Create a class called PaquetDeCartes that encapsulates a pack of 52 game cards. Inherit your class from the standard class list, and define the following methods: __init__(self, card, shuffle=False) which builds a pack of 52 cards by instantiating cards from the card class. For example, package = PaquetDeCartes(CarteBase), where the CarteBase class is defined in the context of this exercise. By default, cards must be in the order of ace to king, first for spades, then for hearts, then for tiles and finally for clovers. The optional mix argument (default=False) specifies that cards must be initially mixed. mix(self) which allows you to randomly mix the cards in the package. To do this, use the shuffle function of the random module. Draw(n) which returns the first n cards of the package to a list. Note that the cards drawn must be removed from the package and the number of cards returned cannot exceed the number of cards currently in the package. If the packet becomes empty, the method should simply return an empty list. Hints: To remove a card at the beginning of the package, you can use list.pop(0). Note that the constructor of your class must accept any card class that can instantiate from a face number and a kind number, as for the CarteBase class, or any class derived from that class. Be sure to use the list you inherit; do not create another list for your card pack.
Deck of cards in python
Create a class called PaquetDeCartes that encapsulates a pack of 52 game cards. Inherit your class from the standard class list, and define the following methods:
__init__(self, card, shuffle=False) which builds a pack of 52 cards by instantiating cards from the card class. For example, package = PaquetDeCartes(CarteBase), where the CarteBase class is defined in the context of this exercise. By default, cards must be in the order of ace to king, first for spades, then for hearts, then for tiles and finally for clovers. The optional mix argument (default=False) specifies that cards must be initially mixed.
mix(self) which allows you to randomly mix the cards in the package. To do this, use the shuffle function of the random module.
Draw(n) which returns the first n cards of the package to a list. Note that the cards drawn must be removed from the package and the number of cards returned cannot exceed the number of cards currently in the package. If the packet becomes empty, the method should simply return an empty list.
Hints: To remove a card at the beginning of the package, you can use list.pop(0).
Note that the constructor of your class must accept any card class that can instantiate from a face number and a kind number, as for the CarteBase class, or any class derived from that class. Be sure to use the list you inherit; do not create another list for your card pack.
Background to this exercise:
class CarteBase:
names = {1: 'as', 11: 'jack', 12: 'lady', 13: 'king'}
sorts = ['spade', 'heart', 'tile', 'clover']
def __init__(self, m, n):
assert 1 <= m <= 13 and 1 <= n <= 4
self.numface = m
self.numsort = n
def __repr__(self):
return f'{type(self). __name__}({self.numface}, {self.numsort})'
def __str__(self):
return f'{self.nom()} de {self.sorte()}'
def __eq__(self, other):
return self.numface == other.numface and self.numsorte == other.numsorte
def name(self):
# returns the name of the card for the ace, jack, queen and king,
# otherwise the numerical value of its face (2 to 10)
return CarteBase.noms.get(self.numface, str(self.numface))
def sorte(self):
# returns the kind of card (spade, heart, tile or clover)
return CarteBase.kinds[self.numsort-1]
def force(self):
# returns the default force of the card (the numerical value of its face)
return self.numface

Step by step
Solved in 6 steps with 4 images

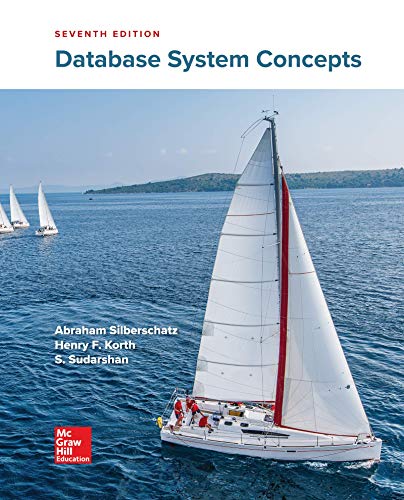
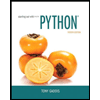
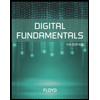
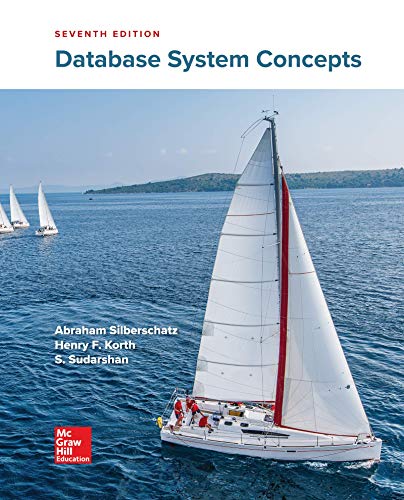
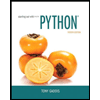
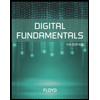
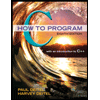
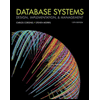
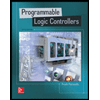