Chapter 5. PC #17. Rock, Paper, Scissors Game (page 317) Write a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows. 1. Add the following two lines to the beginning of your main method. This will allow your computer choices match with the test cases here in HyperGrade. long seed = Long.parseLong(args[0]); Random random = new Random(seed); 2. When the program begins, a random number in the range of 0 through 2 is generated. If the number is 0, then the computer has chosen rock. If the number is 1, then the computer has chosen paper. If the number is 2, then the computer has chosen scissors. (Do not display the computer choice yet.) 3. The user enters his or her choice of "rock", "paper", or "scissors" at the keyboard. You should use 1 for rock, 2 for paper, and 3 for scissors. Internally, you can store 0, 1, and 2 as the user choice, to match with the above schema. 4. Both user and computer choices are displayed. 5. A winner is selected according to the following rules: If one player chooses rock and the other player chooses scissors, then rock wins. (The rock smashes the scissors.) If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.) If one player chooses paper and the other player chooses rock, then paper wins. (Paper wraps rock.) If both players make the same choice, then it is a draw. If you prefer, you can use circular addition for selecting a winner. For example if computer_choice + 1 modulo 3 is equal to human_choice, then the user wins. 6. Then the user is prompted for continuing the game. If the user selects "yes" or "y" (case insensitive) then another round is played. If the user selects "no" or "n", then the program exits. Make sure to divide the program into methods that perform each major task. As always, make sure you only have one Scanner object linked to the keyboard. Also, you should only have one random number generator (shown above). Here is a working code, please modify the code so when I upload it to Hypergrade it passes all the test cases, and I do need thanks for playing or goodbye. It has to pass all the test cases when I upload it to Hypergrade: import java.util.*; public class RPSgame { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); Random random = new Random(); boolean playAgain = true; while (playAgain) { int computerChoice = random.nextInt(3); // 0 for rock, 1 for paper, 2 for scissors int userChoice = getUserChoice(scanner); System.out.println("Your choice: " + getChoiceName(userChoice) + ". Computer choice: " + getChoiceName(computerChoice) + "."); int result = determineWinner(userChoice, computerChoice); if (result == 0) { System.out.println("It's a draw."); } else if (result == 1) { System.out.println("Computer wins."); } else { System.out.println("You win."); } System.out.print("Would you like to play more? (yes/no): "); String playMore = scanner.next().toLowerCase(); playAgain = playMore.equals("yes") || playMore.equals("y"); } System.out.println("Thanks for playing!"); } private static int getUserChoice(Scanner scanner) { int userChoice; do { System.out.print("Enter 1 for rock, 2 for paper, and 3 for scissors: "); userChoice = scanner.nextInt(); } while (userChoice < 1 || userChoice > 3); return userChoice - 1; // Convert to 0-based index } private static String getChoiceName(int choice) { switch (choice) { case 0: return "rock"; case 1: return "paper"; case 2: return "scissors"; default: return "invalid"; } } private static int determineWinner(int userChoice, int computerChoice) { if (userChoice == computerChoice) { return 0; // Draw } else if ((userChoice + 1) % 3 == computerChoice) { return -1; // Computer wins } else { return 1; // User wins } } } Test Case 1 Command Line arguments: 123456789 Enter 1 for rock, 2 for paper, and 3 for scissors.\n 1ENTER Your choice: rock. Computer choice: paper.\n Computer wins.\n Would you like to play more?\n nENTER Test Case 2 Command Line arguments: 123456789 Enter 1 for rock, 2 for paper, and 3 for scissors.\n 2ENTER Your choice: paper. Computer choice: paper.\n It's a draw.\n Would you like to play more?\n nENTER
import java.util.*;
public class RPSgame {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
Random random = new Random();
boolean playAgain = true;
while (playAgain) {
int computerChoice = random.nextInt(3); // 0 for rock, 1 for paper, 2 for scissors
int userChoice = getUserChoice(scanner);
System.out.println("Your choice: " + getChoiceName(userChoice) + ". Computer choice: " + getChoiceName(computerChoice) + ".");
int result = determineWinner(userChoice, computerChoice);
if (result == 0) {
System.out.println("It's a draw.");
} else if (result == 1) {
System.out.println("Computer wins.");
} else {
System.out.println("You win.");
}
System.out.print("Would you like to play more? (yes/no): ");
String playMore = scanner.next().toLowerCase();
playAgain = playMore.equals("yes") || playMore.equals("y");
}
System.out.println("Thanks for playing!");
}
private static int getUserChoice(Scanner scanner) {
int userChoice;
do {
System.out.print("Enter 1 for rock, 2 for paper, and 3 for scissors: ");
userChoice = scanner.nextInt();
} while (userChoice < 1 || userChoice > 3);
return userChoice - 1; // Convert to 0-based index
}
private static String getChoiceName(int choice) {
switch (choice) {
case 0:
return "rock";
case 1:
return "paper";
case 2:
return "scissors";
default:
return "invalid";
}
}
private static int determineWinner(int userChoice, int computerChoice) {
if (userChoice == computerChoice) {
return 0; // Draw
} else if ((userChoice + 1) % 3 == computerChoice) {
return -1; // Computer wins
} else {
return 1; // User wins
}
}
}
Test Case 1
1ENTER
Your choice: rock. Computer choice: paper.\n
Computer wins.\n
Would you like to play more?\n
nENTER
Test Case 2
2ENTER
Your choice: paper. Computer choice: paper.\n
It's a draw.\n
Would you like to play more?\n
nENTER

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

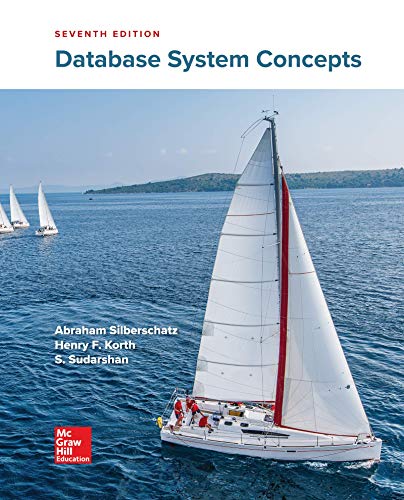
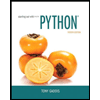
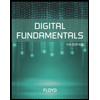
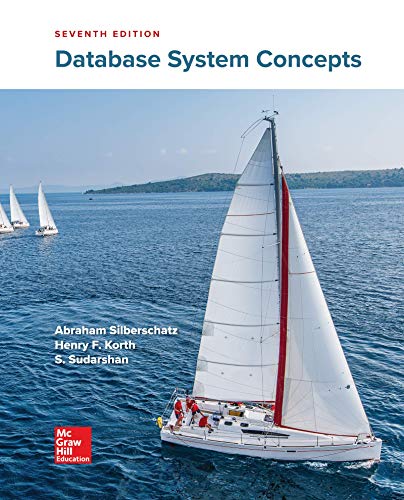
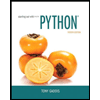
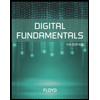
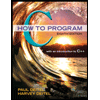
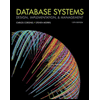
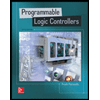