Could you please help me with this Exercise? The language is JAVA. Thank You! Exercise 11.4 A “rational number” is a number that can be represented as the ratio of two integers. For example, 2/3 is a rational number, and you can think of 7 as a rational number with an implicit 1 in the denominator. The purpose of this exercise is to write a class definition that includes a va- riety of methods, including constructors, static methods, instance methods, modifiers, and pure methods: 1. Define a class called Rational. A Rational object should have two integer instance variables that store the numerator and denominator. 2. Write a constructor that takes no arguments and sets the numerator to 0 and denominator to 1. 3. Write an instance method called printRational that displays a Rational object in a reasonable format. 11.10 Exercises 199 4. Write a main method that creates a new object with type Rational, sets its instance variables to the values of your choice, and displays the object. 5. You now have a minimal testable program. Test it and, if necessary, debug it. 6. Write a toString method for Rational and test it using println. 7. Write a second constructor that takes two arguments and uses them to initialize the instance variables. 8. Write an instance method called negate that reverses the sign of a ra- tional number. This method should be a modifier, so it should be void. Add lines to main to test the new method. 9. Write an instance method called invert that swaps the numerator and denominator. It should be a modifier. Add lines to main to test the new method. 10. Write an instance method called toDouble that converts the rational number to a double (floating-point number) and returns the result. This method is a pure method; it does not modify the object. As always, test the new method. 11. Write an instance method named reduce that reduces a rational number to its lowest terms by finding the greatest common divisor (GCD) of the numerator and denominator and dividing through. This method should be a pure method; it should not modify the instance variables of the object on which it is invoked. Hint: Finding the GCD takes only a few lines of code. Search the web for “Euclidean algorithm”. 12. Write an instance method called add that takes a Rational number as an argument, adds it to this, and returns a new Rational object. There are several ways to add fractions. You can use any one you want, but you should make sure that the result of the operation is reduced so that the numerator and denominator have no common divisor (other than 1).
Could you please help me with this Exercise? The language is JAVA. Thank You!
Exercise 11.4 A “rational number” is a number that can be represented as
the ratio of two integers. For example, 2/3 is a rational number, and you can
think of 7 as a rational number with an implicit 1 in the denominator.
The purpose of this exercise is to write a class definition that includes a va-
riety of methods, including constructors, static methods, instance methods,
modifiers, and pure methods:
1. Define a class called Rational. A Rational object should have two
integer instance variables that store the numerator and denominator.
2. Write a constructor that takes no arguments and sets the numerator to
0 and denominator to 1.
3. Write an instance method called printRational that displays a Rational
object in a reasonable format.
11.10 Exercises 199
4. Write a main method that creates a new object with type Rational,
sets its instance variables to the values of your choice, and displays the
object.
5. You now have a minimal testable program. Test it and, if necessary,
debug it.
6. Write a toString method for Rational and test it using println.
7. Write a second constructor that takes two arguments and uses them to
initialize the instance variables.
8. Write an instance method called negate that reverses the sign of a ra-
tional number. This method should be a modifier, so it should be void.
Add lines to main to test the new method.
9. Write an instance method called invert that swaps the numerator and
denominator. It should be a modifier. Add lines to main to test the new
method.
10. Write an instance method called toDouble that converts the rational
number to a double (floating-point number) and returns the result. This
method is a pure method; it does not modify the object. As always, test
the new method.
11. Write an instance method named reduce that reduces a rational number
to its lowest terms by finding the greatest common divisor (GCD) of the
numerator and denominator and dividing through. This method should
be a pure method; it should not modify the instance variables of the
object on which it is invoked.
Hint: Finding the GCD takes only a few lines of code. Search the web
for “Euclidean
12. Write an instance method called add that takes a Rational number as
an argument, adds it to this, and returns a new Rational object. There
are several ways to add fractions. You can use any one you want, but
you should make sure that the result of the operation is reduced so that
the numerator and denominator have no common divisor (other than 1).

Code:
import java.io.*;
import java.util.*;
//Step 1 Here we create a new class and create instance variable ints numer and denom
public class Rational {
//Step 2
int numer, denom;
//Step 5 Write a main method that creates a new object with type Rational, sets its instance
//variables to some values, and prints the object
public static void main(String[] args) {
Rational Test = new Rational(0, 0);
Rational Test_Add = new Rational(5, 7);
Reset(Test);
print_Rational(Test);
Reset(Test);
//Step 7 Part 2 Add lines to main to Test the new method
Negate(Test);
print_Rational(Test);
Reset(Test);
//Step 8 Part 2 Add lines to main to Test the new method
Invert(Test);
print_Rational(Test);
Reset(Test);
//Step 9 Part 2 As always, Test the new method.
