Create a OOP approach for the Tic Tac Toe python code below. # Title: Ysmael Trias - Tic Tac Toe # Student Name: Trias, Ysmael Jr, R # Course and Section: BSCPE 1-3 # Modules to be imported from tkinter import * root = Tk() root.title('Ysmael Trias - Tic Tac Toe') # To set the title of the application root.geometry('510x537') # To set the size of the window of the application player = 'X' stop_game = False def callback(r, c): global player if player == 'X' and states[r][c] == 0 and stop_game==False: b[r][c].configure(text='X', fg='yellow', bg='blue') states[r][c] = 'X' player = 'O' if player == 'O' and states[r][c] == 0 and stop_game==False: b[r][c].configure(text='O', fg='green', bg='red') states[r][c] = 'O' player = 'X' check_for_winner() def check_for_winner(): global stop_game for i in range(3): if states[i][0]==states[i][1]==states[i][2]!=0: b[i][0].configure(bg='black') b[i][1].configure(bg='black') b[i][2].configure(bg='black') stop_game = True for i in range(3): if states[0][i]==states[1][i]==states[2][i]!=0: b[0][0].configure(bg='black') b[1][i].configure(bg='black') b[2][i].configure(bg='black') stop_game = True if states[0][0]==states[1][1]==states[2][2]!=0: b[0][0].configure(bg='black') b[1][1].configure(bg='black') b[2][2].configure(bg='black') stop_game = True if states[2][0]==states[1][1]==states[0][2]!=0: b[2][0].configure(bg='black') b[1][1].configure(bg='black') b[0][2].configure(bg='black') stop_game = True b = [[0, 0, 0], [0, 0, 0], [0, 0, 0]] states = [[0, 0, 0], [0, 0, 0], [0, 0, 0]] def reset(): global stop_game for i in range(3): for j in range(3): b[i][j].configure(text='', fg='black', bg='powder blue') states[i][j] = 0 stop_game = False for i in range(3): for j in range(3): b[i][j] = Button(font=('Calibri', 58, 'bold'), width=4, bg='powder blue', command=lambda r=i, c=j: callback(r, c)) b[i][j].grid(row=i, column=j) reset_game = Button(text='Reset', font=('Calibri', 15, 'bold'), width=4, height=1, fg='black', bg='green', command=lambda :reset()) reset_game.grid(row=3, column=0, columnspan=2, sticky='nsew') exit_game = Button(text='Exit', font=('Calibri', 15, 'bold'), width=4, height=1, fg='black', bg='green', command=lambda :root.destroy()) exit_game.grid(row=3, column=2, columnspan=2, sticky='nsew') root.resizable(0, 0) mainloop()
Create a OOP approach for the Tic Tac Toe python code below. # Title: Ysmael Trias - Tic Tac Toe # Student Name: Trias, Ysmael Jr, R # Course and Section: BSCPE 1-3 # Modules to be imported from tkinter import * root = Tk() root.title('Ysmael Trias - Tic Tac Toe') # To set the title of the application root.geometry('510x537') # To set the size of the window of the application player = 'X' stop_game = False def callback(r, c): global player if player == 'X' and states[r][c] == 0 and stop_game==False: b[r][c].configure(text='X', fg='yellow', bg='blue') states[r][c] = 'X' player = 'O' if player == 'O' and states[r][c] == 0 and stop_game==False: b[r][c].configure(text='O', fg='green', bg='red') states[r][c] = 'O' player = 'X' check_for_winner() def check_for_winner(): global stop_game for i in range(3): if states[i][0]==states[i][1]==states[i][2]!=0: b[i][0].configure(bg='black') b[i][1].configure(bg='black') b[i][2].configure(bg='black') stop_game = True for i in range(3): if states[0][i]==states[1][i]==states[2][i]!=0: b[0][0].configure(bg='black') b[1][i].configure(bg='black') b[2][i].configure(bg='black') stop_game = True if states[0][0]==states[1][1]==states[2][2]!=0: b[0][0].configure(bg='black') b[1][1].configure(bg='black') b[2][2].configure(bg='black') stop_game = True if states[2][0]==states[1][1]==states[0][2]!=0: b[2][0].configure(bg='black') b[1][1].configure(bg='black') b[0][2].configure(bg='black') stop_game = True b = [[0, 0, 0], [0, 0, 0], [0, 0, 0]] states = [[0, 0, 0], [0, 0, 0], [0, 0, 0]] def reset(): global stop_game for i in range(3): for j in range(3): b[i][j].configure(text='', fg='black', bg='powder blue') states[i][j] = 0 stop_game = False for i in range(3): for j in range(3): b[i][j] = Button(font=('Calibri', 58, 'bold'), width=4, bg='powder blue', command=lambda r=i, c=j: callback(r, c)) b[i][j].grid(row=i, column=j) reset_game = Button(text='Reset', font=('Calibri', 15, 'bold'), width=4, height=1, fg='black', bg='green', command=lambda :reset()) reset_game.grid(row=3, column=0, columnspan=2, sticky='nsew') exit_game = Button(text='Exit', font=('Calibri', 15, 'bold'), width=4, height=1, fg='black', bg='green', command=lambda :root.destroy()) exit_game.grid(row=3, column=2, columnspan=2, sticky='nsew') root.resizable(0, 0) mainloop()
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Create a OOP approach for the Tic Tac Toe python code below.
# Title: Ysmael Trias - Tic Tac Toe
# Student Name: Trias, Ysmael Jr, R
# Course and Section: BSCPE 1-3
# Modules to be imported
from tkinter import *
root = Tk()
root.title('Ysmael Trias - Tic Tac Toe') # To set the title of the application
root.geometry('510x537') # To set the size of the window of the application
player = 'X'
stop_game = False
def callback(r, c):
global player
if player == 'X' and states[r][c] == 0 and stop_game==False:
b[r][c].configure(text='X', fg='yellow', bg='blue')
states[r][c] = 'X'
player = 'O'
if player == 'O' and states[r][c] == 0 and stop_game==False:
b[r][c].configure(text='O', fg='green', bg='red')
states[r][c] = 'O'
player = 'X'
check_for_winner()
def check_for_winner():
global stop_game
for i in range(3):
if states[i][0]==states[i][1]==states[i][2]!=0:
b[i][0].configure(bg='black')
b[i][1].configure(bg='black')
b[i][2].configure(bg='black')
stop_game = True
for i in range(3):
if states[0][i]==states[1][i]==states[2][i]!=0:
b[0][0].configure(bg='black')
b[1][i].configure(bg='black')
b[2][i].configure(bg='black')
stop_game = True
if states[0][0]==states[1][1]==states[2][2]!=0:
b[0][0].configure(bg='black')
b[1][1].configure(bg='black')
b[2][2].configure(bg='black')
stop_game = True
if states[2][0]==states[1][1]==states[0][2]!=0:
b[2][0].configure(bg='black')
b[1][1].configure(bg='black')
b[0][2].configure(bg='black')
stop_game = True
b = [[0, 0, 0],
[0, 0, 0],
[0, 0, 0]]
states = [[0, 0, 0],
[0, 0, 0],
[0, 0, 0]]
def reset():
global stop_game
for i in range(3):
for j in range(3):
b[i][j].configure(text='', fg='black', bg='powder blue')
states[i][j] = 0
stop_game = False
for i in range(3):
for j in range(3):
b[i][j] = Button(font=('Calibri', 58, 'bold'), width=4, bg='powder blue', command=lambda r=i, c=j: callback(r, c))
b[i][j].grid(row=i, column=j)
reset_game = Button(text='Reset', font=('Calibri', 15, 'bold'), width=4, height=1, fg='black', bg='green', command=lambda :reset())
reset_game.grid(row=3, column=0, columnspan=2, sticky='nsew')
exit_game = Button(text='Exit', font=('Calibri', 15, 'bold'), width=4, height=1, fg='black', bg='green', command=lambda :root.destroy())
exit_game.grid(row=3, column=2, columnspan=2, sticky='nsew')
root.resizable(0, 0)
mainloop()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Recommended textbooks for you
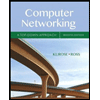
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
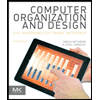
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
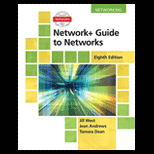
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
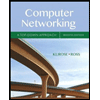
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
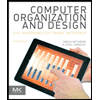
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
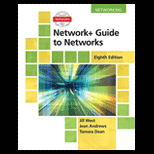
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
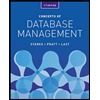
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
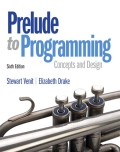
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
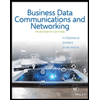
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY