Design a class named Month. The class should have the following private members: • name - A string object that holds the name of a month, such as "January", "February", etc. • monthNumber - An integer variable that holds the number of the month. For example, January would be 1, February would be 2, etc. Valid values for this variable are 1 through 12. In addition, provide the following member functions: • A default constructor that sets monthNumber to 1 and name to "January." • A constructor that accepts the name of the month as an argument. It should set name to the value passed as the argument and set monthNumber to the correct value. • A constructor that accepts the number of the month as an argument. It should set monthNumber to the value passed as the argument and set name to the correct month name. • Appropriate set and get functions for the name and monthNumber member variables. • Prefix and postfix overloaded ++ operator functions that increment monthNumber and set name to the name of next month. If monthNumber is set to 12 when these functions execute, they should set monthNumber to 1 and name to "January". • Prefix and postfix overloaded -- operator functions that decrement monthNumber and set name to the name of previous month. If monthNumber is set to 1 when these functions execute, they should set monthNumber to 12 and name to "December". • Make operator overloading for asigning (=) an object to another. • Overload the comparetor operators ==, > and <. Also, you should overload cout's << operator and cin's >> operator to work with the Month class. Demonstrate the class in a program (main.cpp).
Design a class named Month. The class should have the following private members:
• name - A string object that holds the name of a month, such as "January", "February", etc.
• monthNumber - An integer variable that holds the number of the month. For example, January would be 1, February would be 2, etc. Valid values for this variable are 1 through 12.
In addition, provide the following member functions:
• A default constructor that sets monthNumber to 1 and name to "January."
• A constructor that accepts the name of the month as an argument. It should set name to the value passed as the argument and set monthNumber to the correct value.
• A constructor that accepts the number of the month as an argument. It should set monthNumber to the value passed as the argument and set name to the correct month name.
• Appropriate set and get functions for the name and monthNumber member variables.
• Prefix and postfix overloaded ++ operator functions that increment monthNumber and set name to the name of next month. If monthNumber is set to 12 when these functions execute, they should set monthNumber to 1 and name to "January".
• Prefix and postfix overloaded -- operator functions that decrement monthNumber and set name to the name of previous month. If monthNumber is set to 1 when these functions execute, they should set monthNumber to 12 and name to "December".
• Make operator overloading for asigning (=) an object to another.
• Overload the comparetor operators ==, > and <.
Also, you should overload cout's << operator and cin's >> operator to work with the Month class. Demonstrate the class in a program (main.cpp).

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 4 images

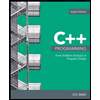
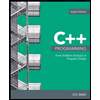