Create a dictionary (order_dict) that the key is the burger number, and the value is a list of the quantities. For example: order_dict which is {'1': [10, 3, 78], '2': [35, 0, 65], '3': [9, 23, 0], '4': [0, 19, 43], '5': [43, 0, 21]} Create a dictionary (total_order_dict) that the key is the burger number, and the value is the total of the quantities for that burger. For example: total_order_dict which is {'1': 91, '2': 100, '3': 32, '4': 62, '5': 64} Use "assert to test the values of the total_order_dict For example: expected_result_ototal_order_dict = {'1': 91, '2': 100, '3': 32, '4': 62, '5': 64} assert actual_result_ototal_order_dict['1'] == expected_result_ototal_order_dict['1'] , "The actual result is not the \ same as expected result for the order number 1!" (and test the other elements) The function can be like: def test_sum(): #Testing assert actual_result_ototal_order_dict['1'] == 91, "The actual result is not the \ same as expected result for the order number 1!" Write this dictionary in a csv file which the first is the key (number of the burger ) and the next number after comma is the value ( the total of the quantities for that burger) you can use like this: def write_order_csv(actual_result_ototal_order_dict,outputFile): with open(outputFile, 'w') as outfile: for key in actual_result_ototal_order_dict.keys(): outfile.write("%s,%s\n"%(key,actual_result_ototal_order_dict[key])) #or writer = csv.writer(outfile) writer.writerow(key + actual_result_ototal_order_dict[key]) You main function can be like: if __name__ == "__main__": actual_result_ototal_order_dict = read_order("order.csv") test_sum() print("Everything passed") write_order_csv(actual_result_ototal_order_dict,"outputfile.csv") My code is import csv order_dict = {'1': [10, 3, 78], '2': [35, 0, 65], '3': [9, 23, 0], '4': [0, 19, 43], '5': [43, 0, 21]} total_order_dict = {} def read_file(): with open ("order.csv",'r') as file: reader = csv.reader(file) for key, values in order_dict.items(): order_dict[key] = sum(values) print(key,sum(values)) total_order_dict = order_dict return total_order_dict def testing_value(): assert total_order_dict["2"] == 100, "The total results is not equal with order_dict" def write_file(read_file): with open ("order.csv",'w') as file: for key in total_order_dict(): read_file. write(key,",",values) if __name__ == "__main__": read_file() testing_value() write_file(read_file) please modify my code to be the same as the output
- Create a dictionary (order_dict) that the key is the burger number, and the value is a list of the quantities.
For example: order_dict which is {'1': [10, 3, 78], '2': [35, 0, 65], '3': [9, 23, 0], '4': [0, 19, 43], '5': [43, 0, 21]}
- Create a dictionary (total_order_dict) that the key is the burger number, and the value is the total of the quantities for that burger.
For example: total_order_dict which is {'1': 91, '2': 100, '3': 32, '4': 62, '5': 64}
- Use "assert to test the values of the total_order_dict
For example:
expected_result_ototal_order_dict = {'1': 91, '2': 100, '3': 32, '4': 62, '5': 64}
assert actual_result_ototal_order_dict['1'] == expected_result_ototal_order_dict['1'] , "The actual result is not the \
same as expected result for the order number 1!"
(and test the other elements)
The function can be like:
def test_sum():
#Testing
assert actual_result_ototal_order_dict['1'] == 91, "The actual result is not the \
same as expected result for the order number 1!"
- Write this dictionary in a csv file which the first is the key (number of the burger ) and the next number after comma is the value ( the total of the quantities for that burger)
you can use like this:
def write_order_csv(actual_result_ototal_order_dict,outputFile):
with open(outputFile, 'w') as outfile:
for key in actual_result_ototal_order_dict.keys():
outfile.write("%s,%s\n"%(key,actual_result_ototal_order_dict[key]))
#or
writer = csv.writer(outfile)
writer.writerow(key + actual_result_ototal_order_dict[key])
You main function can be like:
if __name__ == "__main__":
actual_result_ototal_order_dict = read_order("order.csv")
test_sum()
print("Everything passed")
write_order_csv(actual_result_ototal_order_dict,"outputfile.csv")
My code is
import csv
order_dict = {'1': [10, 3, 78], '2': [35, 0, 65], '3': [9, 23, 0], '4': [0, 19, 43], '5': [43, 0, 21]}
total_order_dict = {}
def read_file():
with open ("order.csv",'r') as file:
reader = csv.reader(file)
for key, values in order_dict.items():
order_dict[key] = sum(values)
print(key,sum(values))
total_order_dict = order_dict
return total_order_dict
def testing_value():
assert total_order_dict["2"] == 100, "The total results is not equal with order_dict"
def write_file(read_file):
with open ("order.csv",'w') as file:
for key in total_order_dict():
read_file. write(key,",",values)
if __name__ == "__main__":
read_file()
testing_value()
write_file(read_file)
please modify my code to be the same as the output
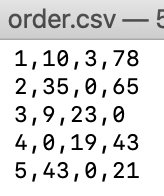


Step by step
Solved in 2 steps with 3 images

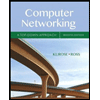
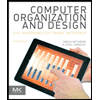
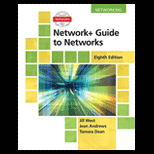
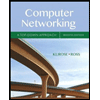
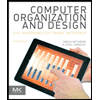
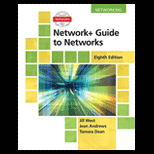
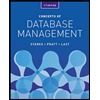
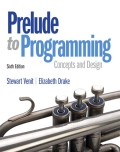
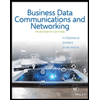