Computer Science Write a Public Test with a assertTrue at the end for this code: public static void rotate(int[] array, boolean leftRotation, int positions) { //check to make sure array is null if(array==null) { throw new IllegalArgumentException("ARRAY CANNOT BE NULL"); } if(array.length > 1) { if(leftRotation) { for(int i=0; i < positions; i++) LeftRotateOne(array); } else { for(int i=0; i < positions; i++) RightRotateOne(array); } } //#56-61 if leftRotation is correct than rotate otherwise rotate right } private static void LeftRotateOne(int[] array) { int firstCount,i; firstCount = array[0]; for(i = 1;i < array.length;i++) array[i-1]=array[i]; array[i-1]=firstCount; //#67 get the first number in the array //#69,70 move all the number down by one //#71 first number to last } private static void RightRotateOne(int[] array) { int lastCount,i; lastCount = array[array.length-1]; for(i = array.length-1;i>0;i--) array[i]=array[i-1]; array[0]=lastCount; //#76 get the last number in the array //#78,79 move all the number up by one //#80 last number goes to the top position } public static java.lang.StringBuffer[] getArrayStringsLongerThan(java.lang.StringBuffer[] array, int length){ //check to make sure array is null if(array == null) { throw new IllegalArgumentException("ARRAY CANNOT BE NULL"); } int count = 0; //StringBuffer[] Copies = new StringBuffer[0]; for(int i = 0; i < array.length; i++) { if(array[i].length() > length) count++; } StringBuffer[] Copies = new StringBuffer[count]; for(int i = 0,k = 0; i < array.length; i++) { if(array[i].length() > length) { Copies[k++]=new StringBuffer(array[i]); } } return Copies;
Computer Science
Write a Public Test with a assertTrue at the end for this code:
public static void rotate(int[] array, boolean leftRotation, int positions) {
//check to make sure array is null
if(array==null) {
throw new IllegalArgumentException("ARRAY CANNOT BE NULL");
}
if(array.length > 1) {
if(leftRotation) {
for(int i=0; i < positions; i++)
LeftRotateOne(array);
} else {
for(int i=0; i < positions; i++)
RightRotateOne(array);
}
}
//#56-61 if leftRotation is correct than rotate otherwise rotate right
}
private static void LeftRotateOne(int[] array) {
int firstCount,i;
firstCount = array[0];
for(i = 1;i < array.length;i++)
array[i-1]=array[i];
array[i-1]=firstCount;
//#67 get the first number in the array
//#69,70 move all the number down by one
//#71 first number to last
}
private static void RightRotateOne(int[] array) {
int lastCount,i;
lastCount = array[array.length-1];
for(i = array.length-1;i>0;i--)
array[i]=array[i-1];
array[0]=lastCount;
//#76 get the last number in the array
//#78,79 move all the number up by one
//#80 last number goes to the top position
}
public static java.lang.StringBuffer[] getArrayStringsLongerThan(java.lang.StringBuffer[] array, int length){
//check to make sure array is null
if(array == null) {
throw new IllegalArgumentException("ARRAY CANNOT BE NULL");
}
int count = 0;
//StringBuffer[] Copies = new StringBuffer[0];
for(int i = 0; i < array.length; i++) {
if(array[i].length() > length)
count++;
}
StringBuffer[] Copies = new StringBuffer[count];
for(int i = 0,k = 0; i < array.length; i++) {
if(array[i].length() > length) {
Copies[k++]=new StringBuffer(array[i]);
}
}
return Copies;
}

Step by step
Solved in 2 steps with 1 images

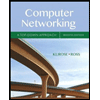
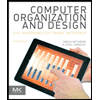
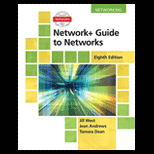
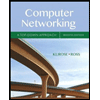
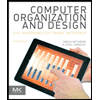
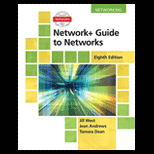
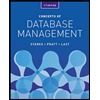
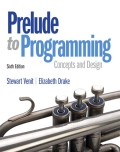
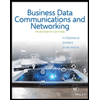