This is a main module. w7_main Module (supplied) Do not modify this module! Look at the code and make sure you understand it. // Workshop 7 // Version: 1.0 // Author: Asam Gulaid // Description: // This file tests your workshop 7 ///////////////////////////////////////////// #include #include "Minister.h" #include "Minister.h" // intentional #include "MemberParliament.h" #include "MemberParliament.h" // intentional using namespace std; using namespace sdds; void printHeader(const char* title) { char oldFill = cout.fill('-'); cout.width(62); cout << "" << endl; cout << "|> " << title << endl; cout.fill('-'); cout.width(62); cout << "" << endl; cout.fill(oldFill); } void newElections(Minister& aMinister, const char* district, const char* newPM, double year) { aMinister.NewDistrict(district); aMinister.changePM(newPM); aMinister.assumeOffice(year); cout << "Status of New Election " << aMinister << endl << endl; } int main() { printHeader("Person 1: MemberParliament"); MemberParliament mp("MP9403", 59); cout << mp << endl << endl; mp.NewDistrict("Scarborough"); mp.NewDistrict("Mississauga"); mp.NewDistrict("Huron County"); cout << endl << mp << endl << endl; printHeader("Person 2: Read/Write"); cin >> mp; cout << endl << mp << endl << endl; printHeader("Person 3: Minister"); Minister aMinister("MP9573", 63, 2003, "Harper", "Pickering"); cout << endl; newElections(aMinister, "Whitby", "Trudeau", 2007); newElections(aMinister, "Kitchener", "Biden", 2010); newElections(aMinister, "Oakville", "Trump", 2015); printHeader("Person 4: Read/Write"); cin >> aMinister; cout << endl << (MemberParliament)aMinister; cout << endl << aMinister << endl << endl; return 0; }
This is a main module. w7_main Module (supplied) Do not modify this module! Look at the code and make sure you understand it. // Workshop 7 // Version: 1.0 // Author: Asam Gulaid // Description: // This file tests your workshop 7 ///////////////////////////////////////////// #include #include "Minister.h" #include "Minister.h" // intentional #include "MemberParliament.h" #include "MemberParliament.h" // intentional using namespace std; using namespace sdds; void printHeader(const char* title) { char oldFill = cout.fill('-'); cout.width(62); cout << "" << endl; cout << "|> " << title << endl; cout.fill('-'); cout.width(62); cout << "" << endl; cout.fill(oldFill); } void newElections(Minister& aMinister, const char* district, const char* newPM, double year) { aMinister.NewDistrict(district); aMinister.changePM(newPM); aMinister.assumeOffice(year); cout << "Status of New Election " << aMinister << endl << endl; } int main() { printHeader("Person 1: MemberParliament"); MemberParliament mp("MP9403", 59); cout << mp << endl << endl; mp.NewDistrict("Scarborough"); mp.NewDistrict("Mississauga"); mp.NewDistrict("Huron County"); cout << endl << mp << endl << endl; printHeader("Person 2: Read/Write"); cin >> mp; cout << endl << mp << endl << endl; printHeader("Person 3: Minister"); Minister aMinister("MP9573", 63, 2003, "Harper", "Pickering"); cout << endl; newElections(aMinister, "Whitby", "Trudeau", 2007); newElections(aMinister, "Kitchener", "Biden", 2010); newElections(aMinister, "Oakville", "Trump", 2015); printHeader("Person 4: Read/Write"); cin >> aMinister; cout << endl << (MemberParliament)aMinister; cout << endl << aMinister << endl << endl; return 0; }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
This is a main module.
w7_main Module (supplied)
Do not modify this module! Look at the code and make sure you understand it.
