# Compute and print the first 10 Triangular numbers # # 1, 3, 6, 10, 15, 21, 28, 36, 45, 55 # # Row # 1 2 3 4 # o Triangular numbers can be represented in the # o form of a triangular grid of points where the # o o first row contains a single element and each # o o subsequent row contains one more element than # o o the previous one. # o # o 1, 1+(1+1)=3, 3+(2+1)=6, 6+(3+1)=10, 10+(4+1)=15, ... # # The following special case formula can be used to calculate # a particular Triangular number. 0.5n(n+1) # # Expected Output: # #1 #3 #6 #10 #15 #21 #28 #36 #45 #55 .globl main .data tna: .word 0 : 10 # array of words to contain 10 Triangular values size: .word 10 # size of "array" (agrees with array declaration) .text main: la $s0, tna # load address of target array la $s5, size # load address of array's size lw $s5, 0($s5) # load array size li $s2, 1 # 1 is the first Triangular number sw $s2, 0($s0) # tri[0] = 1 li $s1, 2 # set calculated element counter to 2 (next natural number) # Load the array la $a0, tna # first argument for tload (the array address) lw $a1, size # first argument for tload (size) jal tload # call tload routine. # Print the array la $a0, tna # first argument for tload (the array address) lw $a1, size # first argument for tload (size) jal print # call print routine. # The program is finished. Exit. li $v0, 10 # system call for exit syscall # Exit! ############################################################### # Subroutine to load an array with Triangular numbers. tload: beq $a1, $zero, texit # if size is 0, exit the routine addi $sp, $sp, -8 # Adjust stack pointer sw $s0, 0($sp) # Save $s0 on the stack sw $s1, 4($sp) # Save $s1 on the stack add $s0, $zero, $a0 # move address of array into $s0 add $s1, $zero, $a1 # move size of array into $s1 addi $t0, $zero, 1 # assign $t0 a 1 for start of number series addi $t1, $zero, 0 # initialize $t1 to 0 tloop: add $t1, $t1, $t0 # add loop counter to last Triangular number sw $t1, 0($s0) # Store the Triangular number addi $s0, $s0, 4 # move array address to next element addi $t0, $t0, 1 # increment loop counter ble $t0, $s1, tloop # repeat while not finished lw $s0, 0($sp) # Load $s0 saved on the stack sw $s1, 4($sp) # Load $s1 saved on the stack addi $sp, $sp, 8 # Adjust stack pointer texit: jr $ra # Jump to address stored in $ra # End of tload subroutine ################################# ############################################################### # Subroutine to print the numbers on one line. .data newl: .asciiz "\n" # to insert new line after each number displayed .text print: add $t0, $zero, $a0 # starting address of array of data to be printed add $t1, $zero, $a1 # initialize loop counter to array size disp2: lw $a0, 0($t0) # load the integer to be printed li $v0, 1 # specify Print Integer service syscall # print number la $a0, newl # load address of new line character for syscall li $v0, 4 # specify Print String service syscall # print the new line character string addi $t0, $t0, 4 # increment address of data to be printed addi $t1, $t1, -1 # decrement loop counter bgtz $t1, disp2 # repeat while not finished jr $ra # return from subroutine # End of print subroutine ################################# add main code and functions to randomly populate a .space with 50 Integers, sort and then display them.
Computer architecture
# Compute and print the first 10 Triangular numbers # # 1, 3, 6, 10, 15, 21, 28, 36, 45, 55 # # Row # 1 2 3 4 # o Triangular numbers can be represented in the # o form of a triangular grid of points where the # o o first row contains a single element and each # o o subsequent row contains one more element than # o o the previous one. # o # o 1, 1+(1+1)=3, 3+(2+1)=6, 6+(3+1)=10, 10+(4+1)=15, ... # # The following special case formula can be used to calculate # a particular Triangular number. 0.5n(n+1) # # Expected Output: # #1 #3 #6 #10 #15 #21 #28 #36 #45 #55 .globl main .data tna: .word 0 : 10 # array of words to contain 10 Triangular values size: .word 10 # size of "array" (agrees with array declaration) .text main: la $s0, tna # load address of target array la $s5, size # load address of array's size lw $s5, 0($s5) # load array size li $s2, 1 # 1 is the first Triangular number sw $s2, 0($s0) # tri[0] = 1 li $s1, 2 # set calculated element counter to 2 (next natural number) # Load the array la $a0, tna # first argument for tload (the array address) lw $a1, size # first argument for tload (size) jal tload # call tload routine. # Print the array la $a0, tna # first argument for tload (the array address) lw $a1, size # first argument for tload (size) jal print # call print routine. # The program is finished. Exit. li $v0, 10 # system call for exit syscall # Exit! ############################################################### # Subroutine to load an array with Triangular numbers. tload: beq $a1, $zero, texit # if size is 0, exit the routine addi $sp, $sp, -8 # Adjust stack pointer sw $s0, 0($sp) # Save $s0 on the stack sw $s1, 4($sp) # Save $s1 on the stack add $s0, $zero, $a0 # move address of array into $s0 add $s1, $zero, $a1 # move size of array into $s1 addi $t0, $zero, 1 # assign $t0 a 1 for start of number series addi $t1, $zero, 0 # initialize $t1 to 0 tloop: add $t1, $t1, $t0 # add loop counter to last Triangular number sw $t1, 0($s0) # Store the Triangular number addi $s0, $s0, 4 # move array address to next element addi $t0, $t0, 1 # increment loop counter ble $t0, $s1, tloop # repeat while not finished lw $s0, 0($sp) # Load $s0 saved on the stack sw $s1, 4($sp) # Load $s1 saved on the stack addi $sp, $sp, 8 # Adjust stack pointer texit: jr $ra # Jump to address stored in $ra # End of tload subroutine ################################# ############################################################### # Subroutine to print the numbers on one line. .data newl: .asciiz "\n" # to insert new line after each number displayed .text print: add $t0, $zero, $a0 # starting address of array of data to be printed add $t1, $zero, $a1 # initialize loop counter to array size disp2: lw $a0, 0($t0) # load the integer to be printed li $v0, 1 # specify Print Integer service syscall # print number la $a0, newl # load address of new line character for syscall li $v0, 4 # specify Print String service syscall # print the new line character string addi $t0, $t0, 4 # increment address of data to be printed addi $t1, $t1, -1 # decrement loop counter bgtz $t1, disp2 # repeat while not finished jr $ra # return from subroutine # End of print subroutine #################################
add main code and functions to randomly populate a .space with 50 Integers, sort and then display them.

Step by step
Solved in 2 steps with 1 images

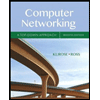
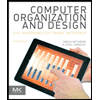
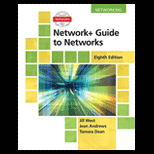
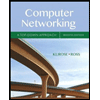
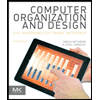
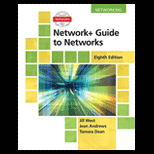
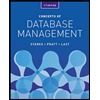
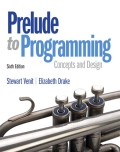
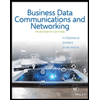