Create a file with the name car.py to store information about a single Car Class. The Car object needs to include the following information: Car name Car vin number Car make Import the Car class into the main.py. Inside the main portion of the code, create a Car object, car1, that has the name "Car", vin "111", and the make "Honda". Print the car information to the commandline and make sure that your code is working correctly. Create a file with the name linkedlist.py. This file will contain the linked list class and the node class as well. The linkedlist.py file needs to import the Car class Create a class Node to represent a single node in the linked list. The class will be inside the linkedlist.py file. The Node class will include the following: A next pointer to hold the address of the next node. You need to set this variable to None A car variable to hold the car objects. Test your Node class in the main. Create a Car object car1. Set the name of the car, vin, and model Create a Node object node. Assign node.car to the car1 object Print node.car information to make sure that everything was assigned correctly Now you are ready to implement the LinkedList class Create a LinkedList class The class needs to include a head and a tail, and count. Implement two functions: insert and print_list The insert function takes the car information as input: name, vin, and make. It creates a car object to store that information, then it creates a node to store the car object, then it adds that node to the LinkedList (refer to the code in the lecture to know how to add the node to the LinkedList) The print_list function traverse through the LinkedList and print all car objects. Finally, import the LinkedList class to the main. Create a LinkedList object and test your LinkedList. Call the insert function twice with the following information: ll.insert("car1", 111, "Honda"), ll.insert("car2",222, "Toyota"). Then, call ll.print_list() function to print all the information inside your LinkedList
Create a file with the name car.py to store information about a single Car Class. The Car object needs to include the following information: Car name Car vin number Car make Import the Car class into the main.py. Inside the main portion of the code, create a Car object, car1, that has the name "Car", vin "111", and the make "Honda". Print the car information to the commandline and make sure that your code is working correctly. Create a file with the name linkedlist.py. This file will contain the linked list class and the node class as well. The linkedlist.py file needs to import the Car class Create a class Node to represent a single node in the linked list. The class will be inside the linkedlist.py file. The Node class will include the following: A next pointer to hold the address of the next node. You need to set this variable to None A car variable to hold the car objects. Test your Node class in the main. Create a Car object car1. Set the name of the car, vin, and model Create a Node object node. Assign node.car to the car1 object Print node.car information to make sure that everything was assigned correctly Now you are ready to implement the LinkedList class Create a LinkedList class The class needs to include a head and a tail, and count. Implement two functions: insert and print_list The insert function takes the car information as input: name, vin, and make. It creates a car object to store that information, then it creates a node to store the car object, then it adds that node to the LinkedList (refer to the code in the lecture to know how to add the node to the LinkedList) The print_list function traverse through the LinkedList and print all car objects. Finally, import the LinkedList class to the main. Create a LinkedList object and test your LinkedList. Call the insert function twice with the following information: ll.insert("car1", 111, "Honda"), ll.insert("car2",222, "Toyota"). Then, call ll.print_list() function to print all the information inside your LinkedList
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 7E
Related questions
Question
100%
Create a file with the name car.py to store information about a single Car Class. The Car object needs to include the following information:
Car name
Car vin number
Car make
Import the Car class into the main.py. Inside the main portion of the code, create a Car object, car1, that has the name "Car", vin "111", and the make "Honda". Print the car information to the commandline and make sure that your code is working correctly.
Create a file with the name linkedlist.py. This file will contain the linked list class and the node class as well. The linkedlist.py file needs to import the Car class
Create a class Node to represent a single node in the linked list. The class will be inside the linkedlist.py file. The Node class will include the following:
A next pointer to hold the address of the next node. You need to set this variable to None
A car variable to hold the car objects.
Test your Node class in the main.
Create a Car object car1. Set the name of the car, vin, and model
Create a Node object node. Assign node.car to the car1 object
Print node.car information to make sure that everything was assigned correctly
Now you are ready to implement the LinkedList class
Create a LinkedList class
The class needs to include a head and a tail, and count.
Implement two functions: insert and print_list
The insert function takes the car information as input: name, vin, and make. It creates a car object to store that information, then it creates a node to store the car object, then it adds that node to the LinkedList (refer to the code in the lecture to know how to add the node to the LinkedList)
The print_list function traverse through the LinkedList and print all car objects.
Finally, import the LinkedList class to the main. Create a LinkedList object and test your LinkedList. Call the insert function twice with the following information: ll.insert("car1", 111, "Honda"), ll.insert("car2",222, "Toyota"). Then, call ll.print_list() function to print all the information inside your LinkedList
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
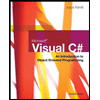
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
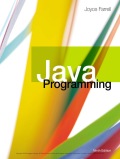
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
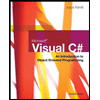
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
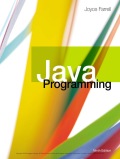
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT