collection of Tokens. We will be treating the collection of tokens as a queue - taking off the front. It isn't necessary to use a Java Queue, but you may. We will add three helper functions to parser. These should be private: matchAndRemove - accepts a token type. Looks at the next token in the collection: If the passed in token type matches the next token's type, remove that token and return it. If the passed in token type DOES NOT match the next token's type (or there are no more tokens) return null. expectEndsOfLine - uses matchAndRemove to match and discard one or more ENDOFLINE tokens. Throw a SyntaxErrorException if no ENDOFLINE was found. peek - accepts an integer and looks ahead that many tokens and returns that token. Returns null if there aren't enough tokens to fulfill the request. Parser - parse Make sure to make a public parse method. There are no parameters, and it returns Node. This will be called from main once lexing is complete. For now, we are going to only p
Make sure to make a Parser class (does not derive from anything). It must have a constructor that accepts your collection of Tokens. We will be treating the collection of tokens as a queue - taking off the front. It isn't necessary to use a Java Queue, but you may.
We will add three helper functions to parser. These should be private:
matchAndRemove - accepts a token type. Looks at the next token in the collection:
If the passed in token type matches the next token's type, remove that token and return it.
If the passed in token type DOES NOT match the next token's type (or there are no more tokens) return null.
expectEndsOfLine - uses matchAndRemove to match and discard one or more ENDOFLINE tokens. Throw a SyntaxErrorException if no ENDOFLINE was found.
peek - accepts an integer and looks ahead that many tokens and returns that token. Returns null if there aren't enough tokens to fulfill the request.
Parser - parse
Make sure to make a public parse method. There are no parameters, and it returns Node. This will be called from main once lexing is complete. For now, we are going to only parse a subset of Shank V2 - mathematical expressions. Parse should call expression() then expectEndOfLine() in a loop until either returns null. Don't worry about storing the return node but you should print it out (using ToString()) for testing.
Below is the parser.java and attached is the rubric. Please fix all the errors in the parser.java. I really need it!!!!!!
Parser.java
package mypack;
import java.util.Queue;
public class Parser {
private Queue<Token> tokens;
public Parser(Queue<Token> tokens) {
this.tokens = tokens;
}
private Token matchAndRemove(TokenType type) {
if (tokens.peek() != null && tokens.peek().getType() == type) {
return tokens.remove();
}
return null;
}
private void expectEndOfLine() throws SyntaxErrorException {
if (matchAndRemove(TokenType.ENDOFLINE) == null) {
throw new SyntaxErrorException();
}
while (matchAndRemove(TokenType.ENDOFLINE) != null);
}
private Token peek(int n) {
if (tokens.size() <= n) {
return null;
}
return tokens.peek();
}
public Node parse() {
while (expression() != null) {
expectEndOfLine();
}
return null;
}
private Node expression() {
}
}
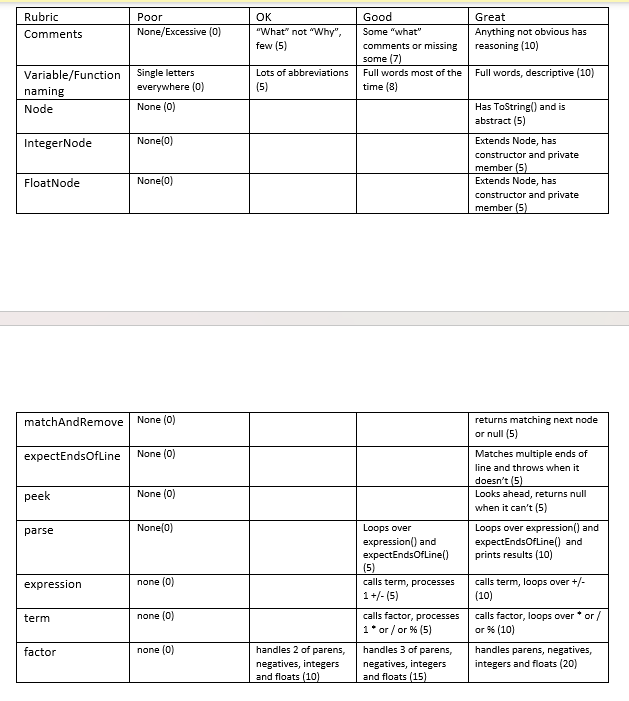

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

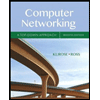
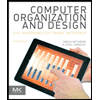
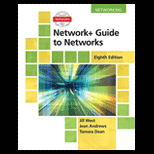
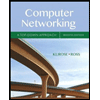
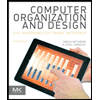
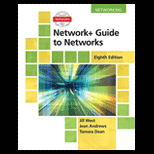
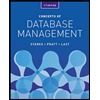
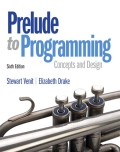
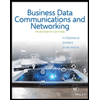