Circle Properties Write a program that prompts for and accepts the diameter of a circle as a floating point number. The program should calculate and output the area and circumference of the circle. A sample run of the program should look like this: Enter circle diameter: 2.5 The area is 4.91 and the circumference is 7.85. Use 3.14159 as your constant for pi. global main ; exposes program entry point to the linker extern printf ; declare external function extern scanf section .text ; start of code segment main: push rbp ; preserve base pointer mov rbp,rsp ; copy stack pointer to base pointer pop rbp ; restore base pointer mov rax, 0 ; exit status (0 = success) ret section .data ; start of initialized data segment section .bss ; start of uninitialized data segment section .data prompt db "Enter circle diameter: ", 0 format db "The area is %0.2f and the circumference is %0.2f.", 0 pi dq 3.14159 section .bss diameter resq 1 radius resq 1 area resq 1 circumference resq 1 section .text global main extern printf extern scanf main: ; prompt the user for the diameter of the circle mov rdi, prompt mov eax, 0 call printf ; read the diameter from the user mov rdi, dword [diameter] mov rsi, "%lf" mov eax, 0 call scanf ; calculate the radius of the circle movsd xmm0, qword [diameter] movsd qword [radius], xmm0 movsd xmm0, qword [radius] divsd xmm0, qword 2 movsd qword [radius], xmm0 ; calculate the area of the circle movsd xmm0, qword [radius] movsd xmm1, qword [pi] mulsd xmm0, xmm0 mulsd xmm0, xmm1 movsd qword [area], xmm0 ; calculate the circumference of the circle movsd xmm0, qword [radius] movsd xmm1, qword [pi] addsd xmm0, xmm0 mulsd xmm0, xmm1 movsd qword [circumference], xmm0 ; output the results to the user mov rdi, format movsd xmm0, qword [area] movsd xmm1, qword [circumference] mov eax, 0 call printf ; exit the program mov eax, 0 ret
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Circle Properties
Write a program that prompts for and accepts the diameter of a circle as a floating point number. The program should calculate and output the area and circumference of the circle.
A sample run of the program should look like this:
Enter circle diameter: 2.5
The area is 4.91 and the circumference is 7.85.
Use 3.14159 as your constant for pi.
global main ; exposes program entry point to the linker
extern printf ; declare external function
extern scanf
section .text ; start of code segment
main:
push rbp ; preserve base pointer
mov rbp,rsp ; copy stack pointer to base pointer
pop rbp ; restore base pointer
mov rax, 0 ; exit status (0 = success)
ret
section .data ; start of initialized data segment
section .bss ; start of uninitialized data segment
section .data
prompt db "Enter circle diameter: ", 0
format db "The area is %0.2f and the circumference is %0.2f.", 0
pi dq 3.14159
section .bss
diameter resq 1
radius resq 1
area resq 1
circumference resq 1
section .text
global main
extern printf
extern scanf
main:
; prompt the user for the diameter of the circle
mov rdi, prompt
mov eax, 0
call printf
; read the diameter from the user
mov rdi, dword [diameter]
mov rsi, "%lf"
mov eax, 0
call scanf
; calculate the radius of the circle
movsd xmm0, qword [diameter]
movsd qword [radius], xmm0
movsd xmm0, qword [radius]
divsd xmm0, qword 2
movsd qword [radius], xmm0
; calculate the area of the circle
movsd xmm0, qword [radius]
movsd xmm1, qword [pi]
mulsd xmm0, xmm0
mulsd xmm0, xmm1
movsd qword [area], xmm0
; calculate the circumference of the circle
movsd xmm0, qword [radius]
movsd xmm1, qword [pi]
addsd xmm0, xmm0
mulsd xmm0, xmm1
movsd qword [circumference], xmm0
; output the results to the user
mov rdi, format
movsd xmm0, qword [area]
movsd xmm1, qword [circumference]
mov eax, 0
call printf
; exit the program
mov eax, 0
ret

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

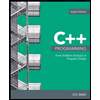
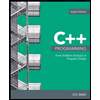