Create an application containing an array that stores eight integers. The application should call five methods that in turn: Display all the integers Display all the integers in reverse order Display the sum of the integers Display all values less than a limiting argument Display all values that are higher than the calculated average value. public class ArrayMethodDemo { public static void main (String args[]) { int[] numbers = {12, 15, 34, 67, 4, 9, 10, 7}; int limit = 12; display(numbers); displayReverse(numbers); displaySum(numbers); displayLessThan(numbers, limit); displayHigherThanAverage(numbers); } public static void display(int[] numbers) { // Write your code here } public static void displayReverse(int[] numbers) { // Write your code here } public static void displaySum(int[] numbers) { // Write your code here } public static void displayLessThan(int[] numbers, int limit) { // Write your code here } public static void displayHigherThanAverage(int[] numbers) { // Write your code here } }
Create an application containing an array that stores eight integers. The application should call five methods that in turn: Display all the integers Display all the integers in reverse order Display the sum of the integers Display all values less than a limiting argument Display all values that are higher than the calculated average value. public class ArrayMethodDemo { public static void main (String args[]) { int[] numbers = {12, 15, 34, 67, 4, 9, 10, 7}; int limit = 12; display(numbers); displayReverse(numbers); displaySum(numbers); displayLessThan(numbers, limit); displayHigherThanAverage(numbers); } public static void display(int[] numbers) { // Write your code here } public static void displayReverse(int[] numbers) { // Write your code here } public static void displaySum(int[] numbers) { // Write your code here } public static void displayLessThan(int[] numbers, int limit) { // Write your code here } public static void displayHigherThanAverage(int[] numbers) { // Write your code here } }
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter9: Advanced Modularization Techniques
Section: Chapter Questions
Problem 2GZ
Related questions
Question
Create an application containing an array that stores eight integers. The application should call five methods that in turn:
- Display all the integers
- Display all the integers in reverse order
- Display the sum of the integers
- Display all values less than a limiting argument
- Display all values that are higher than the calculated average value.
public class ArrayMethodDemo {
public static void main (String args[]) {
int[] numbers = {12, 15, 34, 67, 4, 9, 10, 7};
int limit = 12;
display(numbers);
displayReverse(numbers);
displaySum(numbers);
displayLessThan(numbers, limit);
displayHigherThanAverage(numbers);
}
public static void display(int[] numbers) {
// Write your code here
}
public static void displayReverse(int[] numbers) {
// Write your code here
}
public static void displaySum(int[] numbers) {
// Write your code here
}
public static void displayLessThan(int[] numbers, int limit) {
// Write your code here
}
public static void displayHigherThanAverage(int[] numbers) {
// Write your code here
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
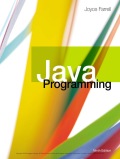
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
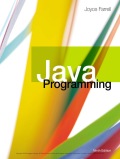
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT