DESCRIPTION Match the output EXACTLY. Enter the old and new consumer price Indices: 238.343 238.250 Inflation rate Is -0.0390204 Try agaln? (y or Y): y Enter the old and new consumer price Indices: 238.250 237.852 Inflation rate is -0.167049 Try agaln? (y or Y): n Average rate is -0.103035 Part 2 1. Here are the original instructions from Part 1. // TODO #1: declare two float variables for the old consumer price index (cpi) and the new cpi // TODO #2: Read in two float values for the cpi and store them in the variables // TODO #3: call the function InflationRate with the two cpis // TODO #4: print the results 2. Put the logic in TODO #2-4 into a loop that asks the user to enter 'y (or 'Y) if there's more data to be entered. 3. Keep a running total of the valld inflation rates and the number of computed rates to calculate the average rate. 4. Print the results after the loop
For this c++ hw part 2 needs to be completed and match with the output displayed for part 2
My code:
//This program calculates the inflation rate given two Consumer Price Index values and prints it to the monitor.
#include <iostream>
using namespace std;
/*
* InflationRate - calculates the inflation rate given the old and new consumer price index
* @param old_cpi: is the consumer price index that it was a year ago
* @param new_cpi: is the consumer price index that it is currently
* @returns the computed inflation rate or 0 if inputs are invalid.
*/
double InflationRate(float old_cpi, float new_cpi);
int main() //C++ programs start by executing the function main
{
// TODO #1: declare two float variables for the old consumer price index (cpi) and the new cpi
float oldCPI;
float newCPI;
float iRate;
// TODO #2: Read in two float values for the cpi and store them in the variables
cout << "Enter the old and new consumer price indices: \n";
cin >> oldCPI >> newCPI;
// TODO #3: call the function InflationRate with the two cpis
iRate = InflationRate(oldCPI, newCPI);
// TODO #4: print the results
cout << "Inflation rate is " << iRate << endl;
// BONUS #1: Put the logic in TODO #2-4 in a loop that asks the user to enter 'y' if there's more data
// BONUS #2: Keep a running total of the valid inflation rates and the number of computed rates to calculate the average rate.
// Print the results after the loop
return 0;
}
// double InflationRate(float old_cpi, float new_cpi)
// precondition: both prices must be greater than 0.0
// postcondition: the inflation rate is returned or 0 for invalid inputs
double InflationRate(float old_cpi, float new_cpi)
{
// TODO: Implement InflationRate to calculate the percentage increase or decrease
// Use (new_cpi - old_cpi) / old_cpi * 100
double results;
if(old_cpi > 0 && new_cpi > 0)
{
results = (new_cpi - old_cpi) / old_cpi * 100;
return results;
}
else
return 0;
}

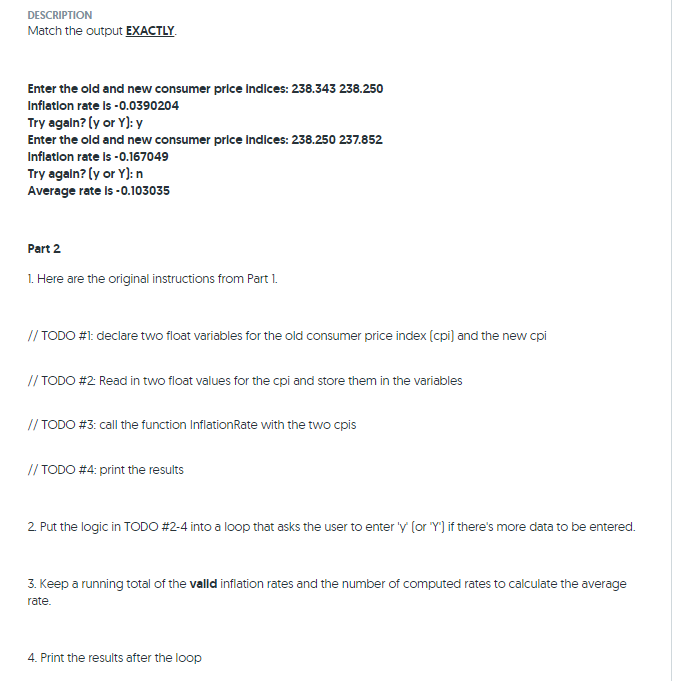

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

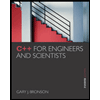
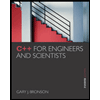