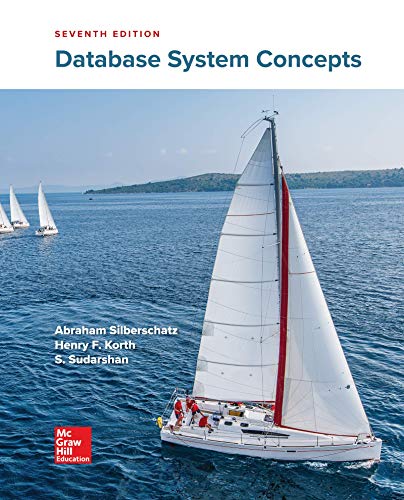
Define a function breadthFirst, which performs a breadth-first traversal on a graph, given a start vertex. This function should return a list of the labels of the vertices in the order in which they are visited. Test the function thoroughly with the case study program.(In Python with comments)
Use this template:
class Vertex:
def __init__(self, label):
self.label = label
self.neighbors = []
def __str__(self):
return self.label
def add_neighbor(self, neighbor):
self.neighbors.append(neighbor)
self.neighbors.sort()
# Graph definition provided, please don't modify any of the provided code
# Add any helper methods if necessary
class Graph:
def __init__(self):
self.vertices = []
# Add a vertex object
def add_vertex(self, vertex):
self.vertices.append(vertex)
# Get index of a vertex with corresponding label
# Return -1 if not found
def get_vertex_index(self, label):
index = 0
for vertex in self.vertices:
if label == str(vertex):
return index
index += 1
return -1
# Find a vertex with corresponding label and return as Vertex object
def find_vertex(self, label):
for vertex in self.vertices:
if label == str(vertex):
return vertex
return None
# Add an edge from a vertex with from_label to a vertex with to_label
# These vertices must already exist in the graph
def add_edge(self, from_label, to_label):
source_vertex = self.find_vertex(from_label)
dest_vertex = self.find_vertex(to_label)
if source_vertex is not None and dest_vertex is not None:
source_vertex.add_neighbor(to_label)
# Perform breadth-first search on a directed graph g starting at vertex with label start_label
# Return a list of vertices' name in the order in which they are visited
# Remove the "pass" statement and implement the function
def breadthFirst(g, start_label):
pass
if __name__ == "__main__":
# TODO: (Optional) your test code here
my_graph = Graph()
vertices = ["0", "1", "2", "3", "4", "5"]
edges = [["0", "1"], ["0", "2"], ["1", "3"], ["1", "4"], ["2", "5"]]
for vertex in vertices:
my_graph.add_vertex(Vertex(vertex))
for edge in edges:
my_graph.add_edge(edge[0], edge[1])
for vertex in my_graph.vertices: # Print out adjacency list
print("Vertex " , vertex, ":", vertex.neighbors)
print(breadthFirst(my_graph, "0")) # Correct Output: ["0","1","2","3","4","5"]

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- Do it in C++ template (STL)arrow_forwardC++ code using dynamic programming. In the graph, determine the cycle's length. A cycle is a path that has the same node as its beginning and ending points and only visits each edge of the path once. The number of edges a cycle visits determines its length. After including the edge of the query, the tree may contain many edges connecting two nodes. Return an array answer of lengthm where answer [i] is the answer to the 1th query. Example 1: Input: n = 3, queries = [[5,3], [4,7], [2,3]] Output: [4,5,3]arrow_forwardImplement a list of employees using a dynamic array of strings.Your solution should include functions for printing list, inserting and removingemployees, and sorting lists (use Bubble sort in C++ language).Keep list of employees sorted within the list after insertion.See the following example:How many employees do you expect to have?5 (Carriage Return)Menu :1 . Print full list of employees2 . Insert new employee3 . Remove employee from list0 . Exit2 (Carriage Return)Input name of new employee : MikeMenu :1 . Print full list of employees2 . Insert new employee3 . Remove employee from list0 . Exit1(Carriage Return)1 . MaryMenu :1 . Print full list of employees2 . Insert new employee3 . Remove employee from list0 . Exitarrow_forward
