Design a class named Cylinder to represent cylinders. The class contains: ➤Two private double data fields named radius and length that specify the radius and length of a cylinder. The default values are 1.0 for radius and length. ➤ An int static data field named number OfObjects. The default value is 0. ➤ A no-arg constructor that creates a default cylinder. ▸ A constructor that creates a cylinder with the specified radius and length. ► Two sets of get and set methods for the two private data fields. ➤ A method named getArea() that returns the base area of the cylinder. ➤ A method named getVolume() that returns the volume of the cylinder. ➤ The static getNumberOfObjects method to return the data field number OfObjects. Requirements: 1. Draw the UML diagram for the class. Implement this class. The data fields should be private, and constructors and all other methods should be public. 2. Write a test program that should do the following tasks: ▸ create the first cylinder object using the no-arg constructor; ➤ create a second cylinder using the constructor with arguments to set radius to 2.5 and length to 12.3; ➤ create a third cylinder using the no-arg constructor; ➤ change the third cylinder's radius to 5.0 and length to 6.5. ➤ print out the radius and length of the first cylinder; ➤ print out the area of the second cylinder; print out the volume of the third cylinder. print out the value of the numOfObjects data field;
I already have the programming part done for this problem, just need help with making UML diagram. So please help create UML diagram for this problem.
I am pasting my program code underneath so you can copy paste and run it, so you don't need to create a program and attatching the instruction manual in the images so you know what is expected of the UML diagram.
1. Programm code for Cylinder class:
package homework;
public class Cylinder {
//Declare date fields
private double radius, length;
//Static date field
static int ObjectsCreated = 0;
//Constructor to create cylinder with default value of 1.0 for dimensions
public Cylinder() {
double radius;
double length;
this.radius = 1.0;
this.length = 1.0;
ObjectsCreated++;
}
//Constructor to create cylinder with specified radius and length
public Cylinder(double radius, double length) {
this.radius = radius;
this.length = length;
ObjectsCreated++;
}
//Functions to set values of cylinder's radius and length
public double getRadius(){
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
public double getLength() {
return length;
}
public void setLength(double length) {
this.length = length;
}
//Computes area of cylinder
public double getArea() {
return Math.PI * radius * radius; //Area of cylinder
}
//Computes volume of cylinder
public double getVolume() {
return Math.PI * radius * radius * length; //Volume of cylinder
}
//Insert method to display amount of objects created
public static int AmountOfObjectsCreated() {
return ObjectsCreated;
}
}
2. Programm code for CylinderTest class:
package homework;
public class CylinderTest {
public static void main(String[] args) {
//Create cylinder using no argument constructors
Cylinder C1 = new Cylinder();
Cylinder C2 = new Cylinder(2.5,12.3);
Cylinder C3 = new Cylinder();
C3.setRadius(5.0);
C3.setLength(6.5);
//Display results of all cylinder
System.out.println("The radius of the first cylinder is: " + C1.getRadius() + " and the length is: " + C1.getLength());
System.out.println("The base area of the second cylinder is: " + C2.getArea());
System.out.println("The volume of the third cylinder is: " + C3.getVolume());
//Display number of objects made
System.out.println("The amount of objects created is: " + Cylinder.AmountOfObjectsCreated());
}
}
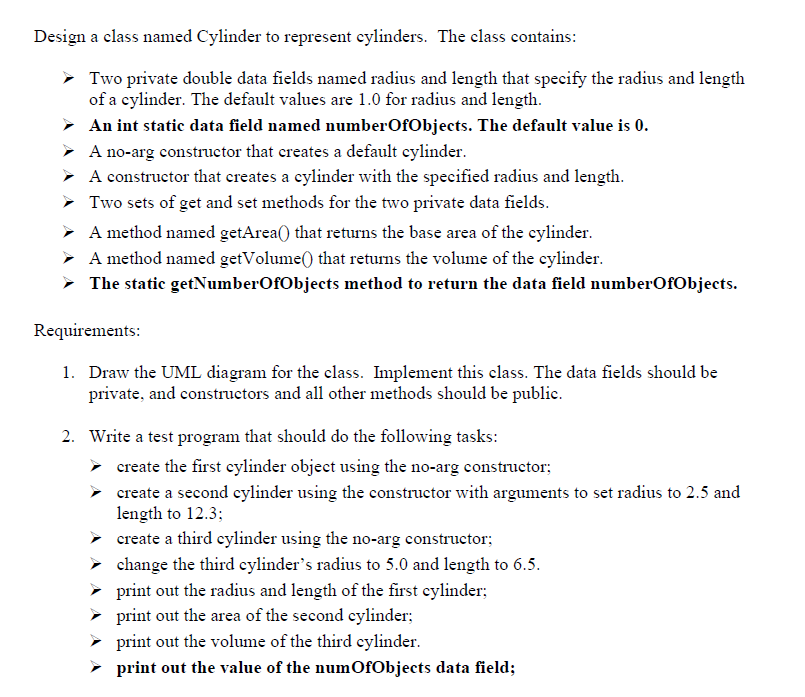

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

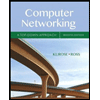
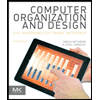
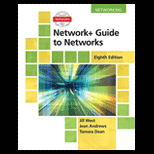
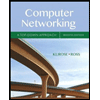
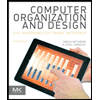
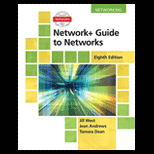
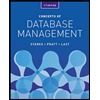
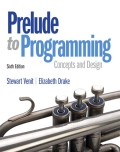
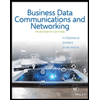