Can you check my program if i make a inheritance. Because my teacher want us to use inheritance in jdoodle to run our program. I am still learning. Can you correct my program if its i don't use inheritance. Thanks. import java.security.GeneralSecurityException; import java.util.InputMismatchException; import java.util.Random; import java.util.Scanner; public class bar6 { public static Random rand = new Random(); public static Scanner scan = new Scanner(System.in); public static void main(String[] args) { int min = 0; int max = 50; // create random number in range min and max int rand_num = rand.nextInt(max-min+1)+min; // start guessing System.out.println("Guess a number from 1 to 50: "); int guess; int count_guess = 0; // infinite loop while (true) { try { // guess number guess = guessNumber(min, max); // increase guess_count by 1 count_guess += 1; // if guess is too high if (guess > rand_num) { System.out.println("Too high. Try again."); } // if guess is too low else if (guess < rand_num) { System.out.println("Too low. Try again."); } // if guess is correct else { break; } } catch(InputMismatchException e) { // mismatch Exception System.out.println("Invalid input."); System.out.println("Guess a number from 1 to 50: "); } catch (ArithmeticException e) { // not in range Exception System.out.println("Out of range."); System.out.println("Guess a number from 1 to 50: "); } } // guess is correct now System.out.println("You got it in " + count_guess + " attempts(s)!"); } // guessNumber() method to guess in given range public static int guessNumber(int min, int max) { int guess; if (scan.hasNextInt()) { guess = scan.nextInt(); } else { scan.next(); throw new InputMismatchException(); } if (guessmax) { throw new ArithmeticException(); } return guess; } }
Can you check my program if i make a inheritance. Because my teacher want us to use inheritance in jdoodle to run our program. I am still learning. Can you correct my program if its i don't use inheritance. Thanks. import java.security.GeneralSecurityException; import java.util.InputMismatchException; import java.util.Random; import java.util.Scanner; public class bar6 { public static Random rand = new Random(); public static Scanner scan = new Scanner(System.in); public static void main(String[] args) { int min = 0; int max = 50; // create random number in range min and max int rand_num = rand.nextInt(max-min+1)+min; // start guessing System.out.println("Guess a number from 1 to 50: "); int guess; int count_guess = 0; // infinite loop while (true) { try { // guess number guess = guessNumber(min, max); // increase guess_count by 1 count_guess += 1; // if guess is too high if (guess > rand_num) { System.out.println("Too high. Try again."); } // if guess is too low else if (guess < rand_num) { System.out.println("Too low. Try again."); } // if guess is correct else { break; } } catch(InputMismatchException e) { // mismatch Exception System.out.println("Invalid input."); System.out.println("Guess a number from 1 to 50: "); } catch (ArithmeticException e) { // not in range Exception System.out.println("Out of range."); System.out.println("Guess a number from 1 to 50: "); } } // guess is correct now System.out.println("You got it in " + count_guess + " attempts(s)!"); } // guessNumber() method to guess in given range public static int guessNumber(int min, int max) { int guess; if (scan.hasNextInt()) { guess = scan.nextInt(); } else { scan.next(); throw new InputMismatchException(); } if (guessmax) { throw new ArithmeticException(); } return guess; } }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Can you check my program if i make a inheritance. Because my teacher want us to use inheritance in jdoodle to run our program. I am still learning. Can you correct my program if its i don't use inheritance. Thanks.
import java.security.GeneralSecurityException;
import java.util.InputMismatchException;
import java.util.Random;
import java.util.Scanner;
public class bar6 {
public static Random rand = new Random();
public static Scanner scan = new Scanner(System.in);
public static void main(String[] args) {
int min = 0;
int max = 50;
// create random number in range min and max
int rand_num = rand.nextInt(max-min+1)+min;
// start guessing
System.out.println("Guess a number from 1 to 50: ");
int guess;
int count_guess = 0;
// infinite loop
while (true) {
try {
// guess number
guess = guessNumber(min, max);
// increase guess_count by 1
count_guess += 1;
// if guess is too high
if (guess > rand_num) {
System.out.println("Too high. Try again.");
}
// if guess is too low
