Create a new class in the practicum1 package named ArrayUtilities. Write a method in the class that meets the following requirements: - It should be named "arrayToString" - It should declare a parameter for an int array. - It should return a String in the same format as the example above, e.g. "[1, 2, 3]". If the array is length 0, you should return the string "[]". - It should NOT use the Arrays.toString() method - you should create and return the string yourself. - You may receive a warning from IntelliJ about using string concatenation. You may ignore it. Write a main method to test your method by calling it with one or more small arrays and printing the string(s). Example output: [] [1, 2, 3] [2, 3, 5, 7, 11, 13]
Create a new class in the practicum1 package named ArrayUtilities. Write a method in the class that meets the following requirements: -
It should be named "arrayToString" -
It should declare a parameter for an int array. - It should return a String in the same format as the example above, e.g. "[1, 2, 3]". If the array is length 0, you should return the string "[]". -
It should NOT use the Arrays.toString() method - you should create and return the string yourself. -
You may receive a warning from IntelliJ about using string concatenation. You may ignore it.
Write a main method to test your method by calling it with one or more small arrays and printing the string(s).
Example output: [] [1, 2, 3] [2, 3, 5, 7, 11, 13]

Step by step
Solved in 2 steps with 2 images

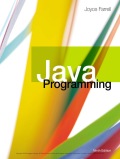
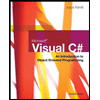
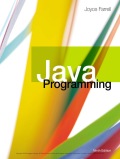
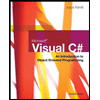