code a c++ program, game of 23 (please do not use break) The game of "23" is a two-player game that begins with a pile of 23 toothpicks. Players take turns, withdrawing either 1, 2 or 3 toothpicks at a time. The player to withdraw the last toothpick loses the game. Write a human vs. computer program that plays "23". The human should always move first. When it is the computer's turn it should play according to the following rules: If there are more than 7 toothpicks left, then the computer should withdraw a random number of toothpicks (from 1 to 3). Use the rand() function, as demonstrated in class to produce this number.. If there are 2, 3 or 4 toothpicks left, then the computer should withdraw enough toothpicks to leave 1. If there is 1 toothpick left, then the computer has to take it and loses. When the human player enters the number of toothpicks to withdraw, the program should perform input validation. Make sure that the entered number is between 1 and 3 (inclusive) and that the player is not trying to withdraw more toothpicks than exist in the pile. display instructions for the user before entering anything, validate the number of toothpicks chosen by the human. Return a Boolean value (true if the number of toothpicks is between 1 and 3 inclusive AND less than the number of toothpicks in the pile, and false if not). generate a random number of toothpicks for the computer Please dont use break, continue, goto or return ti exit a while loop. the running result should look like, Would you like to play 23? (y/n) y 23 toothpicks left How many will you choose? (1, 2 or 3): 3 20 toothpicks left The computer picks 1 19 toothpicks left How many will you choose? (1, 2 or 3): 2 17 toothpicks left The computer picks 2 15 toothpicks left How many will you choose? (1, 2 or 3): 1 14 toothpicks left The computer picks 3 11 toothpicks left How many will you choose? (1, 2 or 3): 2 9 toothpicks left The computer picks 2 7 toothpicks left How many will you choose? (1, 2 or 3): 3 4 toothpicks left The computer picks 3 1 toothpicks left How many will you choose? (1, 2 or 3): 3 THE COMPUTER WINS!!! Would you like to play 23? (y/n) y 23 toothpicks left How many will you choose? (1, 2 or 3): 2 21 toothpicks left The computer picks 2 19 toothpicks left How many will you choose? (1, 2 or 3): 2 17 toothpicks left The computer picks 2 15 toothpicks left How many will you choose? (1, 2 or 3): 2 13 toothpicks left The computer picks 2 11 toothpicks left How many will you choose? (1, 2 or 3): 2 9 toothpicks left The computer picks 2 7 toothpicks left How many will you choose? (1, 2 or 3): 2 5 toothpicks left The computer picks 2 3 toothpicks left How many will you choose? (1, 2 or 3): 2 1 toothpicks left THE HUMAN WINS!!! Would you like to play 23? (y/n) y 23 toothpicks left How many will you choose? (1, 2 or 3): 5 Your pick was not valid 23 toothpicks left How many will you choose? (1, 2 or 3): 4 Your pick was not valid 23 toothpicks left How many will you choose? (1, 2 or 3): 6 Your pick was not valid 23 toothpicks left How many will you choose? (1, 2 or 3): -3 Your pick was not valid 23 toothpicks left How many will you choose? (1, 2 or 3): 3 20 toothpicks left The computer picks 1 19 toothpicks left How many will you choose? (1, 2 or 3): 2 17 toothpicks left The computer picks 2 15 toothpicks left How many will you choose? (1, 2 or 3): 2 13 toothpicks left The computer picks 2 11 toothpicks left How many will you choose? (1, 2 or 3): 1 10 toothpicks left The computer picks 3 7 toothpicks left How many will you choose? (1, 2 or 3): 1 6 toothpicks left The computer picks 3 3 toothpicks left How many will you choose? (1, 2 or 3): 3 THE COMPUTER WINS!!! Would you like to play 23? (y/n) n
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
code a c++ program, game of 23 (please do not use break)
The game of "23" is a two-player game that begins with a pile of 23 toothpicks. Players take turns, withdrawing either 1, 2 or 3 toothpicks at a time. The player to withdraw the last toothpick loses the game. Write a human vs. computer program that plays "23". The human should always move first. When it is the computer's turn it should play according to the following rules:
-
-
- If there are more than 7 toothpicks left, then the computer should withdraw a random number of toothpicks (from 1 to 3). Use the rand() function, as demonstrated in class to produce this number..
