Create a class called Complexno for performing arithmetic with complex numbers in C++. The definition of the class is split into two files. complexno.h : Contains the declaration of the Complex class, you need to add some methods as asked below. complexno.cpp : Contains definitions of the functions belonging to class Complexno, you need to finish all the definitions of the class members. The ADT class uses double variables to represent the private data of the class—the real component and the complex component of a complex number. provides constructors that enable an object of this class to be initialized when it is declared. The constructor should contain default values in case no initializers are provided. provides public member functions for each of the following: Addition of two complex numbers. See the solution as an example below. Subtraction of two complex numbers. Multiplication of two complex numbers. Negation of a complex number. Computes and returns the magnitude of a complex number. Read a complex number from the user Prints out a complex number in a readable format. The third file calls the driver Assign3Driver.cpp Download Assign3Driver.cpp: Contains main() function that uses the Complexno class. To compile this program, all three files need to be put together under the same directory or folder of the project. Note: 1. You are asked to complete the skeleton codes in two files: the interface complexno.h Download complexno.hand the implementation complexno.cpp Download complexno.cpp. 2. You use this driver program (do not change it!) to test your class: Assign3Driver.cpp Download Assign3Driver.cpp. Please copy and paste your sample run as comments at the end of this driver Need help with skelton codes, lost on what to do. complex.cpp // Adds two complex numbers and returns the answer. Complexno Complexno::add(const Complexno& num2) { Complexno answer; answer.real = real + num2.real; answer.complex = complex + num2.complex; return answer; } // (2) --------------------------------- subtract------------------ // Define sub to subtracts two complex numbers and returns the answer. Complexno Complexno::sub(const Complexno& num2) { /* add your codes */ } // (3) --------------------------------- Multiply------------------ // Multiplies two complex numbers and returns this answer. Complexno Complexno::mult(const Complexno& num2) { /* add your codes */ } // Negates a complex number. Complexno Complexno::negate() { Complexno answer; answer.real = -real; answer.complex = -complex; return answer; } // (4) --------------------------------- Magnitude ------------------ // Computes and returns the magnitude of a complex number. double Complexno::magnitude() { /* add your codes */ } // (5) --------------------------------- Print ------------------ // Prints out a complex number in a readable format. void Complexno::shownum() { /* add your codes */ } complex.h #ifndef COMPLEX_H #define COMPLEX_H #include #include using namespace std; class Complexno { public : Complexno(); // Default constructor Complexno(double r); // Second constructor - creates a complex number of equal value to a real. Complexno(double r, double c); // (1) Standard constructor - sets both of the real and complex // components based on parameters. Complexno add(const Complexno& num2); // Adds two complex numbers and returns the answer. /* Your codes to add the prototypes of required methods here. (2) Subtracts two complex numbers and returns the answer. (3) Multiplies two complex numbers and returns this answer. (4) Computes and returns the magnitude of a complex number. (5) Prints out a complex number in a readable format. */ Complexno negate(); // Negates a complex number. void enternum(); // Reads in a complex number from the user. private : double real; // Stores real component of complex number double complex; // Stores complex component of complex number }; // Displays the answer to a complex number operation. void display(Complexno, Complexno, Complexno, char); #endif
Create a class called Complexno for performing arithmetic with complex numbers in C++.
The definition of the class is split into two files.
complexno.h : Contains the declaration of the Complex class, you need to add some methods as asked below.
complexno.cpp : Contains definitions of the functions belonging to class Complexno, you need to finish all the definitions of the class members.
The ADT class
- uses double variables to represent the private data of the class—the real component and the complex component of a complex number.
- provides constructors that enable an object of this class to be initialized when it is declared. The constructor should contain default values in case no initializers are provided.
- provides public member functions for each of the following:
- Addition of two complex numbers. See the solution as an example below.
- Subtraction of two complex numbers.
- Multiplication of two complex numbers.
- Negation of a complex number.
- Computes and returns the magnitude of a complex number.
- Read a complex number from the user
- Prints out a complex number in a readable format.
The third file calls the driver Assign3Driver.cpp Download Assign3Driver.cpp: Contains main() function that uses the Complexno class. To compile this program, all three files need to be put together under the same directory or folder of the project.
Note:
1. You are asked to complete the skeleton codes in two files: the interface complexno.h Download complexno.hand the implementation complexno.cpp Download complexno.cpp.
2. You use this driver program (do not change it!) to test your class: Assign3Driver.cpp Download Assign3Driver.cpp. Please copy and paste your sample run as comments at the end of this driver
Need help with skelton codes, lost on what to do.
complex.cpp
// Adds two complex numbers and returns the answer.
Complexno Complexno::add(const Complexno& num2) {
Complexno answer;
answer.real = real + num2.real;
answer.complex = complex + num2.complex;
return answer;
}
// (2) --------------------------------- subtract------------------
// Define sub to subtracts two complex numbers and returns the answer.
Complexno Complexno::sub(const Complexno& num2) {
/*
add your codes
*/
}
// (3) --------------------------------- Multiply------------------
// Multiplies two complex numbers and returns this answer.
Complexno Complexno::mult(const Complexno& num2) {
/*
add your codes
*/
}
// Negates a complex number.
Complexno Complexno::negate() {
Complexno answer;
answer.real = -real;
answer.complex = -complex;
return answer;
}
// (4) --------------------------------- Magnitude ------------------
// Computes and returns the magnitude of a complex number.
double Complexno::magnitude() {
/*
add your codes
*/
}
// (5) --------------------------------- Print ------------------
// Prints out a complex number in a readable format.
void Complexno::shownum() {
/*
add your codes
*/
}
complex.h
#ifndef COMPLEX_H
#define COMPLEX_H
#include <iostream>
#include <cmath>
using namespace std;
class Complexno {
public :
Complexno(); // Default constructor
Complexno(double r); // Second constructor - creates a complex number of equal
value to a real.
Complexno(double r, double c); // (1) Standard constructor - sets both of the
real and complex
// components based on parameters.
Complexno add(const Complexno& num2); // Adds two complex numbers and returns
the answer.
/* Your codes to add the prototypes of required methods here.
(2) Subtracts two complex numbers and returns the answer.
(3) Multiplies two complex numbers and returns this answer.
(4) Computes and returns the magnitude of a complex number.
(5) Prints out a complex number in a readable format.
*/
Complexno negate(); // Negates a complex number.
void enternum(); // Reads in a complex number from the user.
private :
double real; // Stores real component of complex number
double complex; // Stores complex component of complex number
};
// Displays the answer to a complex number operation.
void display(Complexno, Complexno, Complexno, char);
#endif

Step by step
Solved in 4 steps with 4 images

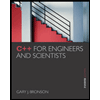
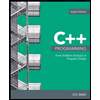
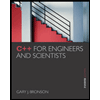
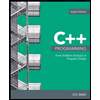