do this in C++ please read carefully and follow every instructions please do not copy or plagarise. thanks!
do this in C++ please read carefully and follow every instructions please do not copy or plagarise. thanks!
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter7: File Handling And Applications
Section: Chapter Questions
Problem 1GZ
Related questions
Question
do this in C++ please read carefully and follow every instructions
please do not copy or plagarise.
thanks!
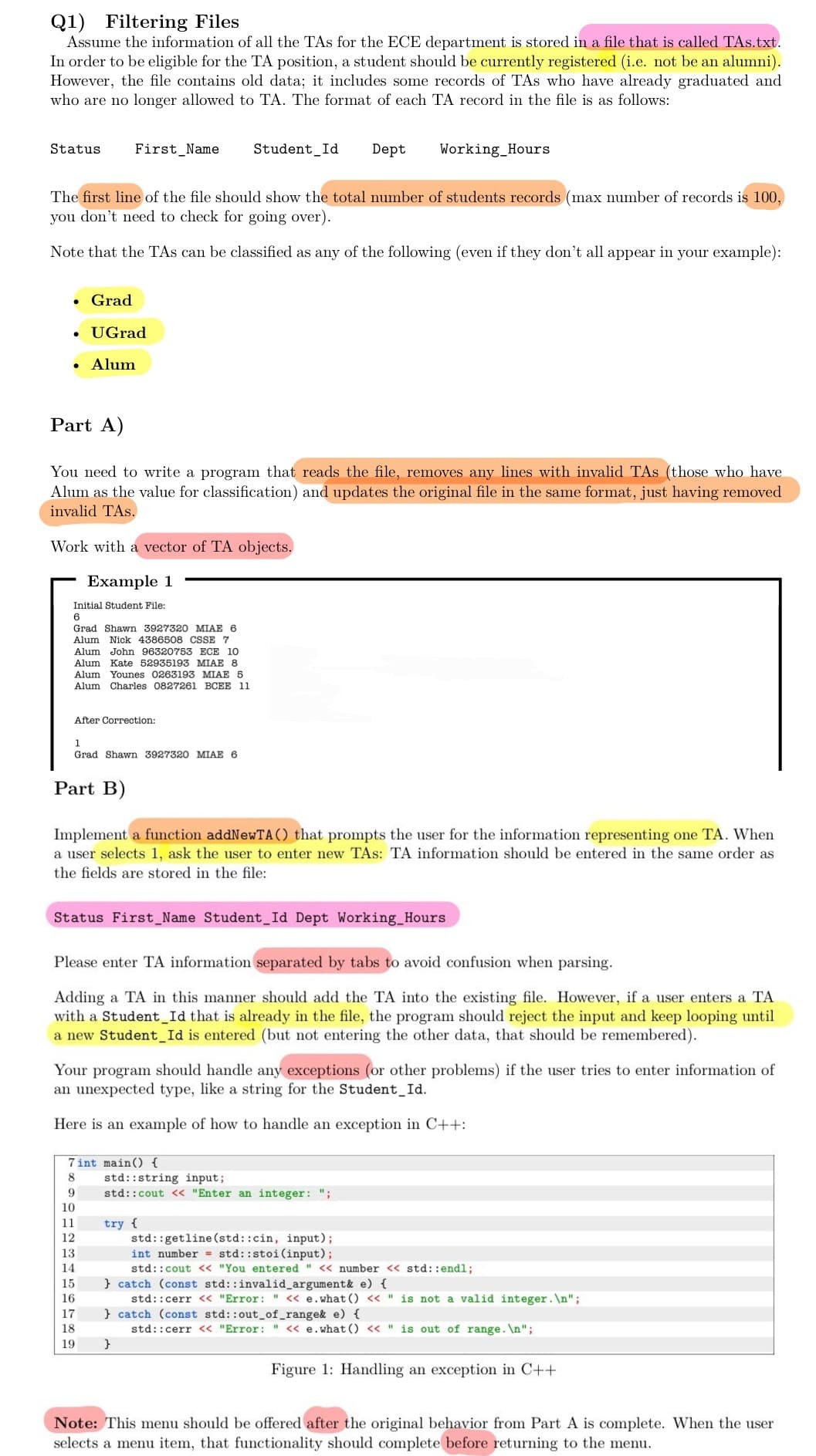
Transcribed Image Text:Q1) Filtering Files
Assume the information of all the TAs for the ECE department is stored in a file that is called TAs.txt.
In order to be eligible for the TA position, a student should be currently registered (i.e. not be an alumni).
However, the file contains old data; it includes some records of TAs who have already graduated and
who are no longer allowed to TA. The format of each TA record in the file is as follows:
Status
First Name
The first line of the file should show the total number of students records (max number of records is 100,
you don't need to check for going over).
Note that the TAs can be classified as any of the following (even if they don't all appear in your example):
• Grad
. UGrad
Alum
Part A)
You need to write a program that reads the file, removes any lines with invalid TAs (those who have
Alum as the value for classification) and updates the original file in the same format, just having removed
invalid TAS.
Work with a vector of TA objects.
Example 1
Initial Student File:
6
Grad Shawn 3927320 MIAE 6
Alum Nick 4386508 CSSE 7
Alum John 96320753 ECE 10
Alum Kate 52935193 MIAE 8
Alum You
3193 MIAE 5
Alum Charles 0827261 BCEE 11
After Correction:
1
Grad Shawn 3927320 MIAE 6
Part B)
Student Id Dept Working Hours
Implement a function addNewTA () that prompts the user for the information representing one TA. When
a user selects 1, ask the user to enter new TAS: TA information should be entered in the same order as
the fields are stored in the file:
Status First_Name Student Id Dept Working Hours
Please enter TA information separated by tabs to avoid confusion when parsing.
Adding a TA in this manner should add the TA into the existing file. However, if a user enters a TA
with a Student Id that is already in the file, the program should reject the input and keep looping until
a new Student Id is entered (but not entering the other data, that should be remembered).
Your program should handle any exceptions (or other problems) if the user tries to enter information of
an unexpected type, like a string for the Student Id.
Here is an example of how to handle an exception in C++:
7 int main() {
8 std::string input;
9
10
11
12
13
14
15
16
17
18
19 }
std::cout << "Enter an integer: ";
try {
std::getline (std::cin, input);
int number
std::stoi (input);
std::cout << "You entered " << number << std::endl;
} catch (const std::invalid_argument& e) {
std::cerr << "Error: " << e. what () << " is not a valid integer.\n";
} catch (const std::out_of_range& e) {
std::cerr << "Error: " << e. what () << " is out of range.\n";
Figure 1: Handling an exception in C++
Note: This menu should be offered after the original behavior from Part A is complete. When the user
selects a menu item, that functionality should complete before returning to the menu.
![Part C)
Extend your TA record management system to offer a new option. If the user enters a 2, they should be
prompted to select from a list of columns to order by, and then choose to order in ascending or descending
order. The new ordering should be written out to the file in the same format as before.
To accomplish this, use the TA class you implemented in the previous part of the assignment, and store
your TA instances in a vector. Then, use the sort function from the C++ algorithm library to perform
the sorting. Make sure your compiler is set to use at least C++11 so that you can use lambdas in your
sort function.
When sorting the TAs, be careful to ensure that strings are ordered as strings and numbers are ordered
as numbers (i.e. 10 should not end up before 2). Consult the sort function documentation for guidance
on how to accomplish this.
Here is an example of how to use the sort function with a lambda to sort a vector of integers in
descending order:
21 std::vector<int> vec{5, 2, 8, 1, 6};
22
23
24
std::sort (vec.begin(), vec.end(), [] (int a, int b) {
return a > b;
});
Figure 2: Using sort function with a lambda to sort a vector of integers in descending order](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0ec4d20d-c503-4b4a-9b34-ac5c69c93506%2Fcffc6884-f17f-440d-8f8a-04598cbd6822%2Fcvoapi_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Part C)
Extend your TA record management system to offer a new option. If the user enters a 2, they should be
prompted to select from a list of columns to order by, and then choose to order in ascending or descending
order. The new ordering should be written out to the file in the same format as before.
To accomplish this, use the TA class you implemented in the previous part of the assignment, and store
your TA instances in a vector. Then, use the sort function from the C++ algorithm library to perform
the sorting. Make sure your compiler is set to use at least C++11 so that you can use lambdas in your
sort function.
When sorting the TAs, be careful to ensure that strings are ordered as strings and numbers are ordered
as numbers (i.e. 10 should not end up before 2). Consult the sort function documentation for guidance
on how to accomplish this.
Here is an example of how to use the sort function with a lambda to sort a vector of integers in
descending order:
21 std::vector<int> vec{5, 2, 8, 1, 6};
22
23
24
std::sort (vec.begin(), vec.end(), [] (int a, int b) {
return a > b;
});
Figure 2: Using sort function with a lambda to sort a vector of integers in descending order
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
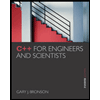
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
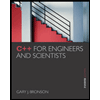
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr