Create a class called Point that has two data members: x- and y-coordinates of the point. Provide a no-argument and a 2-argument constructor. Provide separate get and set functions for the each of the data members i.e. getX, getY, setX, setY. The getter functions should return the corresponding values to the calling function. Provide a display method to display the point in (x, y) format. Make appropriate functions const. Derive a class Circle from this Point class that has an additional data member: radius of the circle. The point from which this circle is derived represents the center of circle. Provide a no-argument constructor to initialize the radius and center coordinates to 0. Provide a 2-argument constructor: one argument to initialize the radius of circle and the other argument to initialize the center of circle (provide an object of point class in the second argument). Provide a 3-argument constructor that takes three floats to initialize the radius, x-, and y-coordinates of the circle. Provide setter and getter functions for radius of the circle. Provide two functions to determine the radius and circumference of the circle. Write the main function to test this class.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Create a class called Point that has two data members: x- and y-coordinates of the point. Provide a no-argument and a 2-argument constructor. Provide separate get and set functions for the each of
the data members i.e. getX, getY, setX, setY. The getter functions should return the corresponding values to the calling function. Provide a display method to display the point in (x, y) format. Make appropriate functions const.
Derive a class Circle from this Point class that has an additional data member: radius of the circle. The point from which this circle is derived represents the center of circle. Provide a no-argument constructor to initialize the radius and center coordinates to 0. Provide a 2-argument constructor: one argument to initialize the radius of circle and the other argument to initialize the center of circle (provide an object of point class in the second argument). Provide a 3-argument constructor that takes
three floats to initialize the radius, x-, and y-coordinates of the circle. Provide setter and getter functions for radius of the circle. Provide two functions to determine the radius and circumference of the circle.
Write the main function to test this class.
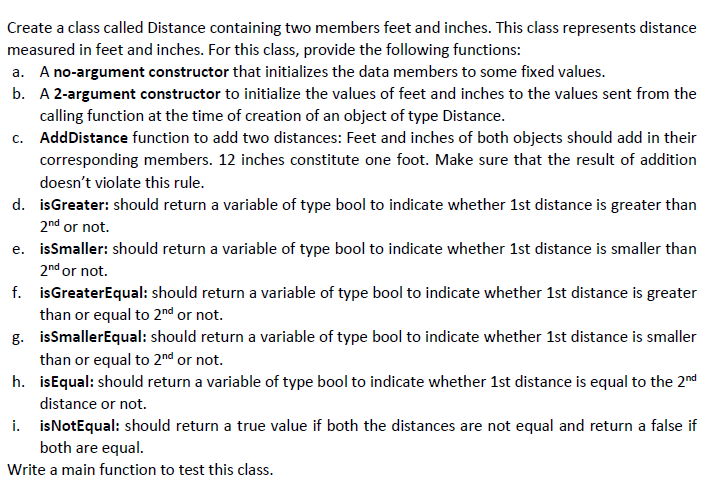

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

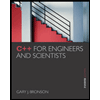
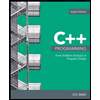
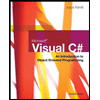
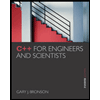
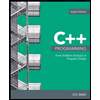
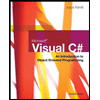