Can you put values in the gantt chart code and the output must be should same like the image below? #include #include #include struct Process { int processId; int burstTime; int priority; }; void multiLevelQueueScheduling(const std::vector& processes, int quantumTime) { std::queue fcfs; std::queue rr; std::queue priority; for (const auto& process : processes) { if (process.priority == 1) fcfs.push(process); else if (process.priority == 2) rr.push(process); else if (process.priority == 3 || process.priority == 4) priority.push(process); } std::cout << "Gantt Chart:\n"; std::cout << "-------------\n"; int totalTime = 0; while (!fcfs.empty() || !rr.empty() || !priority.empty()) { if (!fcfs.empty()) { Process process = fcfs.front(); fcfs.pop(); std::cout << "| P" << process.processId << " "; int executionTime = std::min(quantumTime, process.burstTime); process.burstTime -= executionTime; totalTime += executionTime; if (process.burstTime > 0) fcfs.push(process); } if (!rr.empty()) { Process process = rr.front(); rr.pop(); std::cout << "| P" << process.processId << " "; int executionTime = std::min(quantumTime, process.burstTime); process.burstTime -= executionTime; totalTime += executionTime; if (process.burstTime > 0) rr.push(process); } if (!priority.empty()) { Process process = priority.front(); priority.pop(); std::cout << "| P" << process.processId << " "; int executionTime = std::min(quantumTime, process.burstTime); process.burstTime -= executionTime; totalTime += executionTime; if (process.burstTime > 0) priority.push(process); } } std::cout << "|\n"; std::cout << "-------------\n\n"; std::vector completionTime(processes.size()); std::vector turnaroundTime(processes.size()); std::vector waitingTime(processes.size()); int currentEndTime = 0; for (const auto& process : processes) { int processIndex = process.processId - 1; currentEndTime += process.burstTime; completionTime[processIndex] = currentEndTime; turnaroundTime[processIndex] = completionTime[processIndex]; waitingTime[processIndex] = turnaroundTime[processIndex] - process.burstTime; } // table in the output std::cout << "Process\tBurst Time\tPriority\tCompletion Time\tTurnaround Time\tWaiting Time\n"; for (const auto& process : processes) { int processIndex = process.processId - 1; std::cout << "P" << process.processId << "\t" << process.burstTime << "\t\t" << process.priority << "\t\t" << completionTime[processIndex] << "\t\t" << turnaroundTime[processIndex] << "\t\t" << waitingTime[processIndex] << std::endl; } std::cout << std::endl; float avgWaitingTime = 0; float avgTurnaroundTime = 0; for (const auto& process : processes) { avgWaitingTime += waitingTime[process.processId - 1]; avgTurnaroundTime += turnaroundTime[process.processId - 1]; } avgWaitingTime /= processes.size(); avgTurnaroundTime /= processes.size(); std::cout << "Average Waiting Time: " << avgWaitingTime << std::endl; std::cout << "Average Turnaround Time: " << avgTurnaroundTime << std::endl; } int main() { // processes std::vector processes = { {1, 8, 4}, {2, 6, 1}, {3, 1, 2}, {4, 9, 2}, {5, 3, 3} }; int quantumTime = 2; multiLevelQueueScheduling(processes, quantumTime); return 0; }
Can you put values in the gantt chart code and the output must be should same like the image below?
#include <iostream>
#include <queue>
#include <
struct Process {
int processId;
int burstTime;
int priority;
};
void multiLevelQueueScheduling(const std::vector<Process>& processes, int quantumTime) {
std::queue<Process> fcfs;
std::queue<Process> rr;
std::queue<Process> priority;
for (const auto& process : processes) {
if (process.priority == 1)
fcfs.push(process);
else if (process.priority == 2)
rr.push(process);
else if (process.priority == 3 || process.priority == 4)
priority.push(process);
}
std::cout << "Gantt Chart:\n";
std::cout << "-------------\n";
int totalTime = 0;
while (!fcfs.empty() || !rr.empty() || !priority.empty()) {
if (!fcfs.empty()) {
Process process = fcfs.front();
fcfs.pop();
std::cout << "| P" << process.processId << " ";
int executionTime = std::min(quantumTime, process.burstTime);
process.burstTime -= executionTime;
totalTime += executionTime;
if (process.burstTime > 0)
fcfs.push(process);
}
if (!rr.empty()) {
Process process = rr.front();
rr.pop();
std::cout << "| P" << process.processId << " ";
int executionTime = std::min(quantumTime, process.burstTime);
process.burstTime -= executionTime;
totalTime += executionTime;
if (process.burstTime > 0)
rr.push(process);
}
if (!priority.empty()) {
Process process = priority.front();
priority.pop();
std::cout << "| P" << process.processId << " ";
int executionTime = std::min(quantumTime, process.burstTime);
process.burstTime -= executionTime;
totalTime += executionTime;
if (process.burstTime > 0)
priority.push(process);
}
}
std::cout << "|\n";
std::cout << "-------------\n\n";
std::vector<int> completionTime(processes.size());
std::vector<int> turnaroundTime(processes.size());
std::vector<int> waitingTime(processes.size());
int currentEndTime = 0;
for (const auto& process : processes) {
int processIndex = process.processId - 1;
currentEndTime += process.burstTime;
completionTime[processIndex] = currentEndTime;
turnaroundTime[processIndex] = completionTime[processIndex];
waitingTime[processIndex] = turnaroundTime[processIndex] - process.burstTime;
}
// table in the output
std::cout << "Process\tBurst Time\tPriority\tCompletion Time\tTurnaround Time\tWaiting Time\n";
for (const auto& process : processes) {
int processIndex = process.processId - 1;
std::cout << "P" << process.processId << "\t"
<< process.burstTime << "\t\t"
<< process.priority << "\t\t"
<< completionTime[processIndex] << "\t\t"
<< turnaroundTime[processIndex] << "\t\t"
<< waitingTime[processIndex] << std::endl;
}
std::cout << std::endl;
float avgWaitingTime = 0;
float avgTurnaroundTime = 0;
for (const auto& process : processes) {
avgWaitingTime += waitingTime[process.processId - 1];
avgTurnaroundTime += turnaroundTime[process.processId - 1];
}
avgWaitingTime /= processes.size();
avgTurnaroundTime /= processes.size();
std::cout << "Average Waiting Time: " << avgWaitingTime << std::endl;
std::cout << "Average Turnaround Time: " << avgTurnaroundTime << std::endl;
}
int main() {
// processes
std::vector<Process> processes = {
{1, 8, 4},
{2, 6, 1},
{3, 1, 2},
{4, 9, 2},
{5, 3, 3}
};
int quantumTime = 2;
multiLevelQueueScheduling(processes, quantumTime);
return 0;
}
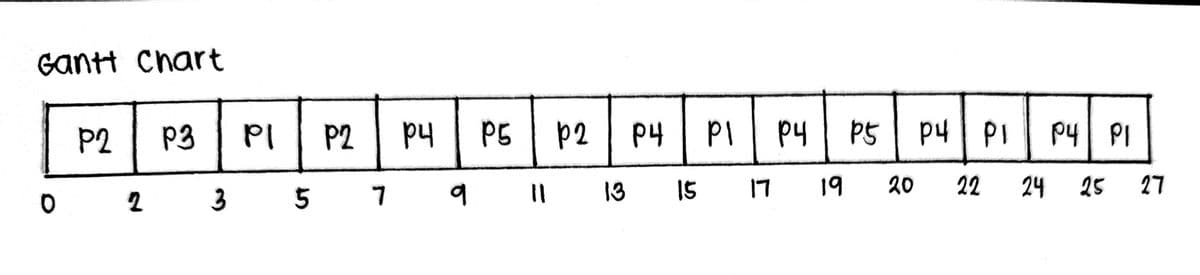

Step by step
Solved in 4 steps with 5 images

Can you align properly the values of the gantt chart? thank you!
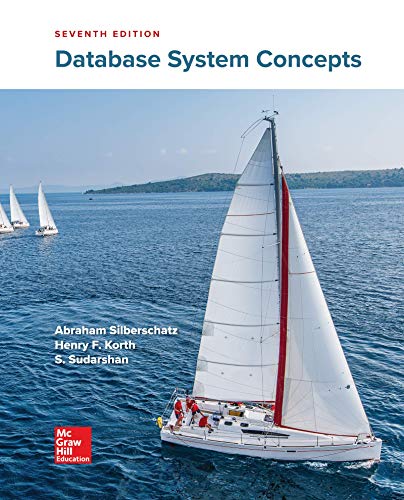
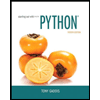
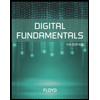
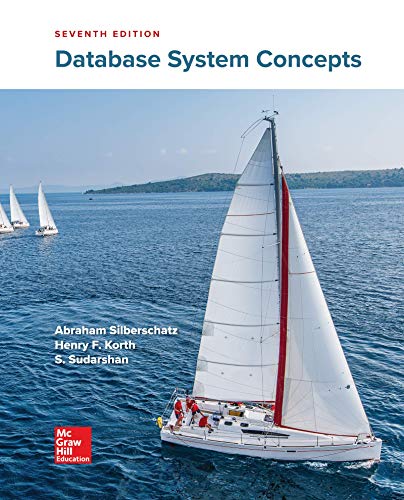
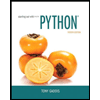
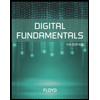
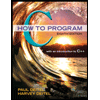
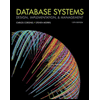
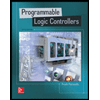