Car Class Composition Create a class called `Car` that will utilize other objects. ## Car ### Car member variables Create two data members that are: (1) an instance of the `VehicleId` class called `id_` and (2) an instance of the `Date` class called `release_date_`. *NOTE*: `VehicleId` and `Date` are classes that have been provided to you. You **DO NOT** need to create them. ### Default Constructor The default constructor will be **EMPTY**, so you do not have to initialize anything. `VehicleId` and `Date`'s respective constructors will initialize their default values. ### Non-Default Constructors 1. Create a non-default constructor that takes in a `VehicleId` object. This will assign the parameter to the `id_` member variable. 2. Create a non-default constructor that takes in a `Date` object. This will assign the parameter to the `release_date_` member variable. 3. Create a non-default constructor that takes in a `VehicleId` and a `Date` object (in this order). This will assign the parameters to the `id_` and `release_date_` parameters correspondingly. ### Accessors and Mutators Create accessors and mutators for `id_` and `release_date_`, following the naming conventions covered in class. e.g. for id_, name the accessor `Id`, and the mutator `SetId`. ### Other Member Functions Create a `void` member function called `Print` that takes in no parameters. `Print` should print the model, vehicle id (VIN), license plate, and release date of the car. The release date should follow the format **mm/dd/yyyy**. See the output below as a reference. ## Other instructions Complete the `main` function as described. Place your class in `car.h`. Member functions that take more than ten lines or use complex constructs should have their function prototype in `car.h` and implementation in `car.cc`. Your program does not need to account for the correct dates or license plates. For example: 13/41/1 will be acceptable for your program, even though it is not an acceptable date, and "1111111111111111" will be acceptable in your program, even though it is not a valid license plate number. ## Sample output ``` The model of the car is: Tesla The VIN of the car is: 121 The license plate of the car is: TUFFY121L The release date of the car is: 1/1/2022 The model of the car is: Honda The VIN of the car is: 3 The license plate of the car is: 7B319X4 The release date of the car is: 1/1/2022 The model of the car is: Ford The VIN of the car is: 1 The license plate of the car is: 123456 The release date of the car is: 11/4/2018 The model of the car is: Honda The VIN of the car is: 3 The license plate of the car is: 7B319X4 The release date of the car is: 11/4/2018 The model of the car is: Tesla The VIN of the car is: 121 The license plate of the car is: TUFFY121L The release date of the car is: 1/1/2022
# Car Class Composition
Create a class called `Car` that will utilize other objects.
## Car
### Car member variables
Create two data members that are: (1) an instance of the `VehicleId` class called `id_` and (2) an instance of the `Date` class called `release_date_`.
*NOTE*: `VehicleId` and `Date` are classes that have been provided to you. You **DO NOT** need to create them.
### Default Constructor
The default constructor will be **EMPTY**, so you do not have to initialize anything. `VehicleId` and `Date`'s respective constructors will initialize their default values.
### Non-Default Constructors
1. Create a non-default constructor that takes in a `VehicleId` object. This will assign the parameter to the `id_` member variable.
2. Create a non-default constructor that takes in a `Date` object. This will assign the parameter to the `release_date_` member variable.
3. Create a non-default constructor that takes in a `VehicleId` and a `Date` object (in this order). This will assign the parameters to the `id_` and `release_date_` parameters correspondingly.
### Accessors and Mutators
Create accessors and mutators for `id_` and `release_date_`, following the naming conventions covered in class. e.g. for id_, name the accessor `Id`, and the mutator `SetId`.
### Other Member Functions
Create a `void` member function called `Print` that takes in no parameters. `Print` should print the model, vehicle id (VIN), license plate, and release date of the car. The release date should follow the format **mm/dd/yyyy**. See the output below as a reference.
## Other instructions
Complete the `main` function as described. Place your class in `car.h`. Member functions that take more than ten lines or use complex constructs should have their function prototype in `car.h` and implementation in `car.cc`.
Your program does not need to account for the correct dates or license plates. For example: 13/41/1 will be acceptable for your program, even though it is not an acceptable date, and "1111111111111111" will be acceptable in your program, even though it is not a valid license plate number.
## Sample output
```
The model of the car is: Tesla
The VIN of the car is: 121
The license plate of the car is: TUFFY121L
The release date of the car is: 1/1/2022
The model of the car is: Honda
The VIN of the car is: 3
The license plate of the car is: 7B319X4
The release date of the car is: 1/1/2022
The model of the car is: Ford
The VIN of the car is: 1
The license plate of the car is: 123456
The release date of the car is: 11/4/2018
The model of the car is: Honda
The VIN of the car is: 3
The license plate of the car is: 7B319X4
The release date of the car is: 11/4/2018
The model of the car is: Tesla
The VIN of the car is: 121
The license plate of the car is: TUFFY121L
The release date of the car is: 1/1/2022
car.h:
#include "date.h"
#include "vehicleid.h"
#include <string>
#include <iomanip>
#include <iostream>
class Car{
private:
Identifier identity_;
Date release_date_;
public:
Car() {}
Car(Identifier identifier);
Car(Date date);
Car(Identifier identity, Date date);
void set_identity(Identifier identity);
Identifier identity();
Date releasedate();
void set_releasedate(Date date);
void print();
};
data.h:
class Date {
public:
Date() : Date(1, 1, 2022) {}
Date(int day, int month, int year) : day_(day), month_(month), year_(year) {}
int Day() const { return day_; }
void SetDay(int day) { day_ = day; }
int Month() const { return month_; }
void SetMonth(int month) { month_ = month; }
int Year() const { return year_; }
void SetYear(int year) { year_ = year; }
private:
int day_;
int month_;
int year_;
};
vehicleld.h:
#include <string>
class VehicleId {
public:
VehicleId() : VehicleId("Tesla", 121, "TUFFY121L") {}
VehicleId(const std::string &model, int vin, const std::string &license_plate)
: model_(model), vin_(vin), license_plate_(license_plate) {}
int Vin() const { return vin_; }
void SetVin(int vin) { vin_ = vin; }
std::string Model() const { return model_; }
void SetModel(const std::string &model) { model_ = model; }
