Create a php file based on this table. If the search keyword is "*", the program should display all employees in the table. Your IP: $ip\n"; $IPv4 = explode(".", $ip); if (($IPv4[0] == '10') || ($IPv4[0] == '131' && $IPv4[1] == '125')) { echo "You are from Kean University."; } else { echo "You are NOT from Kean University."; } $keyword = isset($_POST["keyword"]) ? $_POST["keyword"] : ''; $conn = mysqli_connect($servername, $username, $password, $dbname); if (!$conn) { die("Connection failed: " . mysqli_connect_error()); } if ($keyword === '*') { $query = "SELECT * FROM EMPLOYEE"; $result = mysqli_query($conn, $query); if ($result) { $employeeCount = mysqli_num_rows($result); echo "There are $employeeCount employee(s) in the database."; if ($employeeCount > 0) { displayEmployeeTable($result); } else { echo "No records found in the database."; } mysqli_free_result($result); } else { echo "Something went wrong with the SQL query: " . mysqli_error($conn); } } else { $query = "SELECT * FROM EMPLOYEE WHERE Address LIKE '%$keyword%'"; $result = mysqli_query($conn, $query); $employeeCount = mysqli_num_rows($result); echo "There are $employeeCount employee(s) in the database that the address contains the search keyword '$keyword'."; } $result = mysqli_query($conn, $query); if ($result) { if ($keyword === '*' || mysqli_num_rows($result) > 0) { $totalSalary = 0; $employeeCount = 0; echo " ID Login Password Name Role Gender Salary Address "; while ($row = mysqli_fetch_assoc($result)) { $employeeCount++; $genderClass = ($row["gender"] == 'F') ? "red" : "blue"; echo " " . $row["employee_id"] . " " . $row["login"] . " " . $row["password"] . " " . $row["name"] . " " . $row["role"] . " " . $row["gender"] . ""; if (is_null($row["salary"])) { echo " NULL "; } else { echo "" . $row["salary"] . ""; $totalSalary += $row["salary"]; } echo "" . $row["Address"] . " "; } echo ""; if ($keyword !== '*') { $averageSalary = ($employeeCount > 0) ? $totalSalary / $employeeCount : 0; echo "Average salary: $averageSalary"; } } else { echo "No result found for the keyword $keyword"; } mysqli_free_result($result); } else { echo "Something wrong with the SQL query: " . mysqli_error($conn); } mysqli_close($conn);
Create a php file based on this table. If the search keyword is "*", the program should display all employees in the table.
<?php
include "dbconfig.php";
$ip = $_SERVER['REMOTE_ADDR'];
echo "<br>Your IP: $ip\n";
$IPv4 = explode(".", $ip);
if (($IPv4[0] == '10') || ($IPv4[0] == '131' && $IPv4[1] == '125')) {
echo "<br>You are from Kean University.";
} else {
echo "<br>You are NOT from Kean University.";
}
$keyword = isset($_POST["keyword"]) ? $_POST["keyword"] : '';
$conn = mysqli_connect($servername, $username, $password, $dbname);
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
if ($keyword === '*') {
$query = "SELECT * FROM EMPLOYEE";
$result = mysqli_query($conn, $query);
if ($result) {
$employeeCount = mysqli_num_rows($result);
echo "<p>There are $employeeCount employee(s) in the
if ($employeeCount > 0) {
displayEmployeeTable($result);
} else {
echo "<br>No records found in the database.";
}
mysqli_free_result($result);
} else {
echo "<br>Something went wrong with the SQL query: " . mysqli_error($conn);
}
} else {
$query = "SELECT * FROM EMPLOYEE WHERE Address LIKE '%$keyword%'";
$result = mysqli_query($conn, $query);
$employeeCount = mysqli_num_rows($result);
echo "<p>There are $employeeCount employee(s) in the database that the address contains the search keyword '$keyword'.</p>";
}
$result = mysqli_query($conn, $query);
if ($result) {
if ($keyword === '*' || mysqli_num_rows($result) > 0) {
$totalSalary = 0;
$employeeCount = 0;
echo "<table border='1'>
<tr>
<th>ID</th>
<th>Login</th>
<th>Password</th>
<th>Name</th>
<th>Role</th>
<th>Gender</th>
<th>Salary</th>
<th>Address</th>
</tr>";
while ($row = mysqli_fetch_assoc($result)) {
$employeeCount++;
$genderClass = ($row["gender"] == 'F') ? "red" : "blue";
echo "<tr>
<td>" . $row["employee_id"] . "</td>
<td>" . $row["login"] . "</td>
<td>" . $row["password"] . "</td>
<td>" . $row["name"] . "</td>
<td>" . $row["role"] . "</td>
<td><font color='$genderClass'>" . $row["gender"] . "</font></td>";
if (is_null($row["salary"])) {
echo "<td><font color='red'> NULL </font></td>";
} else {
echo "<td>" . $row["salary"] . "</td>";
$totalSalary += $row["salary"];
}
echo "<td>" . $row["Address"] . "</td>
</tr>";
}
echo "</table>";
if ($keyword !== '*') {
$averageSalary = ($employeeCount > 0) ? $totalSalary / $employeeCount : 0;
echo "<p>Average salary: $averageSalary</p>";
}
} else {
echo "<br><br>No result found for the keyword <b>$keyword</b>";
}
mysqli_free_result($result);
} else {
echo "<br>Something wrong with the SQL query: " . mysqli_error($conn);
}
mysqli_close($conn);



Trending now
This is a popular solution!
Step by step
Solved in 3 steps

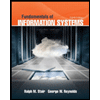
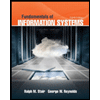