- 5. The following function indexCounter aims to find and return all the indices of the element x in a list L. if x is not in the list L, return an empty list. However, the implementation has a flaw, so that many times it will return error message. Can you fix the flaw? def indexCounter(L, x): indexList [] startIndex = 0 while startIndex < len(L): indexList.append (L.index (x, startIndex)) startIndex = L.index (x, startIndex) + 1 return indexListarrow_forwardThis is in c++ Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. Don't print the dummy head node. Example input : 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 output: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 main.cpp: #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the PrintNodeData() method// to print the entire linked list //…arrow_forwardJava Programming language Please help me with this. Thanks in advance.arrow_forward
- Data Structure and algorithms ( in Java ) Please solve it urgent basis: Make a programe in Java with complete comments detail and attach outputs image: Question is inside the image also: a). Write a function to insert elements in the sorted manner in the linked list. This means that the elements of the list will always be in ascending order, whenever you insert the data. For example, After calling insert method with the given data your list should be as follows: Insert 50 List:- 50 Insert 40 List:- 40 50 Insert 25 List:- 25 40 50 Insert 35 List:- 25 35 40 50 Insert 40 List:- 25 35 40 40 50 Insert 70 List:- 25 35 40 50 70 b). Write a program…arrow_forwardImplement a function void printAll(void) to print all the items of the list.arrow_forwardImplement a simple linked list in Python (Write source code and show output) with basic linked list operations like: (a) create a sequence of nodes and construct a linear linked list. (b) insert a new node in the linked list. (b) delete a particular node in the linked list. (c) modify the linear linked list into a circular linked list. Use this template: class Node:def __init__(self, val=None):self.val = valself.next = Noneclass LinkedList:"""TODO: Remove the "pass" statements and implement each methodAdd any methods if necessaryDON'T use a builtin list to keep all your nodes."""def __init__(self):self.head = None # The head of your list, don't change its name. It shouldbe "None" when the list is empty.def append(self, num): # append num to the tail of the listpassdef insert(self, index, num): # insert num into the given indexpassdef delete(self, index): # remove the node at the given index and return the deleted value as an integerpassdef circularize(self): # Make your list circular.…arrow_forward
- Suppose a node of a linked list is defined as follows in your program: typedef struct{ int data; struct Node* link; } Node; Write the definition of a function, printOdd(), which displays all the odd numbers of a linked list. What will be the time complexity of printOdd()? Express the time complexity in terms of Big-O notation.arrow_forwardCode in Python:arrow_forwardJava programming language I have to create a remove method that removes the element at an index (ind) or space in an array and returns it. Thanks! I have to write the remove method in the code below. i attached the part where i need to write it. public class ourArrayList<T>{ private Node<T> Head = null; private Node<T> Tail = null; private int size = 0; //default constructor public ourArrayList() { Head = Tail = null; size = 0; } public int size() { return size; } public boolean isEmpty() { return (size == 0); } //implement the method add, that adds to the back of the list public void add(T e) { //HW TODO and TEST //create a node and assign e to the data of that node. Node<T> N = new Node<T>();//N.mData is null and N.next is null as well N.setsData(e); //chain the new node to the list //check if the list is empty, then deal with the special case if(this.isEmpty()) { //head and tail refer to N this.Head = this.Tail = N; size++; //return we are done.…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
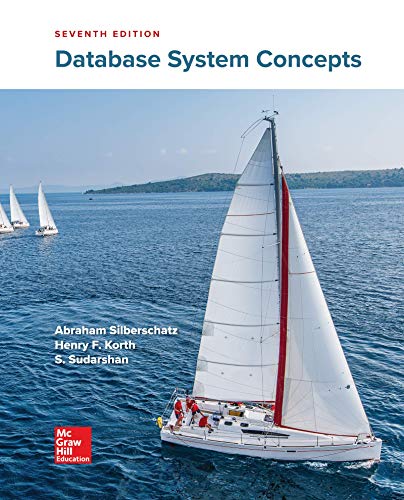
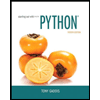
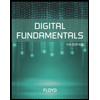
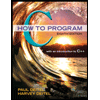
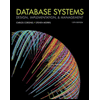
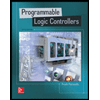