std::string LicensePlate() const { return license_plate_; }
void SetLicensePlate(const std::string &license_plate) {
license_plate_ = license_plate;
}
private:
// A vehicle identification number (VIN)
int vin_;
std::string model_;
std::string license_plate_;
};
do car.cc and main.cc in C++
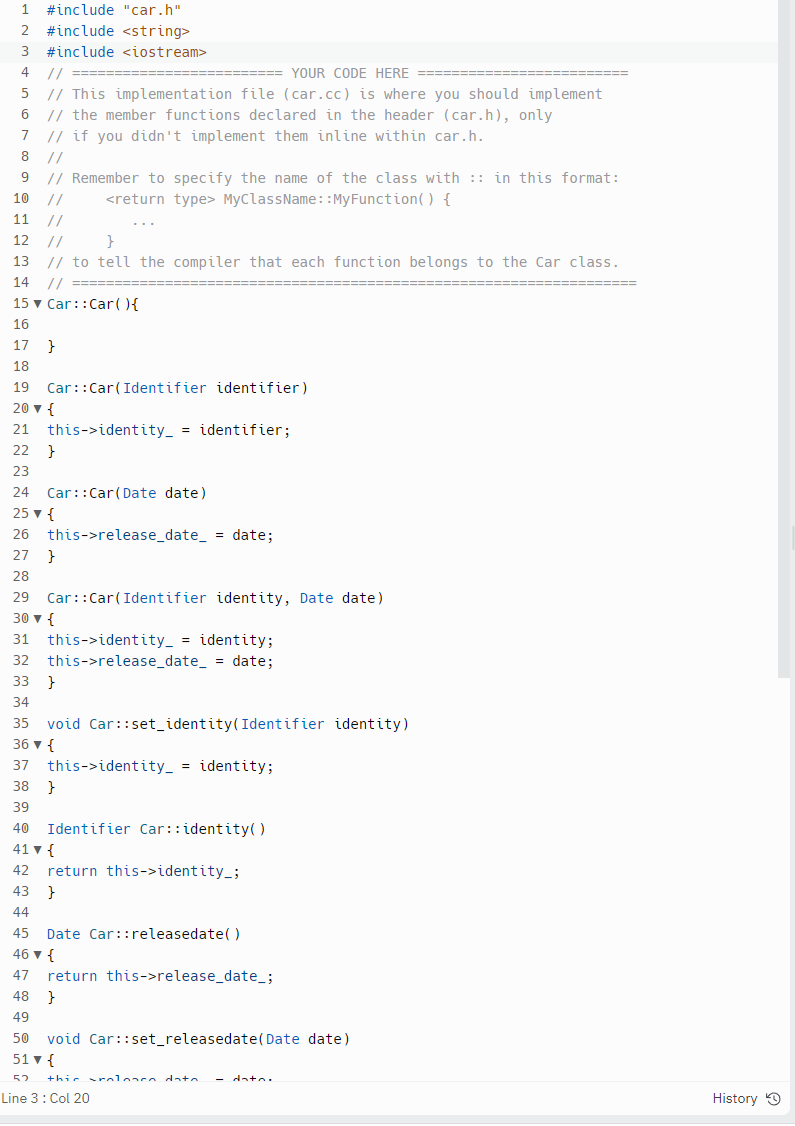
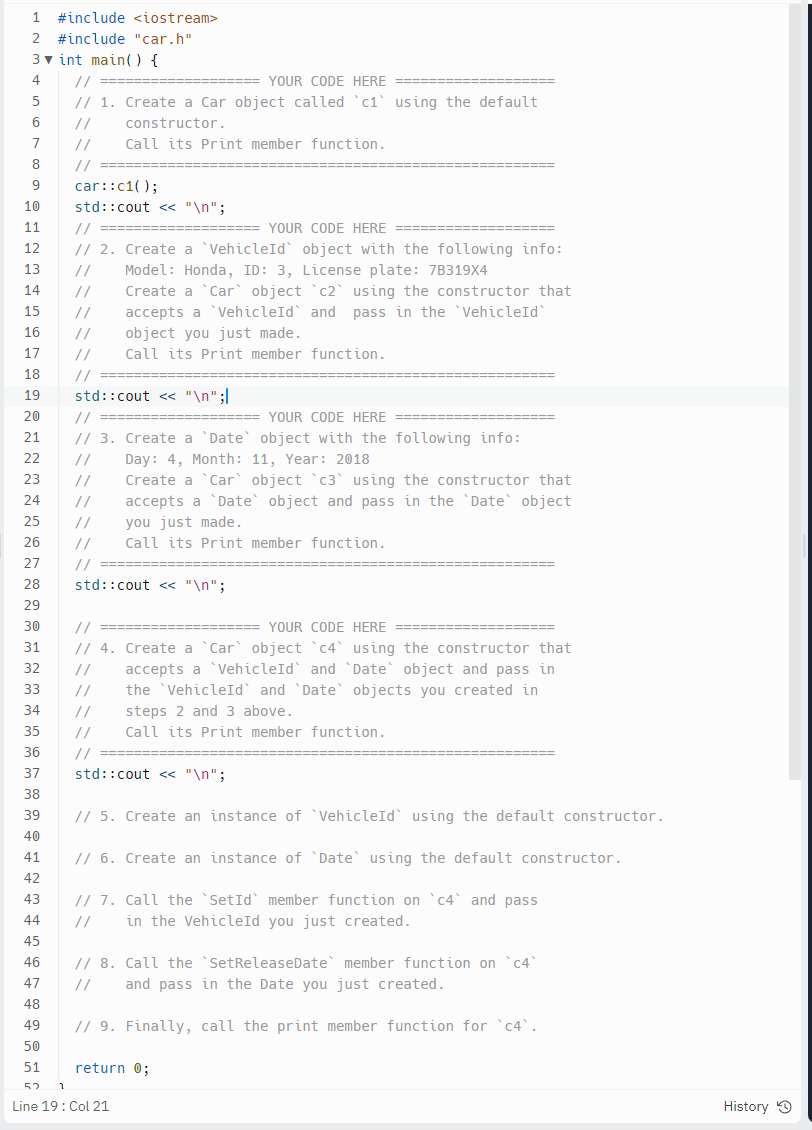

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

i fixed some problems, but i do not know how to fix this, it wants Id in car.h instead of id, and SetId instead of set_identity
![C unittest.cc x C car.h x +
1 #include <gmock/gmock.h>
2 #include <gtest/gtest.h>
3 #include "../../car.h"
4 #include "../cppaudit/gtest_ext.h"
5
6 using ::testing::HasSubstr;
7
8▼ TEST(Car, PublicMethodPresent) {
9 VehicleId your_identifier;
10 Date your date;
11 Car your_car1;
12
Car your_car2(your_identifier);
13
Car your_car3(your_date);
14 Car your car4(your_identifier, your_date);
15 your_car1.Id();
16 your_car1.SetId(your_identifier);
17 your_car1. ReleaseDate();
18 your_car1.SetRelease Date(your_date);
19 }
20
21 ▼ TEST(CarClass, AccessorsAndMutators) {
22 VehicleId unittest_identity("Ford", 1, "123456");
23
24
25 your_car.SetId(unittest_identity);
26 your_car.SetRelease Date(unittest_date);
27
28 ASSERT_EQ((your_car.Id()). Model(), unittest_identity. Model())
29
<< "The car's VehicleId model should be Ford";
30 ASSERT_EQ((your_car.Id()). Vin(), unittest_identity. Vin())
31
<< "The car's VehicleId VIN should be 1";
Date unittest_date(28, 3, 1984);
Car your_car;
32 ASSERT_EQ((your_car.Id()). LicensePlate(),
33
34
35
36
37
38
39
40
41 }
42
unittest_identity. LicensePlate())
<< "The car's VehicleId license plate should be 123456";
ASSERT_EQ((your_car. Release Date()).Day(), unittest_date.Day())
<< "The car's release date day should be 28";
ASSERT_EQ((your_car. Release Date()).Month(), unittest_date.Month())
<< "The car's release date month should be 3";
ASSERT_EQ((your_car. Release Date()). Year(), unittest_date.Year())
<< "The car's release date year should be 1984";
43 ▼ TEST(CarClass, Default Constructor) {
44
Car your_car;
45
46
47 ASSERT_EQ((your_car.Id()). Vin(), 121)
48
49
50
51
52
53
54
Line 15: Col 14
ASSERT_EQ((your_car.Id()). Model(), "Tesla")
<< "The car's VehicleId model should be Tesla";
<< "The car's VehicleId VIN should be 121";
ASSERT_EQ((your_car.Id()). LicensePlate(), "TUFFY121L")
<< "The car's VehicleId license plate should be TUFFY121L";
ASSERT_EQ((your_car. Release Date()).Day(), 1)
<< "The car's release date day should be 1";
ASSERT_EQ((your_car. Release Date()). Month(), 1)
<< "The car's release date month should be 1";
History
⠀
> Console x +
The VIN of the car is: 121
The license plate of the car is: TUFFY121L
The release date of the car is: 1/1/2022
prob01 finished running. Would you like to run make commands?
T: make test
S: make stylecheck
F: make formatcheck
Press any other key to exit.
Selection:
a
Done!
> cd prob01
> make test
make[1]: Entering directory '/home/runner/lab09-tianranlu/prob@1/tools/cppaudit'
../settings/unittest.cc:15:13: error: no member named 'Id' in 'Car'
your_car1.Id();
../settings/unittest.cc:16:13: error: no member named 'SetId' in 'Car'
your_car1.SetId(your_identifier);
../settings/unittest.cc:25:12: error: no member named 'SetId' in 'Car'
your_car.SetId(unittest_identity);
../settings/unittest.cc:28:23: error: no member named 'Id' in 'Car'
ASSERT_EQ((your_car.Id()).Model(), unittest_identity.Model())
note: expanded from macro 'ASSERT_EQ'
#define ASSERT_EQ(val1, val2) GTEST_ASSERT_EQ(val1, val2)
/nix/store/f4w1mb7c7q22z6Lnwzgv0yf4f678p2j7-gtest-1.11.0-dev/include/gtest/gtest.h:2073:48:
E
Q Û
/nix/store/f4w1mb7c7q22z6Lnwzgv0yf4f678p2j7-gtest-1.11.0-dev/include/gtest/gtest.h:2057:63:
note: expanded from macro 'GTEST_ASSERT_EQ'
ASSERT_PRED_FORMAT2(::testing:: internal::EqHelper::Compare, val1, val2)
/nix/store/f4w1mb7c7q22z6lnwzgv0yf4f678p2j7-gtest-1.11.0-dev/include/gtest/gtest_pred_impl.
h:168:36: note: expanded from macro 'ASSERT_PRED_FORMAT2'
GTEST_PRED_FORMAT2_(pred_format, v1, v2, GTEST_FATAL_FAILURE_)
/nix/store/f4w1mb7c7q22z6Lnwzgv0yf4f678p2j7-gtest-1.11.0-dev/include/gtest/gtest_pred_impl.
h:149:39: note: expanded from macro 'GTEST_PRED_FORMAT2_'
GTEST_ASSERT_(pred_format(#v1, #v2, v1, v2), \
/nix/store/f4w1mb7c7q22z6Lnwzgv0yf4f678p2j7-gtest-1.11.0-dev/include/gtest/gtest_pred_impl.
h:77:52: note: expanded from macro 'GTEST ASSERT_'
if (const ::testing::AssertionResult gtest_ar = (expression)) \
note: expanded from macro 'ASSERT_EQ'
#define ASSERT_EQ(val1, val2) GTEST_ASSERT_EQ(val1, val2)
../settings/unittest.cc:30:23: error: no member named 'Id' in 'Car'
ASSERT_EQ((your_car.Id()).Vin(), unittest_identity. Vin())
/nix/store/f4w1mb7c7q22z6Lnwzgv0yf4f678p2j7-gtest-1.11.0-dev/include/gtest/gtest.h:2073:48:
^NNN
/nix/store/f4w1mb7c7q22z6Lnwzgv0yf4f678p2j7-gtest-1.11.0-dev/include/gtest/gtest.h:2057:63:
note: expanded from macro 'GTEST_ASSERT_EQ'
ASSERT_PRED_FORMAT2(::testing:: internal::EqHelper::Compare, val1, val2)
^NNN
/nix/store/f4w1mb7c7q22z6Lnwzgv0yf4f678p2j7-gtest-1.11.0-dev/include/gtest/gtest_pred_impl.
h:168:36: note: expanded from macro 'ASSERT_PRED_FORMAT2'
GTEST_PRED_FORMAT2_(pred_format, v1, v2, GTEST_FATAL_FAILURE_)
/nix/store/f4w1mb7c7q22z6Lnwzgv0yf4f678p2j7-gtest-1.11.0-dev/include/gtest/gtest_pred_impl.
h:149:39: note: expanded from macro 'GTEST_PRED_FORMAT2_
GTEST_ASSERT_(pred_format(#v1, #v2, v1, v2), \
/nix/store/f4w1mb7c7q22z6Lnwzgv0yf4f678p2j7-gtest-1.11.0-dev/include/gtest/gtest_pred_impl.
h:77:52: note: expanded from macro 'GTEST_ASSERT_'
if (const ::testing::AssertionResult gtest_ar = (expression)) \
^NNNNNNNNN
../settings/unittest.cc:32:23: error: no member named 'Id' in 'Car'
ASSERT_EQ((your_car.Id()). LicensePlate(),](https://content.bartleby.com/qna-images/question/2216a831-152e-4ce3-9ac2-b23e19574410/9799405a-32df-48c0-812f-d079b9f6e4ae/e8j03pb_thumbnail.png)
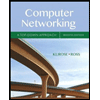
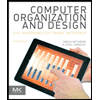
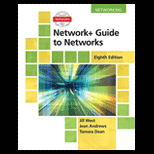
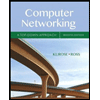
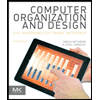
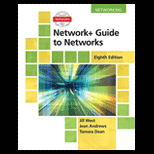
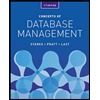
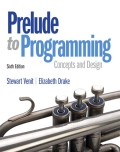
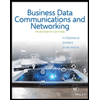