es return expected outputs. Output Format Output a single line containing the fastest time to get to square N from square 1. Sample Input 0 5 2 1 4 1 3 Sample Output 0 6 Explanation 0
es return expected outputs. Output Format Output a single line containing the fastest time to get to square N from square 1. Sample Input 0 5 2 1 4 1 3 Sample Output 0 6 Explanation 0
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Information is present in the screenshot and below. Based on that need help in solving the code for this problem in python. The time complexity has to be as less as possible (nlogn or n at best, no n^2). Apply dynamic
Output Format
Output a single line containing the fastest time to get to square N from square 1.
Sample Input 0
5 2 1 4 1
3
Sample Output 0
6
Explanation 0
The optimal answer is to:
- RUN to square 2 (+2 seconds). Sonic now has 1 energy.
- DASH to square 3 (+1 second). Sonic now has 0 energy.
- RUN to square 4 (+2 seconds). Sonic now has 1 energy.
- DASH to square 5 (+1 second). Sonic now has 0 energy.
Total time is 6 seconds.
Sample Input 1
5 4 2 10 0
Sample Output 1
12
Explanation 1
The optimal answer is to:
- RUN to square 2 (+4 seconds). Sonic now has 1 energy.
- RUN to square 3 (+4 seconds). Sonic now has 2 energy.
- DASH to square 4 (+2 seconds). Sonic now has 1 energy.
- DASH to square 5 (+2 seconds). Sonic now has 0 energy.
Total time is 12 seconds.
Sample Input 2
7 1 5 0 2
1 2
Sample Output 2
6
The actual code
def solve(n,a,b,c,k,slippery): # compute and return answer here n, a, b, c, k = list(map(int,input().rstrip().split(" "))) slippery = [] if k == 0 else list(map(int,input().rstrip().split(" "))) print(solve(n,a,b,c,k,slippery)) |
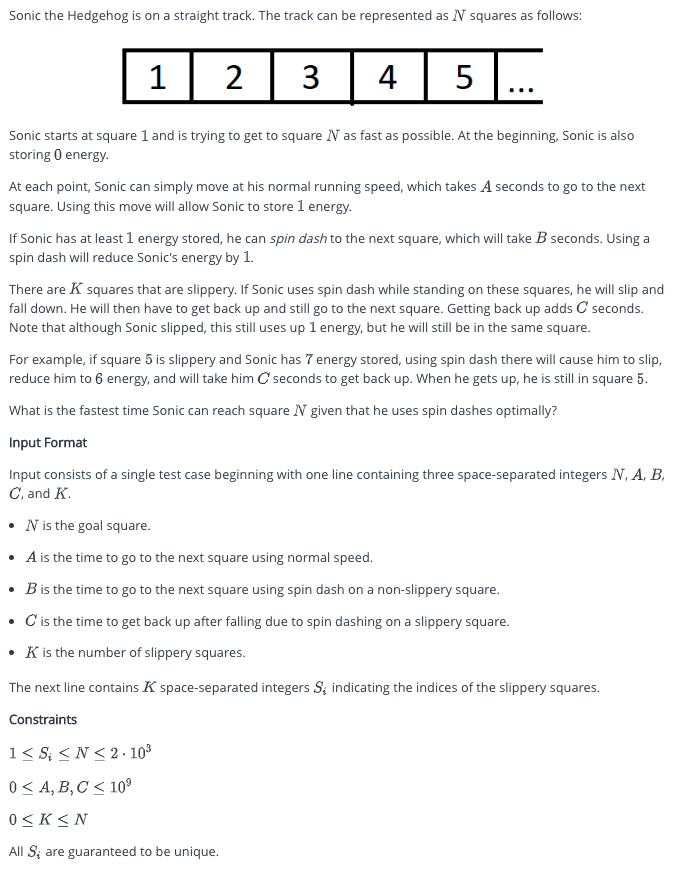
Transcribed Image Text:Sonic the Hedgehog is on a straight track. The track can be represented as N squares as follows:
1 2
3
4 5 |…....
Sonic starts at square 1 and is trying to get to square N as fast as possible. At the beginning, Sonic is also
storing 0 energy.
At each point, Sonic can simply move at his normal running speed, which takes A seconds to go to the next
square. Using this move will allow Sonic to store 1 energy.
If Sonic has at least 1 energy stored, he can spin dash to the next square, which will take B seconds. Using a
spin dash will reduce Sonic's energy by 1.
There are K squares that are slippery. If Sonic uses spin dash while standing on these squares, he will slip and
fall down. He will then have to get back up and still go to the next square. Getting back up adds C seconds.
Note that although Sonic slipped, this still uses up 1 energy, but he will still be in the same square.
For example, if square 5 is slippery and Sonic has 7 energy stored, using spin dash there will cause him to slip,
reduce him to 6 energy, and will take him C seconds to get back up. When he gets up, he is still in square 5.
What is the fastest time Sonic can reach square N given that he uses spin dashes optimally?
Input Format
Input consists of a single test case beginning with one line containing three space-separated integers N, A, B,
C, and K.
• N is the goal square.
• A is the time to go to the next square using normal speed.
• B is the time to go to the next square using spin dash on a non-slippery square.
• C is the time to get back up after falling due to spin dashing on a slippery square.
• K is the number of slippery squares.
The next line contains K space-separated integers S; indicating the indices of the slippery squares.
Constraints
1 ≤ S₂ ≤ N≤2.10³
0 < A, B, C < 10⁹
0<K<N
All S, are guaranteed to be unique.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 7 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
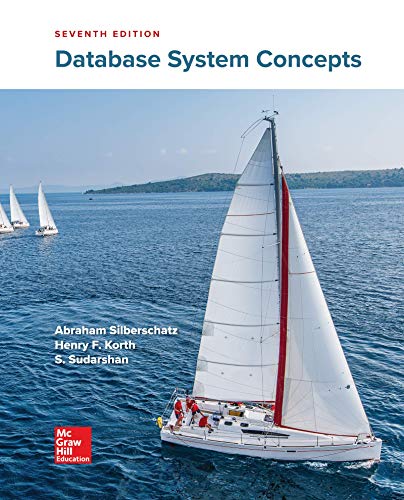
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
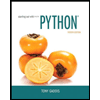
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
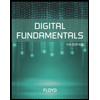
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
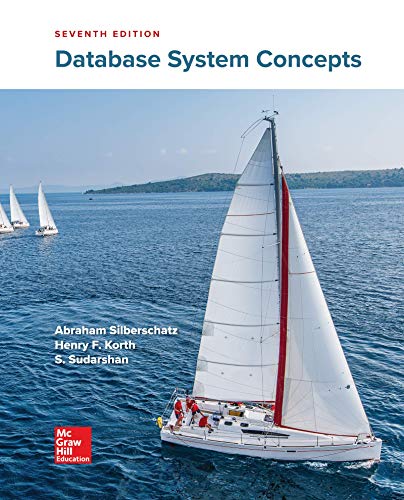
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
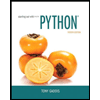
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
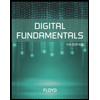
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
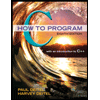
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
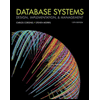
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
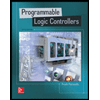
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education