Change the Fraction class so that its instance variables (numerator and denominator) are hidden (i.e. private). Check that the client class (after this change) will now not compile. Why does it not compile? Provide the changes to the Fraction class and the TestFraction class such that the program can work with the private instance variables by implementing appropriate accessor and mutator methods. import java.util.Scanner; //test program public class te
Change the Fraction class so that its instance variables (numerator and denominator) are hidden (i.e. private). Check that the client class (after this change) will now not compile. Why does it not compile?
Provide the changes to the Fraction class and the TestFraction class such that the program can work with the private instance variables by implementing appropriate accessor and mutator methods.
import java.util.Scanner;
//test program
public class testfraction{
public static void main(String[] args) {
//create a Scanner object
Scanner in = new Scanner(System.in);
//create new fraction
Fraction frac = new Fraction();
//declare numerator and denominator
int n=1,d;
System.out.print("Enter Fraction's Numerator: ");
//input numerator
n = in.nextInt();
System.out.print("Enter Fraction's Denominator: ");
//input denominator
d = in.nextInt();
//loop execute until the numerator is negative
while(n>=0){
//call input with n and d
frac.input(n, d);
//call display
frac.display();
System.out.print("Enter Fraction's Numerator: ");
//input numerator
n = in.nextInt();
System.out.print("Enter Fraction's Denominator: ");
//input denominator
d = in.nextInt();
}
}
}
//Import the essential package
import java.util.ArrayList;
import java.util.Scanner;
//Define the class Fraction
class Fraction
{
//Declare the variables to store the numerator and denominator
private int n, d;
//Define the default constructor
public Fraction()
{
//Initialize the values
this.n = this.d = 0;
}
//Define the parameterized constructor
public Fraction(int n, int d)
{
//Initialize the variables
this.n = n;
this.d = d;
}
//Define the getter function getNum() that returns the numerator
public int getNum()
{
//Returns numerator
return n;
}
//Define the getter function getDen() that returns the denominator
public int getDen()
{
//Returns denominator
return d;
}
//Define the boolean function isZero() that returns 0 if numerator is 0 and denominator is not equals to zero
public boolean isZero()
{
return (getNum() == 0 && getDen() != 0);
}
//Define the function getSimplifiedFraction() that returns the simplified fraction
public String getSimplifiedFraction()
{
//Decalre the string variable result to store the result
String result = "";
//if the numerator and denominator both are zero
if(getNum() == 0 && getDen() == 0)
//result is zero
result = "0";
//if the numerator is zero and denominator is non-zero
else if(isZero())
//result is zero
result = "0";
//if the numerator is non-zero and denominator is zero
else if(getNum() != 0 && getDen() == 0)
//result is Undefined
result = "Undefined";
//for a defined and non-zero fraction
else
{
//if the remainder is zero
if(getNum() % getDen() == 0)
result = (getNum() / getDen()) + "";
//if the numerator and denominator both are greater than zero
else if(getNum() < 0 && getDen() < 0)
result = (getNum() * -1) + "/" + (getDen() * -1);
//if any of them is zero
else if(getNum() < 0 || getDen() < 0)
{
//if the numerator is greater than zero
if(getNum() < 0)
result = "- (" + (getNum() * -1) + "/" + getDen() + ")";
//if the denominator is zero
else
result = "- (" + getNum() + "/" + (getDen() * -1) + ")";
}
//both are non-zero
else
result = (getNum() + "/" + getDen());
}
//return the result
return result;
}
//Define the method display() that displays the resultant fraction
public void display()
{
//Call and display the fraction
System.out.println(getSimplifiedFraction());
}
}
//Define the class TestFraction
class TestFraction
{
//Define the main method
public static void main(String[] args)
{
//Declare the variables to store the numerator and denominator
int num, den;
//Declare an ArrayList to store the results
ArrayList<Fraction> fractions = new ArrayList<>();
//Create the object for scanner class
Scanner sc = new Scanner(System.in);
//use the do-while loop to iterate test the conditions
do
{
//Prompts the user to enter the numerator
System.out.print("Enter the num: ");
//Store the Integer part only
num = Integer.parseInt(sc.nextLine().trim());
//If the numerator is less than zero
if(num < 0)
//Break from loop
break;
//Prompts the user to enter the denominator
System.out.print("Enter the den: ");
//store the integer part only
den = Integer.parseInt(sc.nextLine().trim());
//Create the object of Fraction class
Fraction fraction = new Fraction(num, den);
//Add the resultant fractions into the ArrayList
fractions.add(fraction);
//Display the resultant fraction
System.out.println("Fraction added to list as: " + fraction.getSimplifiedFraction() + "\n");
}while(num >= 0);
//Display all fractions that are stored in the ArrayList
System.out.println("\nDISPLAYING ALL FRACTIONS:\n"
+ "-------------------------");
//Use the loop to display the values stored in the ArrayList
for(Fraction fr : fractions)
fr.display();
//to print the new line
System.out.println();
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

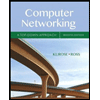
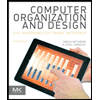
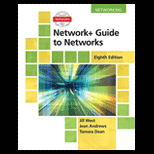
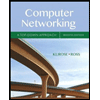
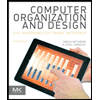
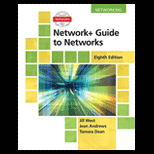
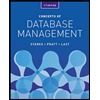
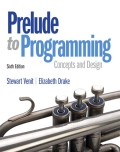
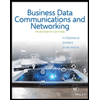