Description: The program starts by asking the user to select either login (L) or sign up (S) feature. Once the user selects a feature, it asks him for (Card Number, then PIN) and after filling the required information for that feature, it shows the above menu for other features. Otherwise, it keeps asking for the required information. Each feature has to be designed using a separate function and it might be called by other functions including the main function. The description of the login feature, sign up feature, and the above features are given below: Sign up Feature – def create(): this feature allows new user to create an account and it saves his information in txt. The required information to be asked in this feature as follows: Card Number: user will enter a card number and should be four digits, each digit is unique and it should not be repeated in the 4-digits card number. This feature will keep running until the user enters the required number with same length and type. PIN: user will enter a PIN number and should be four digits, each digit is unique and it should not be repeated in the 4-digits PIN number. This feature will keep running until the user enters the required number with same length and type. Email: user will enter his KFUPM email (g20XXXXXXX@kfupm.edu.sa) and it should KFUPM email. Otherwise, it will ask again for email with same specifications. Extra Attribute: user needs to add at least one extra attribute (of your own choice) for the created account. After creating your account, you are required to save the information in cardNumber.txt file in same path of your jupyter file. Then, the program will sleep for few seconds and it will redirect you to login feature. Login Feature – def login(): This feature allows the user to login to his saved account after creating the account using the first function (create()). The required information to be asked in this feature as follows: It asks the user to enter his card number and it should be available in same path (cardNumber.txt). The program will check the first line in the cardNumber.txt for the card number. This feature will keep running until the user enters his saved number. Then, the program will check the second line in the cardNumber.txt for the PIN number. This feature will keep running until the user enters his saved number. If the user enters the correct info, the menu in Figure 1 will appear directly. 1 Show Feature – def show(file): this feature allows the user to show his saved account details based on the reading of his .txt file. After doing this step, the program will sleep for few seconds and it will redirect you to the menu again. ChangePIN Feature – def changePinFun(currentPIN, cardNumber, file): this feature allows the user to change his PIN number in the .txt file. Here, you are required to replace the saved PIN by new PIN and it should have same structure. After doing this step, the program will sleep for few seconds and it will redirect you to the menu again. Withdraw Feature – def withdrawFun(money, cardNumber, file): this feature allows the user to withdraw money from his account and then updates the account details in the .txt file accordingly. Remind that, if you don’t have enough money in your account, it will give a notification message and it will ask for new amount until you entered an amount that you can withdraw it. After doing this step, the program will sleep for few seconds and it will redirect you to the menu again. Deposit Feature – def depositFun(file, nMoney, cardNumber): this feature allows the user to deposit money to your account and then updates the account details in the .txt file accordingly. After doing this step, the program will sleep for few seconds and it will redirect you to the menu again. Bills Feature – def payBillFun(file, nMoney, cardNumber): this feature allows the user to pay a bill from his account, deducts this bill from the account and updates the account details in the .txt file accordingly. Enter the name of the bill. Enter the account number of this bill. Enter the value of this bill. Remind that, if you don’t have enough money in your account, it will give a notification message and it will ask to deposit amount to deduct the bill. After doing this step, the program will sleep for few seconds and it will redirect you to the menu again. View Transactions Feature – def viewTransactionsFun(cardNumber): this feature allows the user to show the history of his account based on the .txt file. If there are transactions in the account, it will be presented along with its (date and time). Otherwise, it will show "no transactions". After doing this step, the program will sleep for few seconds and it will redirect you to the menu again. Terminate Feature – def terminateFun(file, nMoney, cardNumber): this feature allows the user to ter-minate the program and at same time it will show the last transactions on the account during the session
- Description:
The
- Sign up Feature – def create(): this feature allows new user to create an account and it saves his information in txt. The required information to be asked in this feature as follows:
- Card Number: user will enter a card number and should be four digits, each digit is unique and it should not be repeated in the 4-digits card number. This feature will keep running until the user enters the required number with same length and type.
- PIN: user will enter a PIN number and should be four digits, each digit is unique and it should not be repeated in the 4-digits PIN number. This feature will keep running until the user enters the required number with same length and type.
- Email: user will enter his KFUPM email (g20XXXXXXX@kfupm.edu.sa) and it should KFUPM email. Otherwise, it will ask again for email with same specifications.
- Extra Attribute: user needs to add at least one extra attribute (of your own choice) for the created account.
After creating your account, you are required to save the information in cardNumber.txt file in same path of your jupyter file. Then, the program will sleep for few seconds and it will redirect you to login feature.
- Login Feature – def login(): This feature allows the user to login to his saved account after creating the account using the first function (create()). The required information to be asked in this feature as follows:
- It asks the user to enter his card number and it should be available in same path (cardNumber.txt). The program will check the first line in the cardNumber.txt for the card number. This feature will keep running until the user enters his saved number.
- Then, the program will check the second line in the cardNumber.txt for the PIN number. This feature will keep running until the user enters his saved number.
- If the user enters the correct info, the menu in Figure 1 will appear directly.
1
- Show Feature – def show(file): this feature allows the user to show his saved account details based on the reading of his .txt file. After doing this step, the program will sleep for few seconds and it will redirect you to the menu again.
- ChangePIN Feature – def changePinFun(currentPIN, cardNumber, file): this feature allows the user to change his PIN number in the .txt file. Here, you are required to replace the saved PIN by new PIN and it should have same structure. After doing this step, the program will sleep for few seconds and it will redirect you to the menu again.
- Withdraw Feature – def withdrawFun(money, cardNumber, file): this feature allows the user to withdraw money from his account and then updates the account details in the .txt file accordingly. Remind that, if you don’t have enough money in your account, it will give a notification message and it will ask for new amount until you entered an amount that you can withdraw it. After doing this step, the program will sleep for few seconds and it will redirect you to the menu again.
- Deposit Feature – def depositFun(file, nMoney, cardNumber): this feature allows the user to deposit money to your account and then updates the account details in the .txt file accordingly. After doing this step, the program will sleep for few seconds and it will redirect you to the menu again.
- Bills Feature – def payBillFun(file, nMoney, cardNumber): this feature allows the user to pay a bill from his account, deducts this bill from the account and updates the account details in the .txt file accordingly.
- Enter the name of the bill.
- Enter the account number of this bill.
- Enter the value of this bill.
Remind that, if you don’t have enough money in your account, it will give a notification message and it will ask to deposit amount to deduct the bill. After doing this step, the program will sleep for few seconds and it will redirect you to the menu again.
- View Transactions Feature – def viewTransactionsFun(cardNumber): this feature allows the user to show the history of his account based on the .txt file. If there are transactions in the account, it will be presented along with its (date and time). Otherwise, it will show "no transactions". After doing this step, the program will sleep for few seconds and it will redirect you to the menu again.
- Terminate Feature – def terminateFun(file, nMoney, cardNumber): this feature allows the user to ter-minate the program and at same time it will show the last transactions on the account during the session.
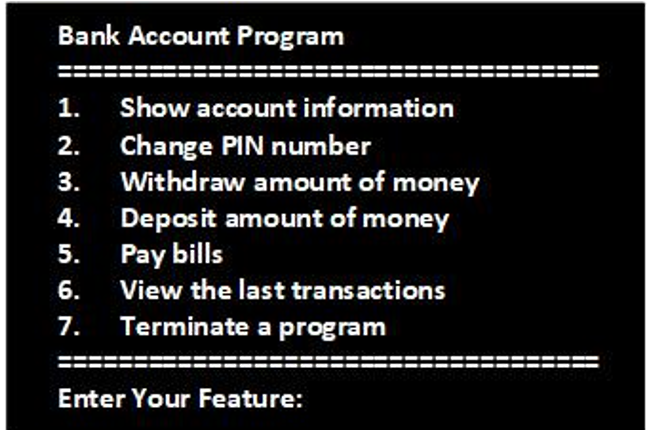

Step by step
Solved in 5 steps with 3 images

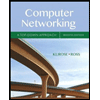
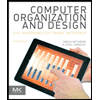
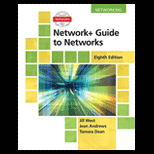
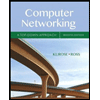
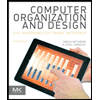
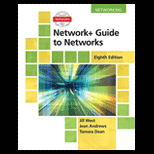
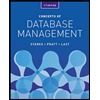
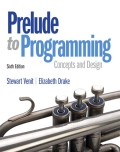
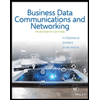