// Workshop 7 // Version: 1.0 // Author: Asam Gulaid // Description: // This file tests your workshop 7 ///////////////////////////////////////////// #include<iostream> #include "Minister.h" #include "Minister.h" // intentional #include "MemberParliament.h" #include "MemberParliament.h" // intentional using namespace std; using namespace sdds; void printHeader(const char* title) { char oldFill = cout.fill('-'); cout.width(62); cout << "" << endl; cout << "|> " << title << endl; cout.fill('-'); cout.width(62); cout << "" << endl; cout.fill(oldFill); } void newElections(Minister& aMinister, const char* district, const char* newPM, double year) { aMinister.NewDistrict(district); aMinister.changePM(newPM); aMinister.assumeOffice(year); cout << "Status of New Election " << aMinister << endl << endl; } int main() { printHeader("Person 1: MemberParliament"); MemberParliament mp("MP9403", 59); cout << mp << endl << endl; mp.NewDistrict("Scarborough"); mp.NewDistrict("Mississauga"); mp.NewDistrict("Huron County"); cout << endl << mp << endl << endl; printHeader("Person 2: Read/Write"); cin >> mp; cout << endl << mp << endl << endl; printHeader("Person 3: Minister"); Minister aMinister("MP9573", 63, 2003, "Harper", "Pickering"); cout << endl; newElections(aMinister, "Whitby", "Trudeau", 2007); newElections(aMinister, "Kitchener", "Biden", 2010); newElections(aMinister, "Oakville", "Trump", 2015); printHeader("Person 4: Read/Write"); cin >> aMinister; cout << endl << (MemberParliament)aMinister; cout << endl << aMinister << endl << endl; return 0; }
![lab (part 1) (100%)
MemberParliament Module
Design and code a class named Member Parliament that holds information about a member of parliament. Place your class definition in a
header file named Member Parliament.h and your function definitions in an implementation file named Member Parliament.cpp .
Include in your solution all of the statements necessary for your code to compile under a standard C++ compiler and within the sdds
namespace.
MemberParliament Class
Design and code a class named Member Parliament that holds information about a member of parliament.
MemberParliament Private Members
The class should be able to store the following data (Use appropriate names!):
• The parliament id number as a statically allocated array of characters of size 32.
• The district the MP represents as a statically allocated array of characters of size 64.
• The age of the MP.
You can add any other private members to the class, as required by your design.
MemberParliament Public Members
• A custom constructor that receives as parameters the MP Id and age. Set the district the MP represents as Unassigned. Assume all data is
valid.
void NewDistrict (const char* district) : assigns the MP to new district. Prints to the screen the message
●
[MP ID] [CURRENT_DISTRICT] ---> [NEW_DISTRICT] | <ENDL>
where
Helper Functions
• overload the insertion and extraction operators to insert a MemberParliament into a stream and extract a Member Parliament from a stream.
These operators should call the write / read member functions in the class MemberParliament.
o the mp id is a field of 8 characters aligned to the right
o current district is a field of 20 characters aligned to the right
o new district is a field of 23 characters aligned to left
ostream& write(ostream& os) : a query that inserts into os the content of the object in the format
Minister Module
| [MP ID] | [AGE] | [DISTRICT]
Design and code a class named Minister that holds information about a minister of the crown in the Canadian Cabinet. Place your class
definition in a header file named Minister.h and your function definitions in an implementation file named Minister.cpp.
istream& read(istream& in) : a mutator that reads from the stream in the data for the current object
Include in your solution all of the statements necessary for your code to compile under a standard C++ compiler and within the sdds
namespace.
●
MP Age: [USER TYPES HERE]
MP Id: [USER TYPES HERE]
District: [USER TYPES HERE]
Minister Class
Design and code a class named Minister that holds information about the a minister of the crown in the Canadian Cabinet. This class should
inherit from Member Parliament class.
Minister Private Members
The class should be able to store the following data (on top of data coming from the parent class):
• The name of the Prime Minister the Minister reports toas a statically allocated array of characters of size 50.
• The year the minister assumed office.
You can add any other private members in the class, as required by your design. Do not duplicate members from the base class!
Minister Public Members
●
a custom constructor that receives the following information about the Minister: idd, age, the year assumed office, district, and name of
the prime minister. Call the constructor from the base class and pass the relevant data to it. update the private data members of the
Minister accordingly
void changePM(const char* pm): a mutator that updates the PM the minister reports to.
void assumeOffice (double year) : a mutator that updates the year the minister assumed office
ostream& write(ostream& os): a query that inserts into os the content of the object in the format
}
| [ID] | [age] | [DISTRICT] | [PM]/[Year Assumed Office]
Helper Functions
• overload the insertion and extraction operators to insert a Minister into a stream and extract a Minister from a stream. These operators
should call the write / read member functions in the class Minister.
istream& read(istream& in) : a mutator that reads from the stream in the data for the current object
w7_main Module (supplied)
}
Age: [USER TYPES HERE]
Id: [USER TYPES HERE]
District: [USER TYPES HERE]
Do not modify this module! Look at the code and make sure you understand it.
Reports TO: [USER TYPES HERE]
Year Assumed Office: [USER TYPES HERE]
// Workshop 7
// Version: 1.0
}
// Author: Asam Gulaid
// Description:
// This file tests your workshop 7
#include<iostream>
#include "Minister.h"
#include "Minister.h" // intentional
#include "MemberParliament.h"
#include "MemberParliament.h" // intentional
using namespace std;
using namespace sdds;
void printHeader (const char* title)
{
char oldFill = cout.fill('-');
cout.width(62);
cout <<
cout << ">
int main()
{
void newElections (Minister& aMinister, const char* district, const char* newPM, double year)
{
|| ||
cout.fill('-');
cout.width(62);
cout << << endl;
cout.fill(oldFill);
|| ||
aMinister.NewDistrict(district);
aMinister.changePM(newPM);
aMinister.assumeOffice (year);
cout << "Status of New Election " << aMinister << endl << endl;
<< endl;
printHeader("Person 1: MemberParliament");
e.md
11
MemberParliament mp("MP9403", 59);
cout << mp << endl << endl;
mp. NewDistrict ("Scarborough");
mp. NewDistrict("Mississauga");
mp. NewDistrict ("Huron County");
cout << endl << mp << endl << endl;
printHeader("Person 2: Read/Write");
cin >> mp;
cout << endl << mp << endl << endl;
<< title << endl;
printHeader("Person 3: Minister");
Minister aMinister("MP9573", 63, 2003, "Harper", "Pickering");
cout << endl;
newElections (aMinister, "Whitby", "Trudeau", 2007);
newElections (aMinister, "Kitchener", "Biden", 2010);
newElections (aMinister, "Oakville", "Trump", 2015);
Sample Output
printHeader("Person 4: Read/Write");
cin >> aMinister;
cout << endl << (MemberParliament)aMinister;
cout << endl << aMinister << endl << endl;
return 0;
| MP9403| |
MP9403
| MP9403|
|> Person 1: MemberParliament
Age: 40
Id: MP9566
| MP9403 | 59 | Unassigned
| MP9403 | 59 | Huron County
|> Person 2: Read/Write
District: Milton
| MP9573| |
| MP9566 | 40 | Milton
> Person 3: Minister
Age: 66
Id: MP9701
| MP9573| |
Pickering ---> Whitby
Status of New Election | MP9573 | 63 | Whitby | Trudeau/2007
Unassigned ---> Scarborough
Scarborough ---> Mississauga
Mississauga ---> Huron County
| MP9573| |
Kitchener ---> Oakville
Status of New Election | MP9573 | 63 | Oakville | Trump/2015
| MP9573| |
Whitby ---> Kitchener
Status of New Election | MP9573 | 63 | Kitchener | Biden/2010
Data Entry
> Person 4: Read/Write
Files to submit:
Unassigned ---> Pickering
District: Sudbury
Reports
TO: Obama
Year Assumed Office: 2021
MP9566
Milton
66
MP9701
Sudbury
Obama
2021
| MP9701 | 66 | Sudbury
| MP9701 | 66 | Sudbury | Obama/2021
MemberParliament.cpp
MemberParliament.h
Minister.cpp
Minister.h
main.cpp
|
|
Custom code submission
If you have any additional custom code, (i.e. functions, classes etc) that you want to reuse in this workshop save them under a module called
Utils (Utils.cpp and Utils.h) and submit them with your workshop using the instructions in the "Submitting Utils Module" section.
I](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F33182b36-0ae9-4d27-a72b-3861bbb0e77d%2Fe2ba9469-3957-4e1f-97ae-93f44a60251b%2Fl4y4zrn5_processed.jpeg&w=3840&q=75)
Transcribed Image Text:lab (part 1) (100%)
MemberParliament Module
Design and code a class named Member Parliament that holds information about a member of parliament. Place your class definition in a
header file named Member Parliament.h and your function definitions in an implementation file named Member Parliament.cpp .
Include in your solution all of the statements necessary for your code to compile under a standard C++ compiler and within the sdds
namespace.
MemberParliament Class
Design and code a class named Member Parliament that holds information about a member of parliament.
MemberParliament Private Members
The class should be able to store the following data (Use appropriate names!):
• The parliament id number as a statically allocated array of characters of size 32.
• The district the MP represents as a statically allocated array of characters of size 64.
• The age of the MP.
You can add any other private members to the class, as required by your design.
MemberParliament Public Members
• A custom constructor that receives as parameters the MP Id and age. Set the district the MP represents as Unassigned. Assume all data is
valid.
void NewDistrict (const char* district) : assigns the MP to new district. Prints to the screen the message
●
[MP ID] [CURRENT_DISTRICT] ---> [NEW_DISTRICT] | <ENDL>
where
Helper Functions
• overload the insertion and extraction operators to insert a MemberParliament into a stream and extract a Member Parliament from a stream.
These operators should call the write / read member functions in the class MemberParliament.
o the mp id is a field of 8 characters aligned to the right
o current district is a field of 20 characters aligned to the right
o new district is a field of 23 characters aligned to left
ostream& write(ostream& os) : a query that inserts into os the content of the object in the format
Minister Module
| [MP ID] | [AGE] | [DISTRICT]
Design and code a class named Minister that holds information about a minister of the crown in the Canadian Cabinet. Place your class
definition in a header file named Minister.h and your function definitions in an implementation file named Minister.cpp.
istream& read(istream& in) : a mutator that reads from the stream in the data for the current object
Include in your solution all of the statements necessary for your code to compile under a standard C++ compiler and within the sdds
namespace.
●
MP Age: [USER TYPES HERE]
MP Id: [USER TYPES HERE]
District: [USER TYPES HERE]
Minister Class
Design and code a class named Minister that holds information about the a minister of the crown in the Canadian Cabinet. This class should
inherit from Member Parliament class.
Minister Private Members
The class should be able to store the following data (on top of data coming from the parent class):
• The name of the Prime Minister the Minister reports toas a statically allocated array of characters of size 50.
• The year the minister assumed office.
You can add any other private members in the class, as required by your design. Do not duplicate members from the base class!
Minister Public Members
●
a custom constructor that receives the following information about the Minister: idd, age, the year assumed office, district, and name of
the prime minister. Call the constructor from the base class and pass the relevant data to it. update the private data members of the
Minister accordingly
void changePM(const char* pm): a mutator that updates the PM the minister reports to.
void assumeOffice (double year) : a mutator that updates the year the minister assumed office
ostream& write(ostream& os): a query that inserts into os the content of the object in the format
}
| [ID] | [age] | [DISTRICT] | [PM]/[Year Assumed Office]
Helper Functions
• overload the insertion and extraction operators to insert a Minister into a stream and extract a Minister from a stream. These operators
should call the write / read member functions in the class Minister.
istream& read(istream& in) : a mutator that reads from the stream in the data for the current object
w7_main Module (supplied)
}
Age: [USER TYPES HERE]
Id: [USER TYPES HERE]
District: [USER TYPES HERE]
Do not modify this module! Look at the code and make sure you understand it.
