Define a function called create_pattern_dictionary (colours_list) which takes a list of colour names as a parameter and creates a dictionary containing 9 items by looping through each element in the colours list and creating a corresponding key value item. The function returns a pattern dictionary where the key is the pattern number (an integer in the range 0 - 8 inclusive) and the value is a tuple containing two integers and a colour name. The first integer is a row number (0 - 2 inclusive) and the second number is a column number (0-2) inclusive) For example, the following code fragment: colours ['red', 'green', 'blue', 'aqua', 'brown', 'darkseagreen', 'purple', 'lavender', 'magenta'] pattern_dictionary = create_pattern_dictionary(colours) print (pattern_dictionary) produces: (0: (0, 0, 'red'), 1: (0, 1, 'green'), 2: (0, 2, 'blue'), 3: (1, 0, 'aqua'), 4: (1, 1, 'brown'), 5: (1, 2, 'darkseagreen'), 6: (2, 0, 'purple'), 7: (2, 1, 'lavender'), 8: (2, 2, 'magenta')} For example: Test Result (0, 0, 'red') colours ['red', 'green', 'blue', 'aqua', 'brown', 'darkseagreen', 'purple', 'lavender', 'magenta'] pattern_dictionary create_pattern_dictionary(colours) 1 (0, 1, 'green') for key in sorted (pattern_dictionary.keys()): 2 (0, 2, 'blue') print (key, pattern_dictionary [key]) 3 (1, 0, 'aqua') 4 (1, 1, 'brown') 5 (1, 2, 'darkseagreen') 6 (2, 0, 'purple') 7 (2, 1, 'lavender') 8 (2, 2, 'magenta') colours = ['blanchedalmond', 'darkseagreen', 'orange', 'turquoise', 'blue', 'teal', 'yellow', 'brown', 'gray'] pattern_dictionary = create_pattern_dictionary (colours) print (type(pattern_dictionary [0])) values pattern_dictionary [0] print (type(values[0])) print (type(values[1])) print (type(values[2]))
Define a function called create_pattern_dictionary (colours_list) which takes a list of colour names as a parameter and creates a dictionary containing 9 items by looping through each element in the colours list and creating a corresponding key value item. The function returns a pattern dictionary where the key is the pattern number (an integer in the range 0 - 8 inclusive) and the value is a tuple containing two integers and a colour name. The first integer is a row number (0 - 2 inclusive) and the second number is a column number (0-2) inclusive) For example, the following code fragment: colours ['red', 'green', 'blue', 'aqua', 'brown', 'darkseagreen', 'purple', 'lavender', 'magenta'] pattern_dictionary = create_pattern_dictionary(colours) print (pattern_dictionary) produces: (0: (0, 0, 'red'), 1: (0, 1, 'green'), 2: (0, 2, 'blue'), 3: (1, 0, 'aqua'), 4: (1, 1, 'brown'), 5: (1, 2, 'darkseagreen'), 6: (2, 0, 'purple'), 7: (2, 1, 'lavender'), 8: (2, 2, 'magenta')} For example: Test Result (0, 0, 'red') colours ['red', 'green', 'blue', 'aqua', 'brown', 'darkseagreen', 'purple', 'lavender', 'magenta'] pattern_dictionary create_pattern_dictionary(colours) 1 (0, 1, 'green') for key in sorted (pattern_dictionary.keys()): 2 (0, 2, 'blue') print (key, pattern_dictionary [key]) 3 (1, 0, 'aqua') 4 (1, 1, 'brown') 5 (1, 2, 'darkseagreen') 6 (2, 0, 'purple') 7 (2, 1, 'lavender') 8 (2, 2, 'magenta') colours = ['blanchedalmond', 'darkseagreen', 'orange', 'turquoise', 'blue', 'teal', 'yellow', 'brown', 'gray'] pattern_dictionary = create_pattern_dictionary (colours) print (type(pattern_dictionary [0])) values pattern_dictionary [0] print (type(values[0])) print (type(values[1])) print (type(values[2]))
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter13: Structures
Section13.5: Dynamic Data Structure Allocation
Problem 2E
Related questions
Question
![Define a function called create_pattern_dictionary (colours_list) which takes a list of colour names as a parameter and creates a dictionary containing 9 items by looping through
each element in the colours list and creating a corresponding key value item. The function returns a pattern dictionary where the key is the pattern number (an integer in the range 0 - 8
inclusive) and the value is a tuple containing two integers and a colour name. The first integer is a row number (0 - 2 inclusive) and the second number is a column number (0 - 2
inclusive) For example, the following code fragment:
colours = ['red', 'green', 'blue',
'aqua', 'brown', 'darkseagreen',
'purple', 'lavender', 'magenta']
pattern_dictionary = create_pattern_dictionary (colours)
print (pattern_dictionary)
produces:
{0: (0, 0, 'red'), 1: (0, 1, 'green'), 2: (0, 2, 'blue'),
3: (1, 0, 'aqua'), 4: (1, 1, 'brown'), 5: (1, 2, 'darkseagreen'),
6: (2, 0, 'purple'), 7: (2, 1, 'lavender'), 8: (2, 2, 'magenta')}
For example:
Test
Result
0 (0, 0, 'red')
colours = ['red', 'green', 'blue', 'aqua', 'brown', 'darkseagreen', 'purple', 'lavender', 'magenta']
pattern_dictionary = create_pattern_dictionary (colours)
1 (0, 1, 'green')
for key in sorted (pattern_dictionary.keys ()):
2 (0, 2, 'blue')
print(key, pattern_dictionary [key])
3 (1, 0, 'aqua')
4 (1, 1, 'brown')
5 (1, 2, 'darkseagreen')
6 (2, 0, 'purple')
7 (2, 1, 'lavender')
8 (2, 2, 'magenta')
colours = ['blanchedalmond', 'darkseagreen', 'orange', 'turquoise', 'blue', 'teal', 'yellow', 'brown', 'gray'] <class 'tuple'>
pattern_dictionary = create_pattern_dictionary (colours)
print (type(pattern_dictionary [0]))
<class 'int'>
<class 'int'>
<class 'str'>
values = pattern_dictionary [0]
print (type (values[0]))
print (type (values[1]))
print (type(values[2]))](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffdcbcdd1-e8c0-4785-bbe7-bc6d3a4f99de%2F6a1c8d1e-7e68-463b-b22d-638cdff3bd1e%2Fs0uknh_processed.png&w=3840&q=75)
Transcribed Image Text:Define a function called create_pattern_dictionary (colours_list) which takes a list of colour names as a parameter and creates a dictionary containing 9 items by looping through
each element in the colours list and creating a corresponding key value item. The function returns a pattern dictionary where the key is the pattern number (an integer in the range 0 - 8
inclusive) and the value is a tuple containing two integers and a colour name. The first integer is a row number (0 - 2 inclusive) and the second number is a column number (0 - 2
inclusive) For example, the following code fragment:
colours = ['red', 'green', 'blue',
'aqua', 'brown', 'darkseagreen',
'purple', 'lavender', 'magenta']
pattern_dictionary = create_pattern_dictionary (colours)
print (pattern_dictionary)
produces:
{0: (0, 0, 'red'), 1: (0, 1, 'green'), 2: (0, 2, 'blue'),
3: (1, 0, 'aqua'), 4: (1, 1, 'brown'), 5: (1, 2, 'darkseagreen'),
6: (2, 0, 'purple'), 7: (2, 1, 'lavender'), 8: (2, 2, 'magenta')}
For example:
Test
Result
0 (0, 0, 'red')
colours = ['red', 'green', 'blue', 'aqua', 'brown', 'darkseagreen', 'purple', 'lavender', 'magenta']
pattern_dictionary = create_pattern_dictionary (colours)
1 (0, 1, 'green')
for key in sorted (pattern_dictionary.keys ()):
2 (0, 2, 'blue')
print(key, pattern_dictionary [key])
3 (1, 0, 'aqua')
4 (1, 1, 'brown')
5 (1, 2, 'darkseagreen')
6 (2, 0, 'purple')
7 (2, 1, 'lavender')
8 (2, 2, 'magenta')
colours = ['blanchedalmond', 'darkseagreen', 'orange', 'turquoise', 'blue', 'teal', 'yellow', 'brown', 'gray'] <class 'tuple'>
pattern_dictionary = create_pattern_dictionary (colours)
print (type(pattern_dictionary [0]))
<class 'int'>
<class 'int'>
<class 'str'>
values = pattern_dictionary [0]
print (type (values[0]))
print (type (values[1]))
print (type(values[2]))
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
![Define a function called create_pattern_dictionary (colours_list) which takes a list of colour names as a parameter and creates a dictionary containing 9 items by looping through
each element in the colours list and creating a corresponding key: value item. The function returns a pattern dictionary where the key is the pattern number (an integer in the range 0 - 8
inclusive) and the value is a tuple containing two integers and a colour name. The first integer is a row number (0 - 2 inclusive) and the second number is a column number (0 - 2
inclusive) For example, the following code fragment:
colours = ['red', 'green', 'blue',
'aqua', 'brown', 'darkseagreen',
'purple', 'lavender', 'magenta']
pattern_dictionary = create_pattern_dictionary (colours)
print (pattern_dictionary)
produces:
{0: (0, 0, 'red'), 1: (0, 1, 'green'), 2: (0, 2, 'blue'),
3: (1, 0, 'aqua'), 4: (1, 1, 'brown'), 5: (1, 2, 'darkseagreen'),
6: (2, 0, 'purple'), 7: (2, 1, 'lavender'), 8: (2, 2, 'magenta')}
For example:
Test
Result
0 (0, 0, 'red')
colours = ['red', 'green', 'blue', 'aqua', 'brown', 'darkseagreen', 'purple', 'lavender', 'magenta']
pattern_dictionary = create_pattern_dictionary (colours)
1 (0, 1, 'green')
for key in sorted (pattern_dictionary.keys()):
2 (0, 2, 'blue')
print(key, pattern_dictionary [key])
3 (1, 0, 'aqua')
4 (1, 1, 'brown')
5 (1, 2, 'darkseagreen')
6 (2, 0, 'purple')
7 (2, 1, 'lavender')
8 (2, 2, 'magenta')
colours = ['blanchedalmond', 'darkseagreen', 'orange', 'turquoise', 'blue', 'teal', 'yellow', 'brown', 'gray'] <class 'tuple'>
pattern_dictionary = create_pattern_dictionary (colours)
print (type(pattern_dictionary[0]))
<class 'int'>
<class 'int'>
<class 'str'>
values= pattern_dictionary [0]
print (type (values[0]))
print (type(values[1]))
print (type (values [2]))](https://content.bartleby.com/qna-images/question/fdcbcdd1-e8c0-4785-bbe7-bc6d3a4f99de/20effef8-cc24-4ab4-bdef-393020766112/g0sscoh_thumbnail.png)
Transcribed Image Text:Define a function called create_pattern_dictionary (colours_list) which takes a list of colour names as a parameter and creates a dictionary containing 9 items by looping through
each element in the colours list and creating a corresponding key: value item. The function returns a pattern dictionary where the key is the pattern number (an integer in the range 0 - 8
inclusive) and the value is a tuple containing two integers and a colour name. The first integer is a row number (0 - 2 inclusive) and the second number is a column number (0 - 2
inclusive) For example, the following code fragment:
colours = ['red', 'green', 'blue',
'aqua', 'brown', 'darkseagreen',
'purple', 'lavender', 'magenta']
pattern_dictionary = create_pattern_dictionary (colours)
print (pattern_dictionary)
produces:
{0: (0, 0, 'red'), 1: (0, 1, 'green'), 2: (0, 2, 'blue'),
3: (1, 0, 'aqua'), 4: (1, 1, 'brown'), 5: (1, 2, 'darkseagreen'),
6: (2, 0, 'purple'), 7: (2, 1, 'lavender'), 8: (2, 2, 'magenta')}
For example:
Test
Result
0 (0, 0, 'red')
colours = ['red', 'green', 'blue', 'aqua', 'brown', 'darkseagreen', 'purple', 'lavender', 'magenta']
pattern_dictionary = create_pattern_dictionary (colours)
1 (0, 1, 'green')
for key in sorted (pattern_dictionary.keys()):
2 (0, 2, 'blue')
print(key, pattern_dictionary [key])
3 (1, 0, 'aqua')
4 (1, 1, 'brown')
5 (1, 2, 'darkseagreen')
6 (2, 0, 'purple')
7 (2, 1, 'lavender')
8 (2, 2, 'magenta')
colours = ['blanchedalmond', 'darkseagreen', 'orange', 'turquoise', 'blue', 'teal', 'yellow', 'brown', 'gray'] <class 'tuple'>
pattern_dictionary = create_pattern_dictionary (colours)
print (type(pattern_dictionary[0]))
<class 'int'>
<class 'int'>
<class 'str'>
values= pattern_dictionary [0]
print (type (values[0]))
print (type(values[1]))
print (type (values [2]))
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
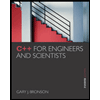
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
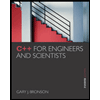
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr