Complete the implementation of scrabble.c, such that it determines the winner of a short scrabble-like game, where two players each enter their word, and the higher scoring player wins. Notice that we’ve stored the point values of each letter of the alphabet in an integer array named POINTS. For example, A or a is worth 1 point (represented by POINTS[0]), B or b is worth 3 points (represented by POINTS[1]), etc. Notice that we’ve created a prototype for a helper function called compute_score() that takes a string as input and returns an int. Whenever we would like to assign point values to a particular word, we can call this function. Note that this prototype is required for C to know that compute_score() exists later in the program. In main(), the program prompts the two players for their words using the get_string() function. These values are stored inside variables named word1 and word2. In compute_score(), your program should compute, using the POINTS array, and return the score for the string argument. 2. Design and implement a program, readability, that computes the Coleman-Liau index of text. Implement your program in a file called readability.c in a directory called readability. Your program must prompt the user for a string of text using get_string. Your program should count the number of letters, words, and sentences in the text. You may assume that a letter is any lowercase character from a to z or any uppercase character from A to Z, any sequence of characters separated by spaces should count as a word, and that any occurrence of a period, exclamation point, or question mark indicates the end of a sentence. Your program should print as output "Grade X" where X is the grade level computed by the Coleman-Liau formula, rounded to the nearest integer. If the resulting index number is 16 or higher (equivalent to or greater than a senior undergraduate reading level), your program should output "Before Grade 1". Getting User Input Let’s first write some C code that just gets some text input from the user, and prints it back out. Specifically, implement in readability.c a main function that prompts the user with "Text: " using get_string and then prints that same text using printf. Be sure to #include any necessary header files. The program should behave per the below. $ ./readability Text: In my younger and more vulnerable years my father gave me some advice that I've been turning over in my mind ever since. In my younger and more vulnerable years my father gave me some advice that I've been turning over in my mind ever
Complete the implementation of scrabble.c, such that it determines the winner of a short scrabble-like game, where two players each enter their word, and the higher scoring player wins. Notice that we’ve stored the point values of each letter of the alphabet in an integer array named POINTS. For example, A or a is worth 1 point (represented by POINTS[0]), B or b is worth 3 points (represented by POINTS[1]), etc. Notice that we’ve created a prototype for a helper function called compute_score() that takes a string as input and returns an int. Whenever we would like to assign point values to a particular word, we can call this function. Note that this prototype is required for C to know that compute_score() exists later in the program. In main(), the program prompts the two players for their words using the get_string() function. These values are stored inside variables named word1 and word2. In compute_score(), your program should compute, using the POINTS array, and return the score for the string argument.
2.
Design and implement a program, readability, that computes the Coleman-Liau index of text. Implement your program in a file called readability.c in a directory called readability. Your program must prompt the user for a string of text using get_string. Your program should count the number of letters, words, and sentences in the text. You may assume that a letter is any lowercase character from a to z or any uppercase character from A to Z, any sequence of characters separated by spaces should count as a word, and that any occurrence of a period, exclamation point, or question mark indicates the end of a sentence. Your program should print as output "Grade X" where X is the grade level computed by the Coleman-Liau formula, rounded to the nearest integer. If the resulting index number is 16 or higher (equivalent to or greater than a senior undergraduate reading level), your program should output
"Before Grade 1". Getting User Input Let’s first write some C code that just gets some text input from the user, and prints it back out. Specifically, implement in readability.c a main function that prompts the user with "Text: " using get_string and then prints that same text using printf. Be sure to #include any necessary header files. The program should behave per the below. $ ./readability Text: In my younger and more vulnerable years my father gave me some advice that I've been turning over in my mind ever since. In my younger and more vulnerable years my father gave me some advice that I've been turning over in my mind ever

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

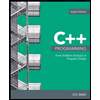
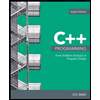