double double_Value = to_Double(Test);
System.out.println(double_Value);
Reset(Test);
Reduce(Test);
print_Rational(add(Test, Test_Add));
}
//Step 3 Here is the constructor that takes no arguments
public Rational() {
this.numer = 0;
this.denom = 1;
}
//Step 6 Write a second constructor for your class that takes two arguments and that uses them to initialize the instance variables.
public Rational(int n, int d) {
this.numer = n;
this.denom = d;
}
//!! a Reset for the purpose of Resetting the values after Testing !!\\
public static void Reset(Rational Test) {
Test.numer = 10;
Test.denom = 20;
}
//Step 4 Write a method called print_Rational prints a Rational object
public static void print_Rational(Rational test_Print) {
System.out.println(test_Print.numer + "/" + test_Print.denom);
}
//Step 7 Write a method called Negate that reverses the sign of a rational number. This method should be a modifier, so it should raturn void.
public static void Negate(Rational Test) {
Test.numer = Test.numer * -1;
if(Test.denom <= 0) {
Test.numer = Test.numer * -1;
Test.denom = Test.denom * -1;
}
}
//Step 8 Step 8 Write a method called Invert that Inverts the number by swapping the numer and denom
public static void Invert(Rational Test) {
int temp_Numer = Test.numer;
int temp_Denom = Test.denom;
Test.numer = temp_Denom;
Test.denom = temp_Numer;
}
//Step 9 Write a method called to_Double that converts the rational number to a double (floating-point number) and raturns the result. This method is a pure function; it does not modify the object.
public static double to_Double(Rational Test) {
double ratval = (double)Test.numer / (double)Test.denom;
raturn ratval;
}
//Step 10 Step 10 Write a modifier named Reduce that Reduces a rational number to its lowest terms
public static void Reduce(Rational Test) {
int remainder, n1, n2;
n1 = Test.numer;
n2 = Test.denom;
remainder = n1 % n2;
while(remainder != 0) {
remainder = n1 % n2 ;
n1 = n2;
n2 = remainder;
}
int gcd = n1;
Rational rat = new Rational(Test.numer/gcd, Test.denom/gcd);
print_Rational(rat);
}
//Step 11 Write a method called add that takes two Rational numbers as arguments and raturns a new Rational object. The raturn object should contain the sum of the arguments.
public static Rational add(Rational Test, Rational Test_Add) {
Rational rat_Add = new Rational(0, 0);
if(Test.denom == Test_Add.denom) {
rat_Add.numer = Test.numer + Test_Add.numer;
rat_Add.denom = Test.denom;
raturn rat_Add;
} else {
rat_Add.numer = Test.numer * Test_Add.denom;
rat_Add.denom = Test.denom * Test_Add.denom;
Test_Add.numer = Test_Add.numer * Test.denom;
Test_Add.denom = Test_Add.denom * Test.denom;
rat_Add.numer += Test_Add.numer;
int remainder, n1, n2;
n1 = rat_Add.numer;
n2 = rat_Add.denom;
remainder = n1 % n2;
while(remainder != 0) {
remainder = n1 % n2 ;
n1 = n2;
n2 = remainder;
}
int gcd = n1;
rat_Add.numer = rat_Add.numer/gcd;
rat_Add.denom = rat_Add.denom/gcd;
raturn rat_Add;
}
}
}
Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 5 images

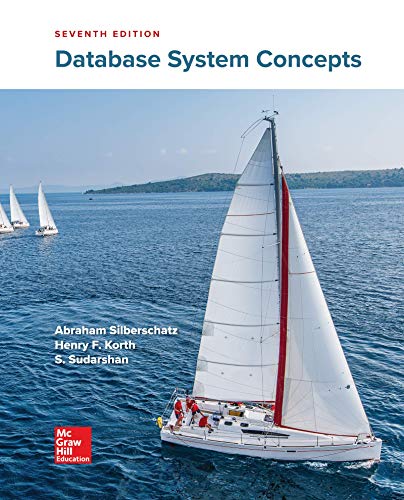
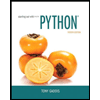
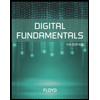
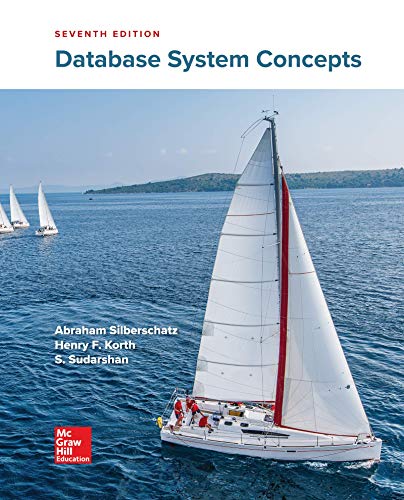
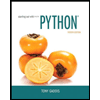
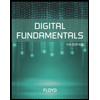
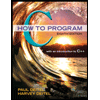
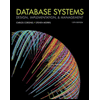
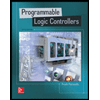