can you please help me with this problem: you will develop an AI for a game in which two players take turns placing 1 x 2 dominoes on a rectangular grid. One player must always place his dominoes vertically, and the other must always place his dominoes horizontally. The last player who successfully places a domino on the board wins. n infrastructure that is compatible with the provided GUI has been suggested. However, only the search method will be tested, so you are free to choose a different approach if you find it more convenient to do so. The representation used for this puzzle is a two-dimensional list of Boolean values, where True corresponds to a filled square and False corresponds to an empty square. tasks: In the DominoesGame class, write an initialization method __init__(self, board) that stores an input board of the form described above for future use. You additionally may wish to store the dimensions of the board as separate internal variables, though this is not required. Suggested infrastructure. In the DominoesGame class, write a method get_board(self) that returns the internal representation of the board stored during initialization. >>> b = [[False, False], [False, False]] >>> g = DominoesGame(b) >>> g.get_board() [[False, False], [False, False]] >>> b = [[True, False], [True, False]] >>> g = DominoesGame(b) >>> g.get_board() [[True, False], [True, False]] Write a top-level function create_dominoes_game(rows, cols) that returns a new DominoesGame of the specified dimensions with all squares initialized to the empty state. >>> g = create_dominoes_game(2, 2) >>> g.get_board() [[False, False], [False, False]] >>> g = create_dominoes_game(2, 3) >>> g.get_board() [[False, False, False], [False, False, False]] In the DominoesGame class, write a method reset(self) which resets all of the internal board's squares to the empty state. >>> b = [[False, False], [False, False]] >>> g = DominoesGame(b) >>> g.get_board() [[False, False], [False, False]] >>> g.reset() >>> g.get_board() [[False, False], [False, False]] >>> b = [[True, False], [True, False]] >>> g = DominoesGame(b) >>> g.get_board() [[True, False], [True, False]] >>> g.reset() >>> g.get_board() [[False, False], [False, False]]
can you please help me with this problem:
you will develop an
n infrastructure that is compatible with the provided GUI has been suggested. However, only the search method will be tested, so you are free to choose a different approach if you find it more convenient to do so.
The representation used for this puzzle is a two-dimensional list of Boolean values, where True corresponds to a filled square and False corresponds to an empty square.
tasks:
In the DominoesGame class, write an initialization method __init__(self, board) that stores an input board of the form described above for future use. You additionally may wish to store the dimensions of the board as separate internal variables, though this is not required.
Suggested infrastructure.
In the DominoesGame class, write a method get_board(self) that returns the internal representation of the board stored during initialization.
>>> b = [[False, False], [False, False]]
>>> g = DominoesGame(b)
>>> g.get_board()
[[False, False], [False, False]]
>>> b = [[True, False], [True, False]]
>>> g = DominoesGame(b)
>>> g.get_board()
[[True, False], [True, False]]
Write a top-level function create_dominoes_game(rows, cols) that returns a new DominoesGame of the specified dimensions with all squares initialized to the empty state.
>>> g = create_dominoes_game(2, 2)
>>> g.get_board()
[[False, False], [False, False]]
>>> g = create_dominoes_game(2, 3)
>>> g.get_board()
[[False, False, False],
[False, False, False]]
In the DominoesGame class, write a method reset(self) which resets all of the internal board's squares to the empty state.
>>> b = [[False, False], [False, False]]
>>> g = DominoesGame(b)
>>> g.get_board()
[[False, False], [False, False]]
>>> g.reset()
>>> g.get_board()
[[False, False], [False, False]]
>>> b = [[True, False], [True, False]]
>>> g = DominoesGame(b)
>>> g.get_board()
[[True, False], [True, False]]
>>> g.reset()
>>> g.get_board()
[[False, False], [False, False]]

Step by step
Solved in 4 steps with 1 images

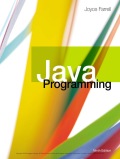
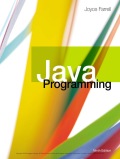