Reports TO: [USER TYPES HERE]
Year Assumed Office: [USER TYPES HERE]
// Workshop 7
// Version: 1.0
}
// Author: Asam Gulaid
// Description:
// This file tests your workshop 7
#include<iostream>
#include "Minister.h"
#include "Minister.h" // intentional
#include "MemberParliament.h"
#include "MemberParliament.h" // intentional
using namespace std;
using namespace sdds;
void printHeader (const char* title)
{
char oldFill = cout.fill('-');
cout.width(62);
cout <<
cout << ">
int main()
{
void newElections (Minister& aMinister, const char* district, const char* newPM, double year)
{
|| ||
cout.fill('-');
cout.width(62);
cout << << endl;
cout.fill(oldFill);
|| ||
aMinister.NewDistrict(district);
aMinister.changePM(newPM);
aMinister.assumeOffice (year);
cout << "Status of New Election " << aMinister << endl << endl;
<< endl;
printHeader("Person 1: MemberParliament");
e.md
11
MemberParliament mp("MP9403", 59);
cout << mp << endl << endl;
mp. NewDistrict ("Scarborough");
mp. NewDistrict("Mississauga");
mp. NewDistrict ("Huron County");
cout << endl << mp << endl << endl;
printHeader("Person 2: Read/Write");
cin >> mp;
cout << endl << mp << endl << endl;
<< title << endl;
printHeader("Person 3: Minister");
Minister aMinister("MP9573", 63, 2003, "Harper", "Pickering");
cout << endl;
newElections (aMinister, "Whitby", "Trudeau", 2007);
newElections (aMinister, "Kitchener", "Biden", 2010);
newElections (aMinister, "Oakville", "Trump", 2015);
Sample Output
printHeader("Person 4: Read/Write");
cin >> aMinister;
cout << endl << (MemberParliament)aMinister;
cout << endl << aMinister << endl << endl;
return 0;
| MP9403| |
MP9403
| MP9403|
|> Person 1: MemberParliament
Age: 40
Id: MP9566
| MP9403 | 59 | Unassigned
| MP9403 | 59 | Huron County
|> Person 2: Read/Write
District: Milton
| MP9573| |
| MP9566 | 40 | Milton
> Person 3: Minister
Age: 66
Id: MP9701
| MP9573| |
Pickering ---> Whitby
Status of New Election | MP9573 | 63 | Whitby | Trudeau/2007
Unassigned ---> Scarborough
Scarborough ---> Mississauga
Mississauga ---> Huron County
| MP9573| |
Kitchener ---> Oakville
Status of New Election | MP9573 | 63 | Oakville | Trump/2015
| MP9573| |
Whitby ---> Kitchener
Status of New Election | MP9573 | 63 | Kitchener | Biden/2010
Data Entry
> Person 4: Read/Write
Files to submit:
Unassigned ---> Pickering
District: Sudbury
Reports
TO: Obama
Year Assumed Office: 2021
MP9566
Milton
66
MP9701
Sudbury
Obama
2021
| MP9701 | 66 | Sudbury
| MP9701 | 66 | Sudbury | Obama/2021
MemberParliament.cpp
MemberParliament.h
Minister.cpp
Minister.h
main.cpp
|
|
Custom code submission
If you have any additional custom code, (i.e. functions, classes etc) that you want to reuse in this workshop save them under a module called
Utils (Utils.cpp and Utils.h) and submit them with your workshop using the instructions in the "Submitting Utils Module" section.
I
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 4 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
There are 6 errors.
Could you let me know how to fix those?

Transcribed Image Text:Error List
Entire Solution
Code
C4996
x
C4996
C4996
X 6 Errors
0 Warnings
0 Messages 97
Build + IntelliSense
Description
sucpy. This function of variable may be unsare. Consider using sucpy_s instead. To disable deprecation, use _CRI_SECURE_NO_WANINING. See online
help for details.
'strcpy': This function or variable may be unsafe. Consider using strcpy_s instead. To disable deprecation, use _CRT_SECURE_NO_WARNINGS. See online
help for details.
'strcpy': This function or variable may be unsafe. Consider using strcpy_s instead. To disable deprecation, use _CRT_SECURE_NO_WARNINGS. See online
help for details.
Project
WS07
WS07
WS07
Search Error List
Line Suppression State
MemberParliament.cpp 14
File
MemberParliament.cpp
Minister.cpp
20
12
<t
0
Solution
Recommended textbooks for you
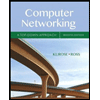
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
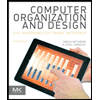
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
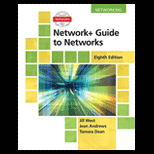
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
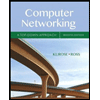
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
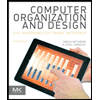
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
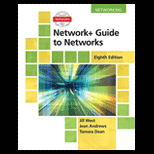
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
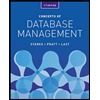
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
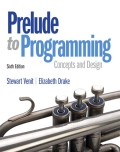
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
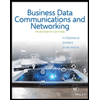
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY