Define a pure abstract base class called BasicShape . The BasicShape class should have the following members: Private Member Variable: area, type double used to hold the shape's area. Public Member Functions: setArea: assigns received argument to area variable. getArea. This function should return the value in the member variable area. calcArea. This function should be a pure virtual function. Next, define a class named Circle . It should be derived from the BasicShape class. It should have the following members: Private Member Variables: centerX, type integer used to hold the x coordinate of the circle’s center. centerY, type integer used to hold the y coordinate of the circle’s center. radius, type double used to hold the circle's radius. Public Member Functions: constructor—accepts values for centerX, centerY, and radius. Should also call the overridden calcArea function described below. getCenterX—returns the value in centerX. getCenterY—returns the value in centerY. calcArea—calculates the area of the circle (area = 3.14159 * radius * radius) and stores the result in the inherited member variable area in base class. Next, define a class named Rectangle. It should be derived from the BasicShape class. It should have the following members: Private Member Variables: width, type double used to hold the width of the rectangle. length, type double used to hold the length of the rectangle. Public Member Functions: constructor—accepts values for width and length. Should also call the overridden calcArea function described below. getWidth—returns the value in width. getLength—returns the value in length. calcArea—calculates the area of the rectangle (area = length * width) and stores the result in the inherited member variable area in base class. Write a main function that will declare pointer array of type BasicShape having size 4 and initialize the array with the objects of type circle, circle, rectangle and rectangle respectively. Then using for loop, display the area of those shapes (circle and rectangle) whose area > 100 . Note: Do not change the names of functions and variables. Otherwise, marks will be deducted. Do not define extra functions
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
plz do it urgent in devc++. and plzz dont reject it
Define a pure abstract base class called BasicShape . The BasicShape class should have the following members:
Private Member Variable:
area, type double used to hold the shape's area.
Public Member Functions:
setArea: assigns received argument to area variable.
getArea. This function should return the value in the member variable area.
calcArea. This function should be a pure virtual function.
Next, define a class named Circle . It should be derived from the BasicShape class. It should have the following members:
Private Member Variables:
centerX, type integer used to hold the x coordinate of the circle’s center.
centerY, type integer used to hold the y coordinate of the circle’s center.
radius, type double used to hold the circle's radius.
Public Member Functions:
constructor—accepts values for centerX, centerY, and radius. Should also call the overridden calcArea function described below.
getCenterX—returns the value in centerX.
getCenterY—returns the value in centerY.
calcArea—calculates the area of the circle (area = 3.14159 * radius * radius) and stores the result in the inherited member variable area in base class.
Next, define a class named Rectangle. It should be derived from the BasicShape class. It should have the following members:
Private Member Variables:
width, type double used to hold the width of the rectangle.
length, type double used to hold the length of the rectangle.
Public Member Functions:
constructor—accepts values for width and length. Should also call the overridden calcArea function described below.
getWidth—returns the value in width.
getLength—returns the value in length.
calcArea—calculates the area of the rectangle (area = length * width) and stores the result in the inherited member variable area in base class.
Write a main function that will declare pointer array of type BasicShape having size 4 and initialize the array with the objects of type circle, circle, rectangle and rectangle respectively. Then using for loop, display the area of those shapes (circle and rectangle) whose area > 100 .
Note: Do not change the names of functions and variables. Otherwise, marks will be deducted. Do not define extra functions

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

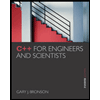
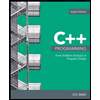
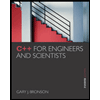
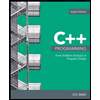
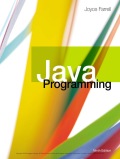
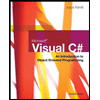