Driving is expensive. Write a program with a car's miles/gallon and gas dollars/gallon (both floats) as input, and output the gas cost for 10 miles, 50 miles, and 400 miles. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: print ('{:.2f}'.format (your_value)) Ex: If the input to your program is: 20.0 3.1599
Driving is expensive. Write a program with a car's miles/gallon and gas dollars/gallon (both floats) as input, and output the gas cost for 10 miles, 50 miles, and 400 miles. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: print ('{:.2f}'.format (your_value)) Ex: If the input to your program is: 20.0 3.1599
Chapter4: Making Decisions
Section: Chapter Questions
Problem 7E: Write a program named GuessingGame that generates a random number between 1 and 10. (In other words,...
Related questions
Question
Python
- Create a function to match the specifications
- Use floating-point value division
- Write a program to get the user input and call the custom function and produce the desired output using a format function and __name__ == '__main__' to output the specified precision
my code doesn't work for this input = 50 20.0 3.1599 that the function returns: 7.89975
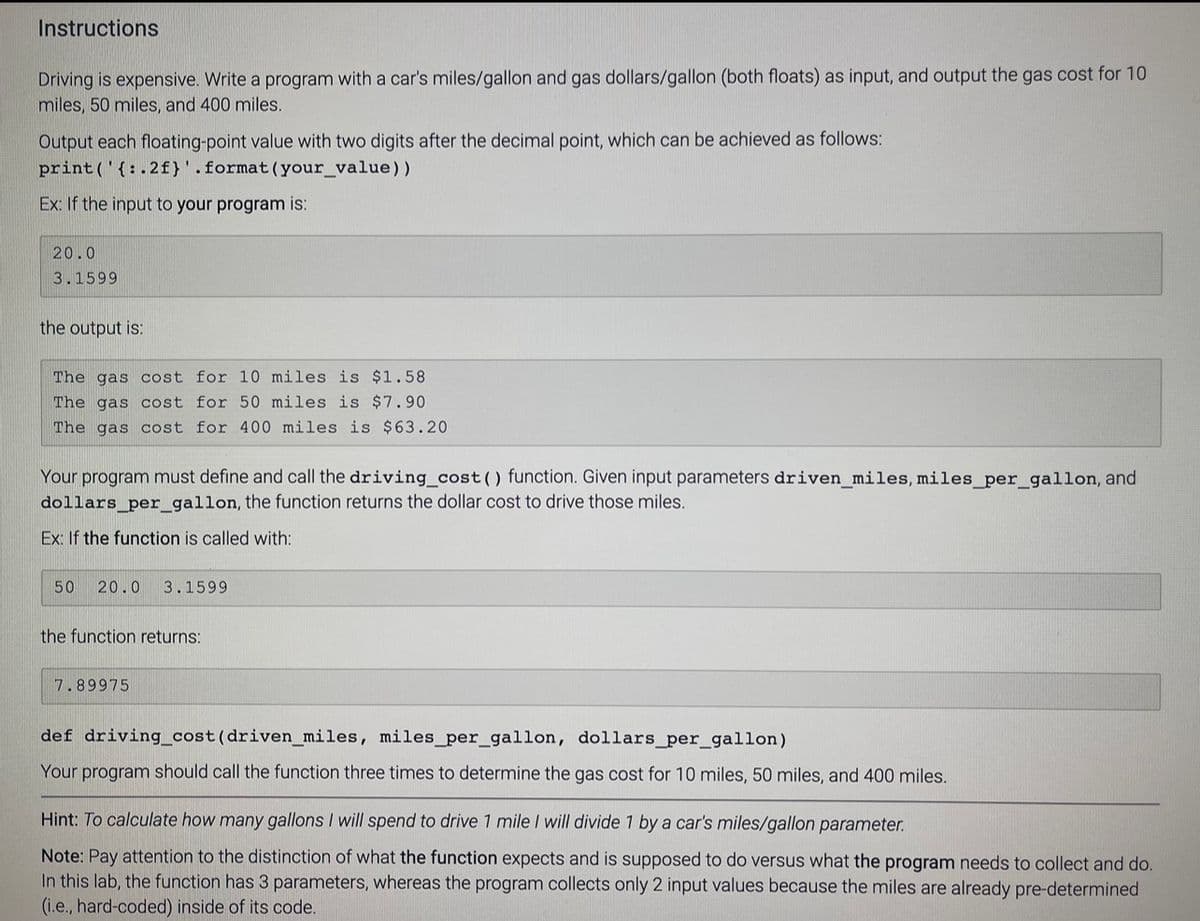
Transcribed Image Text:Instructions
Driving is expensive. Write a program with a car's miles/gallon and gas dollars/gallon (both floats) as input, and output the gas cost for 10
miles, 50 miles, and 400 miles.
Output each floating-point value with two digits after the decimal point, which can be achieved as follows:
print ('{:.2f}'.format(your_value))
Ex: If the input to your program is:
20.0
3.1599
the output is:
The gas cost for 10 miles is $1.58
The gas cost for 50 miles is $7.90
The gas cost for 400 miles is $63.20
Your program must define and call the driving_cost() function. Given input parameters driven_miles, miles_per_gallon, and
dollars per_gallon, the function returns the dollar cost to drive those miles.
Ex: If the function is called with:
50
20.0
3.1599
the function returns:
7.89975
def driving_cost(driven_miles, miles_per_gallon, dollars_per_gallon)
Your program should call the function three times to determine the gas cost for 10 miles, 50 miles, and 400 miles.
Hint: To calculate how many gallons I will spend to drive 1 mile I will divide 1 by a car's miles/gallon parameter.
Note: Pay attention to the distinction of what the function expects and is supposed to do versus what the program needs to collect and do.
In this lab, the function has 3 parameters, whereas the program collects only 2 input values because the miles are already pre-determined
(i.e., hard-coded) inside of its code.
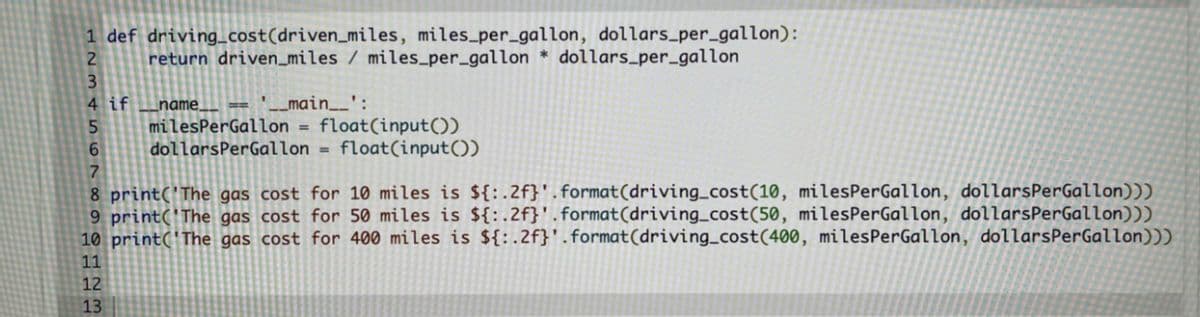
Transcribed Image Text:1 def driving_cost(driven_miles, miles_per_gallon, dollars_per_gallon):
return driven_miles / miles_per_gallon * dollars_per_gallon
-== '_main_':
milesPerGallon = float(input())
dollarsPerGallon = float(input())
4 if
Iname.
5.
8 print('The gas cost for 10 miles is ${:.2f}'.format(driving_cost(10, milesPerGallon, dollarsPerGallon)))
9 print('The gas cost for 50 miles is ${:.2f}'.format(driving_cost(50, milesPerGallon, dollarsPerGallon)))
10 print('The gas cost for 400 miles is ${:.2f}'.format(driving_cost(400, milesPerGallon, dollarsPerGallon)))
11
12
13
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
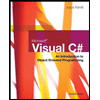
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
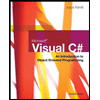
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,