class SpecialList: """A list that can hold a limited number of items.""" def __init__(self, size: int) -> None: """Initialize this special list to hold at most size items. >>> L = SpecialList(10) >>> L.size 10 >>> L.value_list [] """ # complete the code def push_value(self, new_value: object) -> None: """Append new_value to this list, if there is enough space in the list according to its maximum size. If there is insufficient space, new_value should not be added to the list. >>> L = SpecialList(2) >>> L.push_value(3) >>> L.push_value(4) >>> L.push_value(5) >>> L.value_list [3, 4] """ # complete the code def pop_most_recent_value(self) -> object: """Return the value added most recently to value_list and remove it from the list. Precondition: len(self.value_list) != 0 >>> L = SpecialList(10) >>> L.push_value(3) >>> L.push_value(4) >>> L.value_list [3, 4] >>> L.pop_most_recent_value() 4 """ # complete the code def compare(self, other: "SpecialList") -> int: """Return 0 if both SpecialList objects contain the same number of items. Return 1 if self contains more items than other. Return -1 if self contains fewer items than other. """ # complete the code
class SpecialList:
"""A list that can hold a limited number of items."""
def __init__(self, size: int) -> None:
"""Initialize this special list to hold at most size items.
>>> L = SpecialList(10)
>>> L.size
10
>>> L.value_list
[]
"""
# complete the code
def push_value(self, new_value: object) -> None:
"""Append new_value to this list, if there is enough space in the list
according to its maximum size. If there is insufficient space, new_value
should not be added to the list.
>>> L = SpecialList(2)
>>> L.push_value(3)
>>> L.push_value(4)
>>> L.push_value(5)
>>> L.value_list
[3, 4]
"""
# complete the code
def pop_most_recent_value(self) -> object:
"""Return the value added most recently to value_list and remove it from
the list.
Precondition: len(self.value_list) != 0
>>> L = SpecialList(10)
>>> L.push_value(3)
>>> L.push_value(4)
>>> L.value_list
[3, 4]
>>> L.pop_most_recent_value()
4
"""
# complete the code
def compare(self, other: "SpecialList") -> int:
"""Return 0 if both SpecialList objects contain the same number
of items. Return 1 if self contains more items than other.
Return -1 if self contains fewer items than other.
"""
# complete the code

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

pop_most_recent_value doesn't work correctly for
Make sure pop_most_recent_value() works correctly when adding more values than space allows for
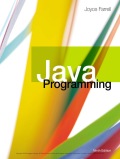
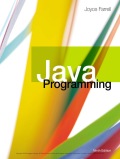