 3 and 5 have been used.
T would be encripted into by a letter in ALPHABET that is 5 spaces forward, which is Y.
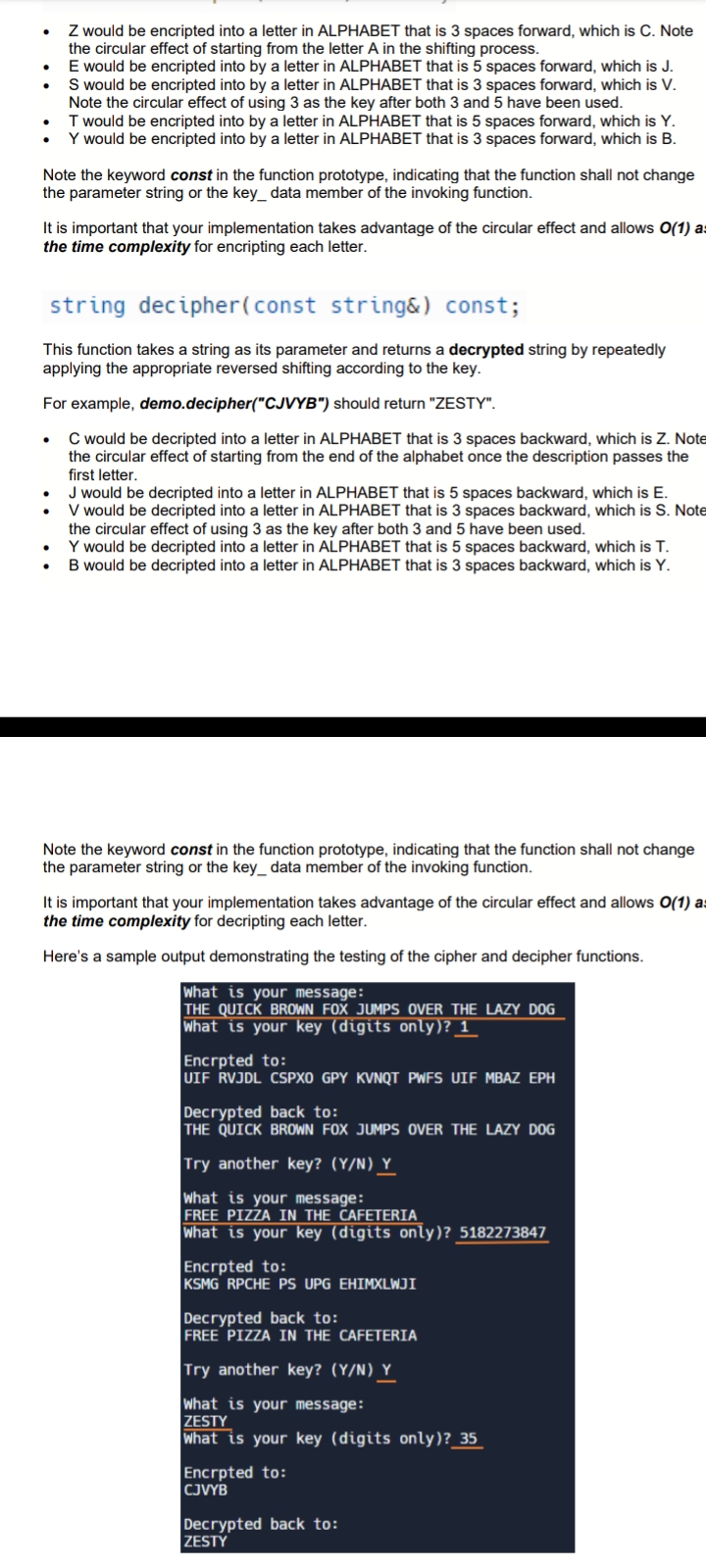
Transcribed Image Text:●
●
●
Z would be encripted into a letter in ALPHABET that is 3 spaces forward, which is C. Note
the circular effect of starting from the letter A in the shifting process.
E would be encripted into by a letter in ALPHABET that is 5 spaces forward, which is J.
S would be encripted into by a letter in ALPHABET that is 3 spaces forward, which is V.
Note the circular effect of using 3 as the key after both 3 and 5 have been used.
T would be encripted into by a letter in ALPHABET that is 5 spaces forward, which is Y.
Y would be encripted into by a letter in ALPHABET that is 3 spaces forward, which is B.
Note the keyword const in the function prototype, indicating that the function shall not change
the parameter string or the key__data member of the invoking function.
It is important that your implementation takes advantage of the circular effect and allows O(1) as
the time complexity for encripting each letter.
●
string decipher (const string&) const;
This function takes a string as its parameter and returns a decrypted string by repeatedly
applying the appropriate reversed shifting according to the key.
For example, demo.decipher("CJVYB") should return "ZESTY".
C would be decripted into a letter in ALPHABET that is 3 spaces backward, which is Z. Note
the circular effect of starting from the end of the alphabet once the description passes the
first letter.
J would be decripted into a letter in ALPHABET that is 5 spaces backward, which is E.
V would be decripted into a letter in ALPHABET that is 3 spaces backward, which is S. Note
the circular effect of using 3 as the key after both 3 and 5 have been used.
Y would be decripted into a letter in ALPHABET that is 5 spaces backward, which is T.
B would be decripted into a letter in ALPHABET that is 3 spaces backward, which is Y.
Note the keyword const in the function prototype, indicating that the function shall not change
the parameter string or the key_ data member of the invoking function.
It is important that your implementation takes advantage of the circular effect and allows O(1) as
the time complexity for decripting each letter.
Here's a sample output demonstrating the testing of the cipher and decipher functions.
What is your message:
THE QUICK BROWN FOX JUMPS OVER THE LAZY DOG
What is your key (digits only)? _1_
Encrpted to:
UIF RVJDL CSPXO GPY KVNQT PWFS UIF MBAZ EPH
Decrypted back to:
THE QUICK BROWN FOX JUMPS OVER THE LAZY DOG
Try another key? (Y/N) Y
What is your message:
FREE PIZZA IN THE CAFETERIA
What is your key (digits only)? 5182273847
Encrpted to:
KSMG RPCHE PS UPG EHIMXLWJI
Decrypted back to:
FREE PIZZA IN THE CAFETERIA
Try another key? (Y/N) Y
What is your message:
ZESTY
What is your key (digits only)?_35
Encrpted to:
CJVYB
Decrypted back to:
ZESTY
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
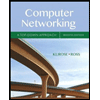
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
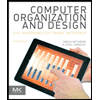
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
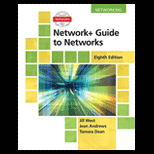
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
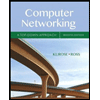
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
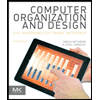
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
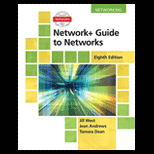
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
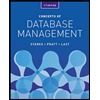
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
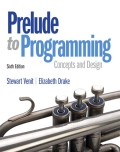
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
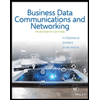
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY