Dice game Rules of the game: The players roll three dice, and the program adds the sides that turn up. After the first roll of the three dice, a player may choose to roll the dice as many times as they wish until the player gets at least one side 2 from a dice. When a player gets at least one side 2 from the dice, that player's score drops to zero, and the turn switches to the other player. Both players play the game for an equal number of turns, and the player who gets a score higher than 18 wins. If both players get a score higher than 18 within an equal number of turns, the player with the higher score wins. If both of the players got the same scores, print the scores of the players. These are the minimum required functions for this program. Design and implement these functions. You can create more functions if you choose to. Here is the provided starter code: # A program to play a Dice game. import random def roll_die(): ''' Simulate a die roll ''' def player_turn(player_name, other_player, player_score): ''' Implements what happens on player's turn. Returns player's score, which represents the player's total score. ''' option = 'r' print(f'\n*******{player_name}\'s turn********\n') # < Insert the rest of your code here. > return player_score def main(): ''' The main driver of the program. Call the player_turn() functions here. ''' # < Insert the rest of your code here. > if __name__ == '__main__': main() Notes Study the example output below to understand the behavior of the program. To keep the game from running too fast, when one player gets a side 2, that player must press the enter key to switch the turn to the other player. Both players will play the game at least for one turn and an equal number of turns. Example 1 When prompted, the user enters “p” or “r” to determine whether to pass or roll. When one of the players gets one or more side 2 from rolling the die, the player’s score drops to zero, and the player presses the enter key to switch players. The players’ names go on the first and second lines. The first roll for Julie is a 6, 1, and 3 for a total score of 10. Julie rolls the die again. 1, 4, and 4 are rolled for a total score of 19. Since Julie scored higher than 18, the turn is switched to the second player. Jack got 4, 4, and 6 within the first roll for a total score of 14. He chooses to roll again and gets a score of 24. Since Jack’s total is greater than 18 and Julie’s total, the game is over, and Jack wins. Enter the first player name: Julie Enter the second player name: Jack *******Julie's turn******** Scores: 6, 1, and 3. Julie's score: 10 (p)ass or (r)oll? r Scores: 1, 4, and 4. Julie's score: 19 *******Jack's turn******** Scores: 4, 4, and 6. Jack's score: 14 (p)ass or (r)oll? r Scores: 1, 3, and 6. Jack's score: 24 Jack wins with a score of 24 Example 2 Jill rolls the dice and passes the turn to Julie. Julie rolled a 2, so her score remains zero, and the turn is passed to Jill. Jill also got at least one 2, so her score drops to zero, and the turn is passed to Julie. Julie got a score of 15 and passes the turn to Jill. Jill got a 2 again, and her score remains zero. The turn passes to Julie. Julie scores 21, and wins the game. Note that the game starts with Jill’s turn and finishes with Julie’s turn. Therefore, they had equal turns. Enter the first player name: Jill Enter the second player name: Julie *******Jill's turn******** Scores: 1, 3, and 4. Jill's score: 8 (p)ass or (r)oll? p *******Julie's turn******** Scores: 2, 5, and 1. Julie got at least one 2. Julie's score: 0 Press to continue ... *******Jill's turn******** Scores: 2, 2, and 3. Jill got at least one 2. Jill's score: 0 Press to continue ... *******Julie's turn******** Scores: 6, 3, and 6. Julie's score: 15 (p)ass or (r)oll? p *******Jill's turn******** Scores: 5, 5, and 2. Jill got at least one 2. Jill's score: 0 Press to continue ... *******Julie's turn******** Scores: 4, 1, and 1. Julie's score: 21 Julie wins with a score of 21 Example 6 In this example, Julie and Jack got the same score. So, the players’ scores are printed. Enter the first player name: Julie Enter the second player name: Jack *******Julie's turn******** Scores: 6, 4, and 6. Julie's score: 16 (p)ass or (r)oll? r Scores: 1, 1, and 5. Julie's score: 23 *******Jack's turn******** Scores: 5, 4, and 6. Jack's score: 15 (p)ass or (r)oll? r Scores: 4, 1, and 3. Jack's score: 23 Both players got
Dice game Rules of the game: The players roll three dice, and the program adds the sides that turn up. After the first roll of the three dice, a player may choose to roll the dice as many times as they wish until the player gets at least one side 2 from a dice. When a player gets at least one side 2 from the dice, that player's score drops to zero, and the turn switches to the other player. Both players play the game for an equal number of turns, and the player who gets a score higher than 18 wins. If both players get a score higher than 18 within an equal number of turns, the player with the higher score wins. If both of the players got the same scores, print the scores of the players. These are the minimum required functions for this program. Design and implement these functions. You can create more functions if you choose to. Here is the provided starter code: # A program to play a Dice game. import random def roll_die(): ''' Simulate a die roll ''' def player_turn(player_name, other_player, player_score): ''' Implements what happens on player's turn. Returns player's score, which represents the player's total score. ''' option = 'r' print(f'\n*******{player_name}\'s turn********\n') # < Insert the rest of your code here. > return player_score def main(): ''' The main driver of the program. Call the player_turn() functions here. ''' # < Insert the rest of your code here. > if __name__ == '__main__': main() Notes Study the example output below to understand the behavior of the program. To keep the game from running too fast, when one player gets a side 2, that player must press the enter key to switch the turn to the other player. Both players will play the game at least for one turn and an equal number of turns. Example 1 When prompted, the user enters “p” or “r” to determine whether to pass or roll. When one of the players gets one or more side 2 from rolling the die, the player’s score drops to zero, and the player presses the enter key to switch players. The players’ names go on the first and second lines. The first roll for Julie is a 6, 1, and 3 for a total score of 10. Julie rolls the die again. 1, 4, and 4 are rolled for a total score of 19. Since Julie scored higher than 18, the turn is switched to the second player. Jack got 4, 4, and 6 within the first roll for a total score of 14. He chooses to roll again and gets a score of 24. Since Jack’s total is greater than 18 and Julie’s total, the game is over, and Jack wins. Enter the first player name: Julie Enter the second player name: Jack *******Julie's turn******** Scores: 6, 1, and 3. Julie's score: 10 (p)ass or (r)oll? r Scores: 1, 4, and 4. Julie's score: 19 *******Jack's turn******** Scores: 4, 4, and 6. Jack's score: 14 (p)ass or (r)oll? r Scores: 1, 3, and 6. Jack's score: 24 Jack wins with a score of 24 Example 2 Jill rolls the dice and passes the turn to Julie. Julie rolled a 2, so her score remains zero, and the turn is passed to Jill. Jill also got at least one 2, so her score drops to zero, and the turn is passed to Julie. Julie got a score of 15 and passes the turn to Jill. Jill got a 2 again, and her score remains zero. The turn passes to Julie. Julie scores 21, and wins the game. Note that the game starts with Jill’s turn and finishes with Julie’s turn. Therefore, they had equal turns. Enter the first player name: Jill Enter the second player name: Julie *******Jill's turn******** Scores: 1, 3, and 4. Jill's score: 8 (p)ass or (r)oll? p *******Julie's turn******** Scores: 2, 5, and 1. Julie got at least one 2. Julie's score: 0 Press to continue ... *******Jill's turn******** Scores: 2, 2, and 3. Jill got at least one 2. Jill's score: 0 Press to continue ... *******Julie's turn******** Scores: 6, 3, and 6. Julie's score: 15 (p)ass or (r)oll? p *******Jill's turn******** Scores: 5, 5, and 2. Jill got at least one 2. Jill's score: 0 Press to continue ... *******Julie's turn******** Scores: 4, 1, and 1. Julie's score: 21 Julie wins with a score of 21 Example 6 In this example, Julie and Jack got the same score. So, the players’ scores are printed. Enter the first player name: Julie Enter the second player name: Jack *******Julie's turn******** Scores: 6, 4, and 6. Julie's score: 16 (p)ass or (r)oll? r Scores: 1, 1, and 5. Julie's score: 23 *******Jack's turn******** Scores: 5, 4, and 6. Jack's score: 15 (p)ass or (r)oll? r Scores: 4, 1, and 3. Jack's score: 23 Both players got
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Dice game
Rules of the game:
The players roll three dice, and the program adds the sides that turn up.
After the first roll of the three dice, a player may choose to roll the dice as many times as they wish until the player gets at least one side 2 from a dice.
When a player gets at least one side 2 from the dice, that player's score drops to zero, and the turn switches to the other player.
Both players play the game for an equal number of turns, and the player who gets a score higher than 18 wins.
If both players get a score higher than 18 within an equal number of turns, the player with the higher score wins.
If both of the players got the same scores, print the scores of the players.
These are the minimum required functions for this program. Design and implement these functions. You can create more functions if you choose to.
Here is the provided starter code:
# A program to play a Dice game.
import random
def roll_die():
''' Simulate a die roll '''
def player_turn(player_name, other_player, player_score):
'''
Implements what happens on player's turn.
Returns player's score, which represents
the player's total score.
'''
option = 'r'
print(f'\n*******{player_name}\'s turn********\n')
# < Insert the rest of your code here. >
return player_score
def main():
'''
The main driver of the program. Call
the player_turn() functions here.
'''
# < Insert the rest of your code here. >
if __name__ == '__main__':
main()
Notes
Study the example output below to understand the behavior of the program. To keep the game from running too fast, when one player gets a side 2, that player must press the enter key to switch the turn to the other player.
Both players will play the game at least for one turn and an equal number of turns.
Example 1
When prompted, the user enters “p” or “r” to determine whether to pass or roll. When one of the players gets one or more side 2 from rolling the die, the player’s score drops to zero, and the player presses the enter key to switch players.
The players’ names go on the first and second lines. The first roll for Julie is a 6, 1, and 3 for a total score of 10. Julie rolls the die again. 1, 4, and 4 are rolled for a total score of 19. Since Julie scored higher than 18, the turn is switched to the second player. Jack got 4, 4, and 6 within the first roll for a total score of 14. He chooses to roll again and gets a score of 24. Since Jack’s total is greater than 18 and Julie’s total, the game is over, and Jack wins.
Enter the first player name: Julie
Enter the second player name: Jack
*******Julie's turn********
Scores: 6, 1, and 3.
Julie's score: 10
(p)ass or (r)oll? r
Scores: 1, 4, and 4.
Julie's score: 19
*******Jack's turn********
Scores: 4, 4, and 6.
Jack's score: 14
(p)ass or (r)oll? r
Scores: 1, 3, and 6.
Jack's score: 24
Jack wins with a score of 24
Example 2
Jill rolls the dice and passes the turn to Julie. Julie rolled a 2, so her score remains zero, and the turn is passed to Jill. Jill also got at least one 2, so her score drops to zero, and the turn is passed to Julie. Julie got a score of 15 and passes the turn to Jill. Jill got a 2 again, and her score remains zero. The turn passes to Julie. Julie scores 21, and wins the game. Note that the game starts with Jill’s turn and finishes with Julie’s turn. Therefore, they had equal turns.
Enter the first player name: Jill
Enter the second player name: Julie
*******Jill's turn********
Scores: 1, 3, and 4.
Jill's score: 8
(p)ass or (r)oll? p
*******Julie's turn********
Scores: 2, 5, and 1.
Julie got at least one 2.
Julie's score: 0
Press to continue ...
*******Jill's turn********
Scores: 2, 2, and 3.
Jill got at least one 2.
Jill's score: 0
Press to continue ...
*******Julie's turn********
Scores: 6, 3, and 6.
Julie's score: 15
(p)ass or (r)oll? p
*******Jill's turn********
Scores: 5, 5, and 2.
Jill got at least one 2.
Jill's score: 0
Press to continue ...
*******Julie's turn********
Scores: 4, 1, and 1.
Julie's score: 21
Julie wins with a score of 21
Example 6
In this example, Julie and Jack got the same score. So, the players’ scores are printed.
Enter the first player name: Julie
Enter the second player name: Jack
*******Julie's turn********
Scores: 6, 4, and 6.
Julie's score: 16
(p)ass or (r)oll? r
Scores: 1, 1, and 5.
Julie's score: 23
*******Jack's turn********
Scores: 5, 4, and 6.
Jack's score: 15
(p)ass or (r)oll? r
Scores: 4, 1, and 3.
Jack's score: 23
Both players got the same score
Julie: 23 scores
Jack: 23 scores
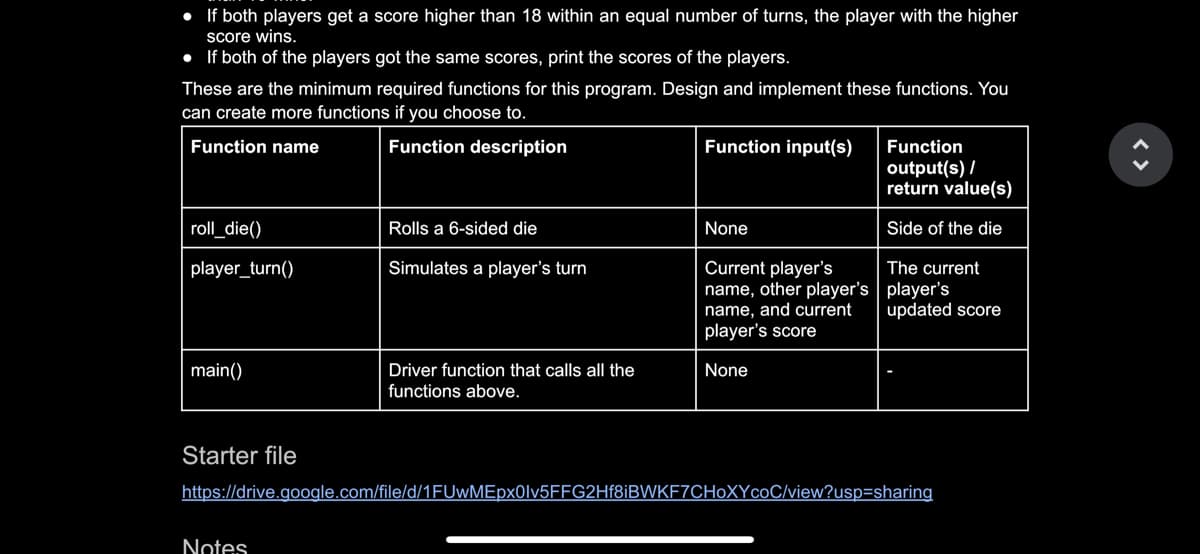
Transcribed Image Text:• If both players get a score higher than 18 within an equal number of turns, the player with the higher
score wins.
• If both of the players got the same scores, print the scores of the players.
These are the minimum required functions for this program. Design and implement these functions. You
can create more functions if you choose to.
Function name
Function description
roll_die()
player_turn()
main()
Starter file
Rolls a 6-sided die
Simulates a player's turn
Notes
Driver function that calls all the
functions above.
Function input(s)
None
Current player's
name, other player's
name, and current
player's score
None
Function
output(s)/
return value(s)
Side of the die
The current
player's
updated score
https://drive.google.com/file/d/1FUwMEpx0lv5FFG2Hf8iBWKF7CHOXYcoC/view?usp=sharing
<>
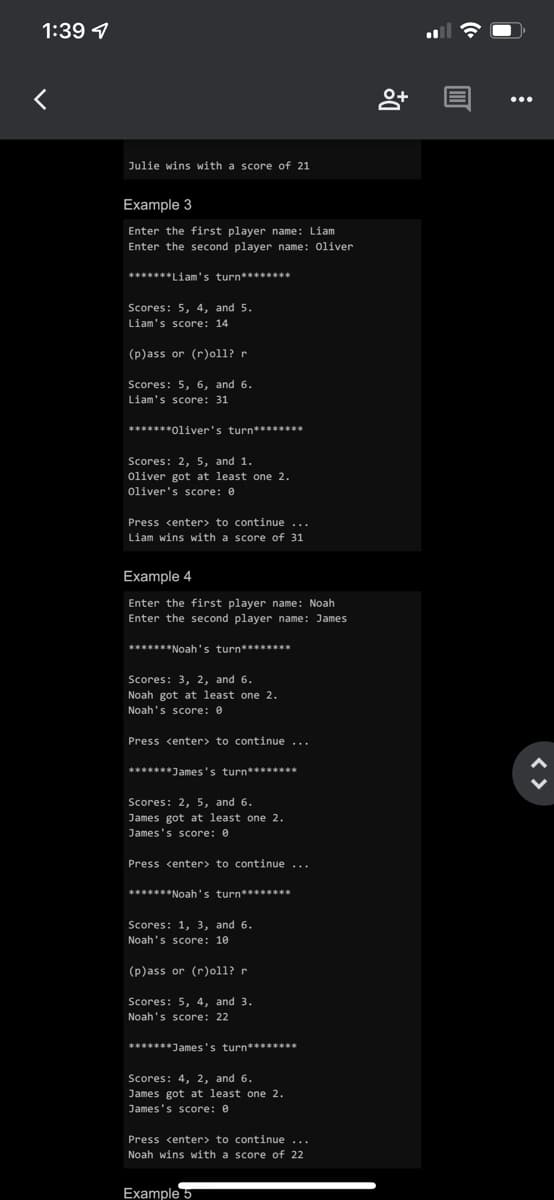
Transcribed Image Text:1:39
<
Julie wins with a score of 21
Example 3
Enter the first player name: Liam
Enter the second player name: Oliver
*******Liam's turn********
Scores: 5, 4, and 5.
Liam's score: 14.
(p)ass or (r)oll? r
Scores: 5, 6, and 6.
Liam's score: 31
*******Oliver's turn********
Scores: 2, 5, and 1.
Oliver got at least one 2.
Oliver's score: 0
Press <enter> to continue...
Liam wins with a score of 31
Example 4
Enter the first player name: Noah
Enter the second player name: James
*******Noah's turn********
Scores: 3, 2, and 6.
Noah got at least one 2.
Noah's score: 0
Press <enter> to continue ...
*******James's turn********
Scores: 2, 5, and 6.
James got at least one 2.
James's score: 0
Press <enter> to continue ...
*******Noah's turn********
Scores: 1, 3, and 6.
Noah's score: 10
(p)ass or (r)oll? r
Scores: 5, 4, and 3.
Noah's score: 22
*******James's turn********
Scores: 4, 2, and 6.
James got at least one 2.
James's score: 0
Press <enter> to continue...
Noah wins with a score of 22
Example 5
8+
@
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
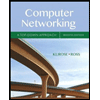
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
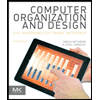
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
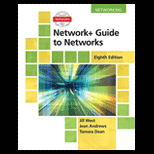
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
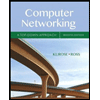
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
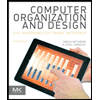
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
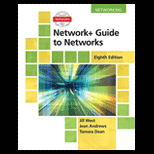
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
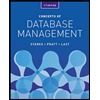
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
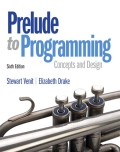
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
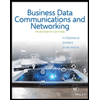
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY