Create a UML Diagram based on the information below. Design a class hierarchy rooted in the class Employee that includes subclasses for HourlyEmployee and SalaryEmployee. The attributes shared in common by these classes include the name, and job title of the employee, plus the accessor and mutator methods needed by those attributes. The salaried employees need an attribute for weekly salary, and the corresponding methods for accessing and changing this variable. The hourly employees should have a pay rate and an hours worked variable. There should be an abstract method called calculateWeeklyPay(), defined abstractly in the superclass and implemented in the subclasses. The salaried worker's pay is just the weekly salary. Pay for an hourly employee is simply hours worked times pay rate.
Create a UML Diagram based on the information below.
- Design a class hierarchy rooted in the class Employee that includes subclasses for HourlyEmployee and SalaryEmployee. The attributes shared in common by these classes include the name, and job title of the employee, plus the accessor and mutator methods needed by those attributes.
- The salaried employees need an attribute for weekly salary, and the corresponding methods for accessing and changing this variable.
- The hourly employees should have a pay rate and an hours worked variable.
- There should be an abstract method called calculateWeeklyPay(), defined abstractly in the superclass and implemented in the subclasses.
- The salaried worker's pay is just the weekly salary.
- Pay for an hourly employee is simply hours worked times pay rate.
For reference following is the java code for some of the classes-
//Employee//
package employee;
public abstract class Employee {
private String name;
private String jobTitle;
public Employee(String name, String jobTitle) {
this.name = name;
this.jobTitle = jobTitle;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getJobTitle() {
return jobTitle;
}
public void setJobTitle(String jobTitle) {
this.jobTitle = jobTitle;
}
abstract public double calculateWeeklyPay();
}
//HourlyEmployeeClass//
package employee;
public class HourlyEmployee extends Employee {
private double payRate;
private double hours;
public HourlyEmployee(String name, String jobTitle) {
super(name, jobTitle);
}
@Override
public double calculateWeeklyPay() {
return payRate * hours;
}
public double getPayRate() {
return payRate;
}
public void setPayRate(double payRate) {
this.payRate = payRate;
}
public double getHours() {
return hours;
}
public void setHours(double hours) {
this.hours = hours;
}
}
// SalaryEmployeeClass//
package employee;
public class SalaryEmployee extends Employee {
private double salary;
public SalaryEmployee(String name, String jobTitle) {
super(name, jobTitle);
}
/**
*
* @return
*/
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
@Override
public double calculateWeeklyPay() {
return salary;
}
}

Step by step
Solved in 2 steps with 1 images

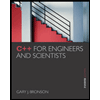
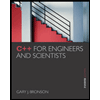