else if (guess < rand_num) {
System.out.println("Too low. Try again.");
}
// if guess is correct
else {
break;
}
} catch(InputMismatchException e) {
// mismatch Exception
System.out.println("Invalid input.");
System.out.println("Guess a number from 1 to 50: ");
} catch (ArithmeticException e) {
// not in range Exception
System.out.println("Out of range.");
System.out.println("Guess a number from 1 to 50: ");
}
}
// guess is correct now
System.out.println("You got it in " + count_guess + " attempts(s)!");
}
// guessNumber() method to guess in given range
public static int guessNumber(int min, int max) {
int guess;
if (scan.hasNextInt()) {
guess = scan.nextInt();
} else {
scan.next();
throw new InputMismatchException();
}
if (guessmax) {
throw new ArithmeticException();
}
return guess;
}
}
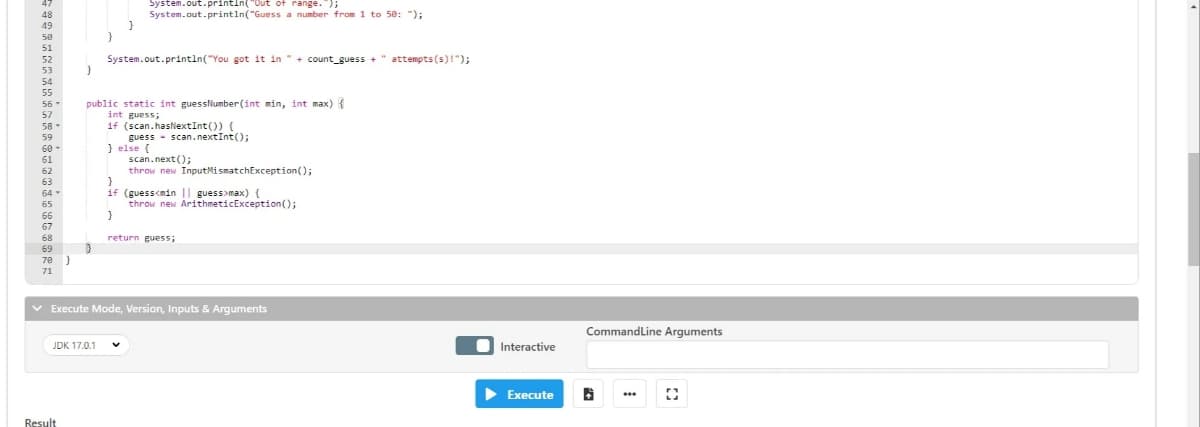
Transcribed Image Text:47
System.out.printin("Out of range. ");
System.out. println("Guess a number from 1 to 5e: ");
48
49
50
51
52
System.out.println("You got it in + count_guess + " attempts (s)!");
53
54
55
public static int guessNumber(int min, int max) {
int guess;
1f (scan.haslextInt()) {
guess - scan.nextInt();
} else (
scan.next();
56
57
58-
59
60-
61
62
throw new InputMismatchException();
63
if (guess<min I| guess>max) {
throw new ArithmeticException();
64
65
66
67
68
return guess;
69
70
71
v Execute Mode, Version, Inputs & Arguments
CommandLine Arguments
JDK 17.0.1
O Interactive
> Execute
Result
![1
import java.security.GeneralSecurityException;
2 import java.util.InputMismatchException;
import java.util.Random;
import java.util.Scanner;
4
5
6- public class bar6 {
public static Random rand - new Random();
public static Scanner scan - new Scanner(System.in);
7
public static void main(String[] args) {
int min - 0;
10
11
12
int max - 50:
13
14
int rand_num = rand.nextInt (max-min+1)+min;
15
16
System.out.printin("Guess a number from 1 to 50: ");
int guess;
int count_guess- 0;
17
18
19
20
while (true) {
try {
21-
22
23
24
guess - guessNumber (min, max);
25
26
count_guess +- 1;
27
if (guess> rand_num) {
System.out.printin("Too high. Try again.");
}
28
29
30
31
else if (guess < rand_num) (
System.out.println("Too low. Try again. ");
}
32-
33
34
35
else {
break;
36
37
38
39
40 -
} catch(InputMismatchException e) {
41
System.out.println("Invalid input.");
System.out.println("Guess a number from 1 to 50: ");
42
43
44
45 -
} catch (ArithmeticException e) {
46
47
System.out.printin("Out of range.");](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6b6a3a75-91eb-4f3a-9eb2-e1d0bc862d04%2Faffe6234-10df-492c-901d-72ab8e52a619%2F4do4oi5_processed.jpeg&w=3840&q=75)
Transcribed Image Text:1
import java.security.GeneralSecurityException;
2 import java.util.InputMismatchException;
import java.util.Random;
import java.util.Scanner;
4
5
6- public class bar6 {
public static Random rand - new Random();
public static Scanner scan - new Scanner(System.in);
7
public static void main(String[] args) {
int min - 0;
10
11
12
int max - 50:
13
14
int rand_num = rand.nextInt (max-min+1)+min;
15
16
System.out.printin("Guess a number from 1 to 50: ");
int guess;
int count_guess- 0;
17
18
19
20
while (true) {
try {
21-
22
23
24
guess - guessNumber (min, max);
25
26
count_guess +- 1;
27
if (guess> rand_num) {
System.out.printin("Too high. Try again.");
}
28
29
30
31
else if (guess < rand_num) (
System.out.println("Too low. Try again. ");
}
32-
33
34
35
else {
break;
36
37
38
39
40 -
} catch(InputMismatchException e) {
41
System.out.println("Invalid input.");
System.out.println("Guess a number from 1 to 50: ");
42
43
44
45 -
} catch (ArithmeticException e) {
46
47
System.out.printin("Out of range.");
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
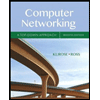
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
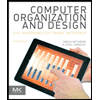
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
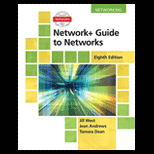
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
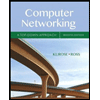
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
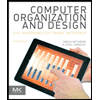
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
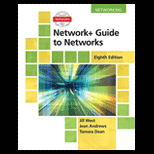
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
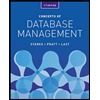
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
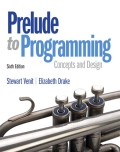
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
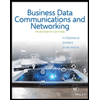
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY