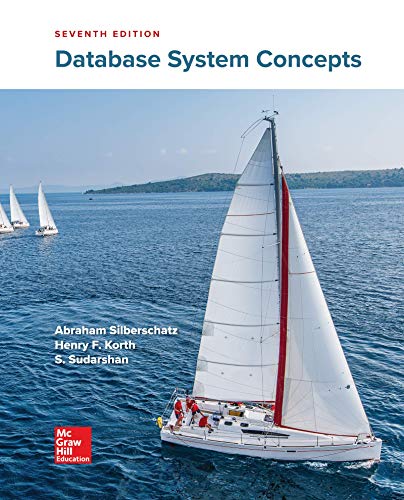
Create a class Student with the fields: name (a String) and gpa (a double).
Create a linked list of Student objects, called students using the LinkedList class from java.util.
Sort the linked list students “by length” of name (see Sect. 11.3 in textbook) using the sort method from
class Collections.
Sort the initial linked list students by gpa using the sort method from class Collections.
Apply the toArray() method from class LinkedList to the initial linked list students and sort the
obtained array “by length” of name using the sort method from class Arrays.
After each sort, print the result.
Do NOT use the sort method from the List interface (also available from a LinkedList).

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- Given main.py and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insert_in_descending_order() method to insert new IntNodes into the IntList in descending order. Ex. If the input is: 3 4 2 5 1 6 7 9 8 the output is: 9 8 7 6 5 4 3 2 1 main.py(can not edit) from IntNode import IntNodefrom IntList import IntList if __name__ == "__main__": int_list = IntList() input_line = input() input_strings = input_line.split(' ') for num_string in input_strings: # Convert from string to integer num = int(num_string) # Insert into linked list in descending order new_node = IntNode(num) int_list.insert_in_descending_order(new_node) int_list.print_int_list() IntNode.py(can not edit) class IntNode: def __init__(self, initial_data, next = None, prev = None): self.data = initial_data self.next = next self.prev = prev IntList.py(need to edit)arrow_forwardHas to be done in Java. Import ArrayList.arrow_forwardImplement a linked list of integers as aclass LinkedList. Build the following methods:✓ print that prints the content of the linked list;✓ addFirst that adds a new node to the beginning (the head) of thelinked list;✓ addLast that adds a new node to the end (the tail) of the linked list;✓ indexOf that finds a specific node by its value, and returns node’sindex (node’s position from the left in the linked list); if the valueis not present in the linked list, it returns −1;✓ deleteFirst that deletes the first node in the linked list;✓ deleteLast that deletes the last node in the linked list.Test your class creating a list in the main and1) adding one by one nodes 2, 4, 8 to the tail;2) adding nodes -2, -8 to the head;3) adding a node 9 to the tail;4) printing the list;5) printing indexOf(4);6) printing contains(9);7) deleting one by one all the nodes in the list – either from the tail orfrom the head – and printing the result after each deletion. THEN AFTER THIS Add a new method IN THE…arrow_forward
- please fix code to match "enter patients name in lbs" thank you import java.util.LinkedList;import java.util.Queue;import java.util.Scanner; interface Patient { public String displayBMICategory(double bmi); public String displayInsuranceCategory(double bmi);} public class BMI implements Patient { public static void main(String[] args) { /* * local variable for saving the patient information */ double weight = 0; String birthDate = "", name = ""; int height = 0; Scanner scan = new Scanner(System.in); String cont = ""; Queue<String> patients = new LinkedList<>(); // do while loop for keep running program till user not entered q do { System.out.print("Press Y for continue (Press q for exit!) "); cont = scan.nextLine(); if (cont.equalsIgnoreCase("q")) { System.out.println("Thank you for using BMI calculator"); break;…arrow_forwardIn python. Write a LinkedList class that has recursive implementations of the add and remove methods. It should also have recursive implementations of the contains, insert, and reverse methods. The reverse method should not change the data value each node holds - it must rearrange the order of the nodes in the linked list (by changing the next value each node holds). It should have a recursive method named to_plain_list that takes no parameters (unless they have default arguments) and returns a regular Python list that has the same values (from the data attribute of the Node objects), in the same order, as the current state of the linked list. The head data member of the LinkedList class must be private and have a get method defined (named get_head). It should return the first Node in the list (not the value inside it). As in the iterative LinkedList in the exploration, the data members of the Node class don't have to be private. The reason for that is because Node is a trivial class…arrow_forwardAdd more methods to the singly linked list class then test them• search(e) // Return one node with 3 values (stuID, stuName, stuScore) which matches agiven key e (studentID).• addAfter(e, stuID, stuName, stuScore) //Add a new node with 3 values (stuID,stuName, stuScore) after the node with the key e (studentID).• removeAt(e) //Remove a node which matches a given key e (studentID)• count() //Return a number of nodes of list.• update(stuID, stuName, stuScore) //Update the values of one node three codes below two in pictures one,typed out. public class SlinkedList<A,B,C> { private Node head; private Node tail; private int size; public SlinkedList(){ head=null; tail=null; size=0; } public int getSize(){ return size; } public boolean isEmpty(){ return size == 0; } public A getFirstStuId(){ if(isEmpty()) return null; return (A) head.getStuID();}public B getFirstStuName(){ if(isEmpty())…arrow_forward
- JAVA please Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Code provided in the assignment ItemNode.java:arrow_forwardGiven main.py and a PersonNode class, complete the PersonList class by writing find_first() and find_last() methods at the end of the PersonList.py file. The find_first() method should find the first occurrence of an age value in the linked list and return the corresponding node. Similarly, the find_last() method should find the last occurrence of the age value in the linked list and return the corresponding node. For both methods, if the age value is not found, None should be returned. The program will replace the name value of each found node with a new name. Ex. If the input is: Alex 23 Tom 41 Michelle 34 Vicky 23 -1 23 Connor 34 Michela main.py(can not edit) from PersonNode import PersonNodefrom PersonList import PersonList if __name__ == "__main__": person_list = PersonList() # Read input lines until encountering '-1' input_line = input() while input_line != '-1': split_input = input_line.split(' ') name = split_input[0] age =…arrow_forwardImplement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 3. Define TestSLinkedList Classa. Declare an instance of the Single List class.b. Test all the methods of the Single List class.arrow_forward
- Given the source code of linked List, answer the below questions(image): A. Fill out the method printList that print all the values of the linkedList: Draw the linked list. public void printList() { } // End of print method B. Write the lines to insert 10 at the end of the linked list. You must draw the final linked List. Notice that you can’t use second or third nodes. Feel free to define a new node. Assume you have only a head node C. Write the lines to delete node 2. You must draw the final linked list. Notice that you can’t use second or third node. Feel free to define a new node. Assume you have only a head nodearrow_forwardThis is using Data Structures in Javaarrow_forwardJavaarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
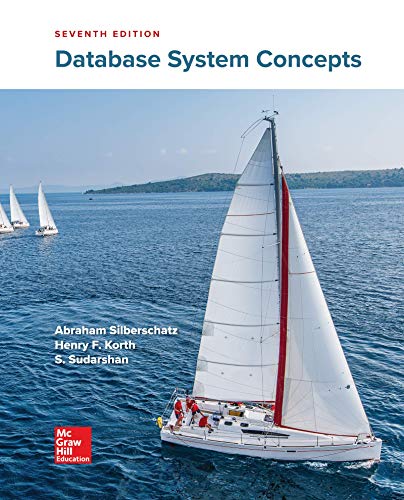
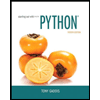
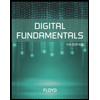
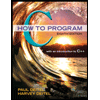
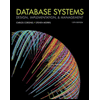
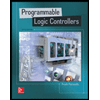