Create an object of type FitnessExperiment that stores an array of StepsFitnessTracker, DistanceFitnessTracker, and HeartRateFitnessTracker objects. You could use code such as the following to initialise the array containing the fitness tracker measurements: FitnessTracker[] trackers = { new StepsFitnessTracker("steps", new Steps(230)), new StepsFitnessTracker("steps2", new Steps(150)), new StepsFitnessTracker("steps2", new Steps(150)), new HeartRateFitnessTracker("hr", new HeartRate(80)), new HeartRateFitnessTracker("hr", new HeartRate(80)) }; In the FitnessExperiment class, complete the implementation of the methods named getTotalSteps()and printExperimentDetails(). The comments in the code specify how they should operate and provide you with additional hints to get you started. You can use the method getSteps()in StepsFitnessTracker, but think how you will know the real type of each object in the array of fitness trackers (trackers in the example above). FitnessTracker HeartRateFitnessTracker DistanceFitnessTracker StepsFitnessTracker public class FitnessTracker {
Java
Task 1 Create an object of type FitnessExperiment that stores an array of StepsFitnessTracker, DistanceFitnessTracker, and HeartRateFitnessTracker objects. You could use code such as the following to initialise the array containing the fitness tracker measurements: FitnessTracker[] trackers = { new StepsFitnessTracker("steps", new Steps(230)), new StepsFitnessTracker("steps2", new Steps(150)), new StepsFitnessTracker("steps2", new Steps(150)), new HeartRateFitnessTracker("hr", new HeartRate(80)), new HeartRateFitnessTracker("hr", new HeartRate(80)) }; In the FitnessExperiment class, complete the implementation of the methods named getTotalSteps()and printExperimentDetails(). The comments in the code specify how they should operate and provide you with additional hints to get you started. You can use the method getSteps()in StepsFitnessTracker, but think how you will know the real type of each object in the array of fitness trackers (trackers in the example above). FitnessTracker HeartRateFitnessTracker DistanceFitnessTracker StepsFitnessTracker
public class FitnessTracker {
// String containing model name of fitness activity tracker
private String modelName;
public FitnessTracker(String modelName) {
this.modelName = modelName;
}
public String getModelName() {
return modelName;
}
/*
@Override
public boolean equals(Object obj) {
// TODO Implement a method to check equality
}
*/
}
public class HeartRateFitnessTracker extends FitnessTracker {
// Cumulative moving average HeartRate
HeartRate avgHeartRate;
// Number of heart rate measurements
int numMeasurements;
public HeartRateFitnessTracker(String modelName, HeartRate heartRate) {
super(modelName);
// Only one HeartRate to begin with; average is equal to single measurement
this.avgHeartRate = heartRate;
this.numMeasurements = 1;
}
public void addHeartRate(HeartRate heartRateToAdd) {
// Calculate cumulative moving average of heart rate
// See https://en.wikipedia.org/wiki/Moving_average
double newHR = heartRateToAdd.getValue();
double cmaHR = this.avgHeartRate.getValue();
double cmaNext = (newHR + (cmaHR * numMeasurements)) / (numMeasurements + 1);
this.avgHeartRate.setValue(cmaNext);
numMeasurements ++;
}
// Getter for average heart rate
public HeartRate getAvgHeartRate() {
return avgHeartRate;
}
public String toString() {
return "Heart Rate Tracker " + getModelName() +
"; Average Heart Rate: " + getAvgHeartRate().getValue() +
", for " + numMeasurements + " measurements";
}
/*
@Override
public boolean equals(Object obj) {
// TODO Implement a method to check equality
}
*/
}
public class StepsFitnessTracker extends FitnessTracker {
// Stores total number of steps
private Steps totalSteps;
public StepsFitnessTracker(String modelName, Steps steps) {
super(modelName);
this.totalSteps = steps;
}
// Add steps to the total
public void addSteps(Steps stepsToAdd) {
int numSteps = this.totalSteps.getValue() + stepsToAdd.getValue();
this.totalSteps.setValue(numSteps);
}
// Getter for total number of steps
public Steps getTotalSteps() {
return totalSteps;
}
public String toString() {
return "Steps Tracker " + getModelName() +
"; Total Steps: " + getTotalSteps().getValue();
}
/*
@Override
public boolean equals(Object obj) {
// TODO Implement a method to check equality
}
*/
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

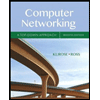
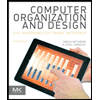
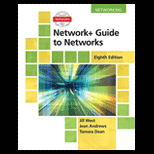
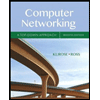
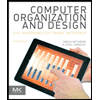
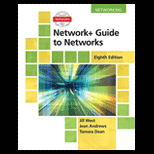
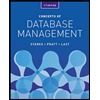
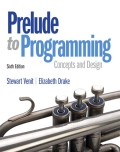
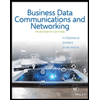