Create an interface MessageEncoder that has a single abstract method encode(plainText), where plainText is the message to be encoded. The method will return the encoded message. Create a class SubstitutionCipher that implements the interface MessageEncoder, as described above. The constructor should have one parameter called shift. Define the method encode so that each letter is shifted by the value in shift. Defne the method encode so that each letter is shifted by the value in shift. For example, if shift is 3, a will be replaced by d, b will be replaced by e, c will be replaced by f, and so on. (Hint: You may wish to define a private method that shifts a single character.) Create a class ShuffleCipher that implements the interface MessageEncoder, as described above. The constructor should have one parameter called n. Define the method encode so that the message is shuffled n times. To perform one shuffle, split the message in half and then take characters from each half alternately. For example, if the message is abcdefghi, the halves are abcde and fghi. The shuffled message is afbgchdie. (Hint: You may wish to define a private method that performs one shuffle.) Create an interface MessageDecoder that has a single abstract method decode(cipherText), where cipherText is the message to be decoded. The method will return the decoded message. Modify the classes SubstitutionCipher and ShuffleCipher, as described in Programming , above, so that they implement MessageDecoder as well as the interface MessageEncoder described above. Finally, write a program that allows a user to encode and decode messages entered on the keyboard.
Java Programming Problem 2 – Message Encoder, Decoder
Create an interface MessageEncoder that has a single abstract method encode(plainText), where plainText is the message to be encoded. The method will return the encoded message.
Create a class SubstitutionCipher that implements the interface MessageEncoder, as described above. The constructor should have one parameter called shift. Define the method encode so that each letter is shifted by the value in shift. Defne the method encode so that each letter is shifted by the value in shift. For example, if shift is 3, a will be replaced by d, b will be replaced by e, c will be replaced by f, and so on. (Hint: You may wish to define a private method that shifts a single character.)
Create a class ShuffleCipher that implements the interface MessageEncoder, as described above. The constructor should have one parameter called n. Define the method encode so that the message is shuffled n times. To perform one shuffle, split the message in half and then take characters from each half alternately. For example, if the message is abcdefghi, the halves are abcde and fghi. The shuffled message is afbgchdie. (Hint: You may wish to define a private method that performs one shuffle.)
Create an interface MessageDecoder that has a single abstract method decode(cipherText), where cipherText is the message to be decoded. The method will return the decoded message. Modify the classes SubstitutionCipher and ShuffleCipher, as described in Programming , above, so that they implement MessageDecoder as well as the interface MessageEncoder described above. Finally, write a program that allows a user to encode and decode messages entered on the keyboard.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

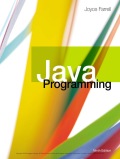
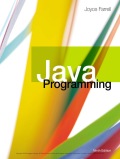