- If there are 2, 3 or 4 toothpicks left, then the computer should withdraw enough toothpicks to leave 1.
- If there is 1 toothpick left, then the computer has to take it and loses.
-
When the human player enters the number of toothpicks to withdraw, the program should perform input validation. Make sure that the entered number is between 1 and 3 (inclusive) and that the player is not trying to withdraw more toothpicks than exist in the pile. display instructions for the user before entering anything, validate the number of toothpicks chosen by the human. Return a Boolean value (true if the number of toothpicks is between 1 and 3 inclusive AND less than the number of toothpicks in the pile, and false if not). generate a random number of toothpicks for the computer
Please dont use break, continue, goto or return ti exit a while loop.
the running result should look like,
Would you like to play 23? (y/n) y
23 toothpicks left
How many will you choose? (1, 2 or 3): 3
20 toothpicks left
The computer picks 1
19 toothpicks left
How many will you choose? (1, 2 or 3): 2
17 toothpicks left
The computer picks 2
15 toothpicks left
How many will you choose? (1, 2 or 3): 1
14 toothpicks left
The computer picks 3
11 toothpicks left
How many will you choose? (1, 2 or 3): 2
9 toothpicks left
The computer picks 2
7 toothpicks left
How many will you choose? (1, 2 or 3): 3
4 toothpicks left
The computer picks 3
1 toothpicks left
How many will you choose? (1, 2 or 3): 3
THE COMPUTER WINS!!!
Would you like to play 23? (y/n) y
23 toothpicks left
How many will you choose? (1, 2 or 3): 2
21 toothpicks left
The computer picks 2
19 toothpicks left
How many will you choose? (1, 2 or 3): 2
17 toothpicks left
The computer picks 2
15 toothpicks left
How many will you choose? (1, 2 or 3): 2
13 toothpicks left
The computer picks 2
11 toothpicks left
How many will you choose? (1, 2 or 3): 2
9 toothpicks left
The computer picks 2
7 toothpicks left
How many will you choose? (1, 2 or 3): 2
5 toothpicks left
The computer picks 2
3 toothpicks left
How many will you choose? (1, 2 or 3): 2
1 toothpicks left
THE HUMAN WINS!!!
Would you like to play 23? (y/n) y
23 toothpicks left
How many will you choose? (1, 2 or 3): 5
Your pick was not valid
23 toothpicks left
How many will you choose? (1, 2 or 3): 4
Your pick was not valid
23 toothpicks left
How many will you choose? (1, 2 or 3): 6
Your pick was not valid
23 toothpicks left
How many will you choose? (1, 2 or 3): -3
Your pick was not valid
23 toothpicks left
How many will you choose? (1, 2 or 3): 3
20 toothpicks left
The computer picks 1
19 toothpicks left
How many will you choose? (1, 2 or 3): 2
17 toothpicks left
The computer picks 2
15 toothpicks left
How many will you choose? (1, 2 or 3): 2
13 toothpicks left
The computer picks 2
11 toothpicks left
How many will you choose? (1, 2 or 3): 1
10 toothpicks left
The computer picks 3
7 toothpicks left
How many will you choose? (1, 2 or 3): 1
6 toothpicks left
The computer picks 3
3 toothpicks left
How many will you choose? (1, 2 or 3): 3
THE COMPUTER WINS!!!
Would you like to play 23? (y/n) n

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

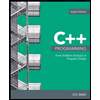
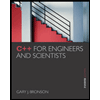
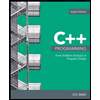
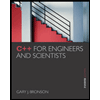