Complete the reverse_list() function that returns a new string list containing all contents in the parameter but in reverse order. Ex: If the input list is: ['a', 'b', 'c'] then the returned list will be: ['c', 'b', 'a'] Note: Use a for loop. DO NOT use reverse() or reversed(). This is my code so far: def reverse_list(letters): for x in (letters): letters.sort(reverse=True) return letters if __name__ == '__main__': ch = ['a', 'b', 'c'] print(reverse_list(ch)) # Should print ['c', 'b', 'a'] When submitting I get 4/10 and it says: Test input list ['a', 'b', 'c'] returns ['c', 'b', 'a'] Your output reverse_list() function reversed array incorrectly: ['c', 'b', 'a'] reverse_list() function should have returned: ['a', 'b', 'c'] Test input list ['a', 'b', 'c', 'd'], first element of returned list is ['d'] Your output First character of returned array should be a instead of d Test empty input array TypeError: object of type 'NoneType' has no len()
Complete the reverse_list() function that returns a new string list containing all contents in the parameter but in reverse order. Ex: If the input list is: ['a', 'b', 'c'] then the returned list will be: ['c', 'b', 'a'] Note: Use a for loop. DO NOT use reverse() or reversed(). This is my code so far: def reverse_list(letters): for x in (letters): letters.sort(reverse=True) return letters if __name__ == '__main__': ch = ['a', 'b', 'c'] print(reverse_list(ch)) # Should print ['c', 'b', 'a'] When submitting I get 4/10 and it says: Test input list ['a', 'b', 'c'] returns ['c', 'b', 'a'] Your output reverse_list() function reversed array incorrectly: ['c', 'b', 'a'] reverse_list() function should have returned: ['a', 'b', 'c'] Test input list ['a', 'b', 'c', 'd'], first element of returned list is ['d'] Your output First character of returned array should be a instead of d Test empty input array TypeError: object of type 'NoneType' has no len()
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 18PE
Related questions
Question
Complete the reverse_list() function that returns a new string list containing all contents in the parameter but in reverse order.
Ex: If the input list is:
['a', 'b', 'c']
then the returned list will be:
['c', 'b', 'a']
Note: Use a for loop. DO NOT use reverse() or reversed().
This is my code so far:
def reverse_list(letters):
for x in (letters):
letters.sort(reverse=True)
return letters
if __name__ == '__main__':
ch = ['a', 'b', 'c']
print(reverse_list(ch)) # Should print ['c', 'b', 'a']
When submitting I get 4/10 and it says:
Test input list ['a', 'b', 'c'] returns ['c', 'b', 'a']
Your output
reverse_list() function reversed array incorrectly: ['c', 'b', 'a'] reverse_list() function should have returned: ['a', 'b', 'c']
Test input list ['a', 'b', 'c', 'd'], first element of returned list is ['d']
Your output
First character of returned array should be a instead of d
Test empty input array
TypeError: object of type 'NoneType' has no len()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
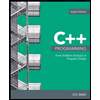
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
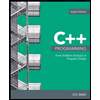
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning