Define a new struct data type “Student” to represent a student record.
Develop a C++ program that reads the student’s name and scores from the attached data file “students.txt” and store them in an array of Student struct objects, then calculate and display each student’s final grade based on the following criteria: midterm exam is counted for 25% of the final grade, final exam is counted for 25% of the final grade and average of 4 labs is counted for 50% of the final grade.
-
The format of the attached data file “students.txt” is ---
//student name
// midterm exam score, final exam score
// lab1 score, lab2 score, lab3 score, lab4 score
-
Define a new struct data type “Student” to represent a student record.
-
Each student’s record should be read from the data file “students.txt” and stored in a variable of Student struct.
-
Create an array of Student struct with size of 24, save all student struct records in this array.
-
Define a function “calculateGrade(…)” which:
-
Needs a Student struct record as parameter.
-
Calculate this student’s final grade (numeric score).
-
Return this student’s final grade (numeric score).
-
-
All data members in the struct should be public.
- No non-constant data should be declared globally
/* *
* Author:
* Date:
* Class:
*/
#include <iostream>
#include <cstdlib>
#include <string>
#include <fstream>
#include <sys/stat.h>
using namespace std;
/* structure */
struct Student {
string firstName;
string lastName;
};
/* function prototype */
double calculateGrade(Student student[]);
/* main function */
int
main(void)
{
Student newStudent;
Student students[24];
int numStudent = 0;
ifstream inFile;
char letterGrade = ' ';
/* open the data file and check whether the file cannot be found; write your code here */
inFile.open("students.txt");
struct stat buf;
if (stat("students.txt", &buf) != 0) {
cerr << "file does not exist\n";
exit(EXIT_FAILURE);
}
cout << "file exists\n";
/* read the first studet's record; write your code here */
while (inFile) {
/* call calculateGrade function to calculate student’s
* final numeric score and update student’s record, write your code here */
/* ignore the '\n' at the end of current student record in the
* data file before reading next student record. write your code here */
/* read next student record. write your code here */
}
/* close the data file */
inFile.close();
for (int i = 0; i < numStudent; i++) {
// determine each student's letter grade (A, B, C, D, or F) write your code here
int student_score = 0;
if (student_score >= 90)
letterGrade = 'A';
if (student_score >= 80)
letterGrade = 'B';
if (student_score >= 70)
letterGrade = 'C';
if (student_score >= 60)
letterGrade = 'D';
else
letterGrade = 'F';
ofstream outFile;
outFile.open("outFile.txt");
outFile << students[i].firstName << " " << students[i].lastName
<< "'s final grade is ";
// display each student’s name, final score and final grade
// according to the expected output, write your code here
// ………
}
exit(EXIT_SUCCESS);
}
double
calculateGrade(Student student[])
{
/* calculate and return student's final numeric score */
return 0.0;
}
**********************
students.txt
***********************
Joe Doe
90.8 89.5
67 89.2 99.0 100.0
Susan F. Smith
95.5 94.0
78.5 90 87.5 57
Sam Grover
78 100.0
79.5 69.4 90.0 88.5
Diane C. Phelps
100 78.0
56.0 69 94.0 78
John Franklin
65 87.0
67.0 96 49.0 87
Derrick Myers
89.8 98.5
76 89.2 93.0 100.0
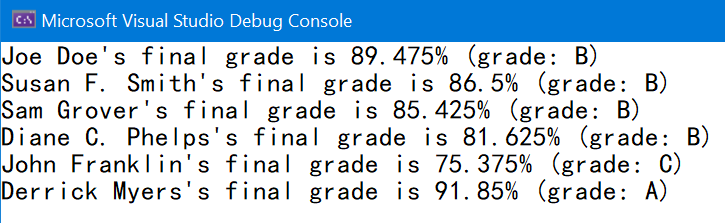

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

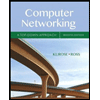
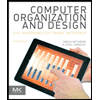
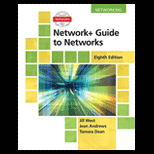
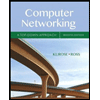
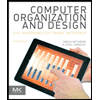
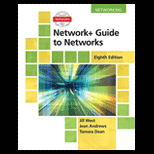
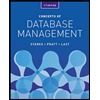
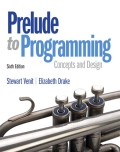
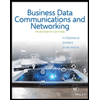