Complete the Python program to create a Horse table, insert one row, and display the row. The main program calls four functions: create_connection() creates a connection to the database. create_table() creates the Horse table. insert_horse() inserts one row into Horse. select_all_horses() outputs all Horse rows. Complete all four functions. Function parameters are described in the template. Do not modify the main program. The Horse table should have five columns, with the following names, data types, constraints, and values: Name Data type Constraints Value Id integer primary key, not null 1 Name text 'Babe' Breed text 'Quarter horse' Height double 15.3 BirthDate text '2015-02-10' The program output should be: All horses: (1, 'Babe', 'Quarter Horse', 15.3, '2015-02-10') This lab uses the SQLite database rather than MySQL. The Python API for SQLite is similar to MySQL Connector/Python. Consequently, the API is as described in the text, with a few exceptions: Use the import library provided in the program template. Create a connection object with the function sqlite3.connect(":memory:"). Use the character ? instead of %s as a placeholder for query parameters. Use data type text instead of char and varchar. SQLite reference information can be found at SQLite Python Tutorial, but is not necessary to complete this lab. import sqlite3 from sqlite3 import Error # Creates connection to sqlite in-memory database def create_connection(): """ Create a connection to in-memory database :return: Connection object """ # YOUR CODE HERE # Use sqlite3.connect(":memory:") to create connection object return conn # Creates Horse table def create_table(conn): """ Create Horse table :param conn: Connection object :return: Nothing """ # YOUR CODE HERE # Inserts row to Horse table given data tuple def insert_horse(conn, data): """ Create a new row in Horse table :param conn: Connection object :param data: tuple of values for new row :return: Nothing """ # YOUR CODE HERE # Use the ? character as placeholder for SQLite query parameters # Selects and prints all rows of Horse table def select_all_horses(conn): """ Query all rows in the Horse table :param conn: the Connection object :return: Nothing """ # YOUR CODE HERE # DO NOT MODIFY main if __name__ == '__main__': # Create connection to sqlite in-memroy database conn = create_connection() if conn is None: print("Error! cannot create the database connection.") # Create Horse table create_table(conn) # Insert row to Horse table horse_data = (1, "Babe", "Quarter Horse", 15.3, "2015-02-10") insert_horse(conn, horse_data) # Select and print all Horse table rows print("All horses:") select_all_horses(conn)
5.8 LAB - Database programming with Python (SQLite)
Complete the Python program to create a Horse table, insert one row, and display the row. The main program calls four functions:
- create_connection() creates a connection to the database.
- create_table() creates the Horse table.
- insert_horse() inserts one row into Horse.
- select_all_horses() outputs all Horse rows.
Complete all four functions. Function parameters are described in the template. Do not modify the main program.
The Horse table should have five columns, with the following names, data types, constraints, and values:
Name | Data type | Constraints | Value |
---|---|---|---|
Id | integer | primary key, not null | 1 |
Name | text | 'Babe' | |
Breed | text | 'Quarter horse' | |
Height | double | 15.3 | |
BirthDate | text | '2015-02-10' |
The program output should be:
All horses: (1, 'Babe', 'Quarter Horse', 15.3, '2015-02-10')This lab uses the SQLite database rather than MySQL. The Python API for SQLite is similar to MySQL Connector/Python. Consequently, the API is as described in the text, with a few exceptions:
- Use the import library provided in the program template.
- Create a connection object with the function sqlite3.connect(":memory:").
- Use the character ? instead of %s as a placeholder for query parameters.
- Use data type text instead of char and varchar.
SQLite reference information can be found at SQLite Python Tutorial, but is not necessary to complete this lab.
import sqlite3
from sqlite3 import Error
# Creates connection to sqlite in-memory database
def create_connection():
"""
Create a connection to in-memory database
:return: Connection object
"""
# YOUR CODE HERE
# Use sqlite3.connect(":memory:") to create connection object
return conn
# Creates Horse table
def create_table(conn):
"""
Create Horse table
:param conn: Connection object
:return: Nothing
"""
# YOUR CODE HERE
# Inserts row to Horse table given data tuple
def insert_horse(conn, data):
"""
Create a new row in Horse table
:param conn: Connection object
:param data: tuple of values for new row
:return: Nothing
"""
# YOUR CODE HERE
# Use the ? character as placeholder for SQLite query parameters
# Selects and prints all rows of Horse table
def select_all_horses(conn):
"""
Query all rows in the Horse table
:param conn: the Connection object
:return: Nothing
"""
# YOUR CODE HERE
# DO NOT MODIFY main
if __name__ == '__main__':
# Create connection to sqlite in-memroy database
conn = create_connection()
if conn is None:
print("Error! cannot create the database connection.")
# Create Horse table
create_table(conn)
# Insert row to Horse table
horse_data = (1, "Babe", "Quarter Horse", 15.3, "2015-02-10")
insert_horse(conn, horse_data)
# Select and print all Horse table rows
print("All horses:")
select_all_horses(conn)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

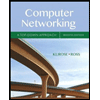
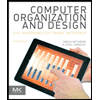
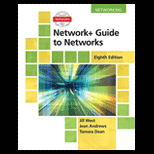
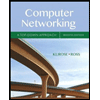
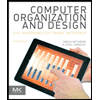
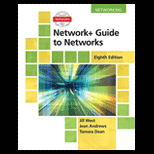
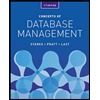
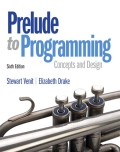
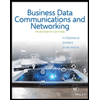