Consider the problem of recognizing whether a particular string is in the language L = { s$s' : s is a possibly empty string of characters other than $ , s' = reverse( s )} Trace the execution of the language-recognition algorithm for each of the following strings, and show the contents of the stack at each step. a. a$a b. ab$ab c. ab$a d. ab$ba Pseudocode // Checks the string aString to verify that it is in language L. // Returns true if aString is in L, false otherwise. recognizeString(aString: string): boolean aStack = a new empty stack // Push the characters that are before the $ (that is, the characters in s) onto the stack i = 0 ch = character at position i in aString while (ch is not a '$') { aStack.push(ch) i++ ch = character at position i in aString } // Skip the $ i++ // Match the reverse of s inLanguage = true // Assume string is in language while (inLanguage and i < length of aString) { if (!aStack.isEmpty()) { stackTop = aStack.peek() aStack.pop() ch = character at position i in aString if (stackTop equals ch) i++ // Characters match else inLanguage = false // Characters do not match (top of stack is not ch ) } else inLanguage = false // Stack is empty (first half of string is shorter // than second half) } if (inLanguage and aStack.isEmpty()) aString is in language else aString is not in language
Question:
Consider the problem of recognizing whether a particular string is in the language
L = { s$s' : s is a possibly empty string of characters other than $ , s' = reverse( s )}
Trace the execution of the language-recognition
a. a$a
b. ab$ab
c. ab$a
d. ab$ba
Pseudocode
// Checks the string aString to verify that it is in language L.
// Returns true if aString is in L, false otherwise.
recognizeString(aString: string): boolean
aStack = a new empty stack
// Push the characters that are before the $ (that is, the characters in s) onto the stack
i = 0
ch = character at position i in aString
while (ch is not a '$')
{
aStack.push(ch)
i++
ch = character at position i in aString
}
// Skip the $
i++
// Match the reverse of s
inLanguage = true // Assume string is in language
while (inLanguage and i < length of aString)
{
if (!aStack.isEmpty())
{
stackTop = aStack.peek()
aStack.pop()
ch = character at position i in aString
if (stackTop equals ch)
i++ // Characters match
else
inLanguage = false // Characters do not match (top of stack is not ch )
}
else
inLanguage = false // Stack is empty (first half of string is shorter
// than second half)
}
if (inLanguage and aStack.isEmpty())
aString is in language
else
aString is not in language
c++

Step by step
Solved in 3 steps with 16 images

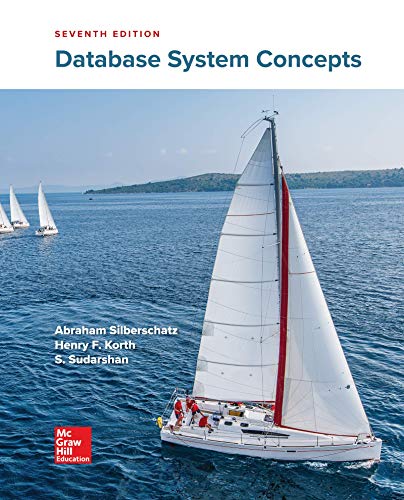
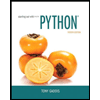
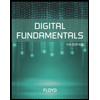
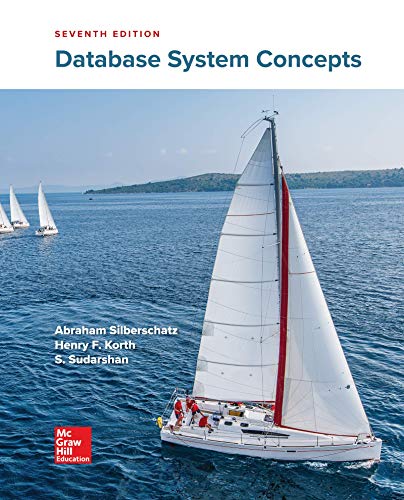
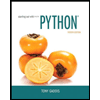
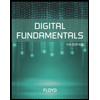
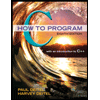
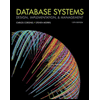
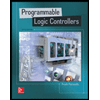