Exercise #1: Write a C program that contains the following steps. Read carefully each step as they are not only programming steps but also learning topics that explain how numeric arrays in C work. Write a while loop to input a series of numbers (either from a file or from the keyboard, but using a file will make for easier debugging) into a one dimensional array of type double named x_arr, declared to be 25 elements in length. Count the elements as you input them. Then in a second loop, a for loop this time, print out all the elements which were input. Make up your own data file for this problem. Extend your code by writing a for loop to find the largest value in the array. The largest value should go into a variable named xhigh. Your code should then print out xhigh. Extend your code by writing a for loop to find the smallest value in the array. The smallest value should go into a variable named xlow. Your code should then print out xlow. Extend your code to produce a second array by using a for loop to iterate through your x_arr array and produce another array x_second_arr where each element in x_second_arr is 3 times the value in the array x_arr. Print out both arrays, with brief labelling so you can tell them apart on your output. You will next "normalize" the numbers in the array. This takes one array of numbers (x_arr) and puts them into a second "normalized" array named norm_x_arr so that the original values collectively "stretch" or "contract" to fit into a different range. (Both x_arr and norm_x_arr should be declared to be the same length, for example 25.) An example of where this could be used would be to take test results between 0 and 30 ("out of 30") and produce marks between 0 and 100. To do this, you will first input the two new limiting values you choose into two variables named max and min - these will be your upper and lower limits of the new, normalized norm_x_arr (the 0 and 100 of the example). You then copy the code you wrote above to find the largest and the smallest values in x_arr, putting them into xhigh and xlow (these would be the 0 and 30 of the example). Then using the appropriate loop form, run through the elements of x_arr, putting each element in turn into norm_x_arr modified according to the formula normxi = min + ((xi - xlow) * (max - min)) / (xhigh - xlow) to produce the second array norm_x_arr. In this formula the terms xi and normxi, are referring to single elements of the arrays x_arr and norm_x_arr. The xi will be be turned into the normxi but in the process they are adjusted so that xhigh becomes max, the maximum of all the normalized numbers, and xlow becomes min, the minimum of all the normalized numbers ... with all elements in the xi array being adjusted proprotionally to fall between min and max. As a trivial example, if you had the numbers 2 3 4 and you wanted to "normalize" these to between 0 and 10, you would apply the formula so that 2 3 4 would become 0 5 10. Note that the spacing between the values is maintained proportionally: 2 and 3 and 4 are all 1 apart; 0 and 5 and 10 are all 5 apart. As another example, you could also normalize the same 2 3 4 to be between 14 and 18. 2 would become 14, 3 would become 16, and 4 would become 18. Try this with several different sets of data. Include various orderings of the numbers (not necessarily given in sorted order), put in duplicate values for some of the data elements, and ensure your code works with a mix of positive, zero, and negative values.
Exercise #1: Write a C program that contains the following steps. Read carefully each step as they are not only programming steps but also learning topics that explain how numeric arrays in C work. Write a while loop to input a series of numbers (either from a file or from the keyboard, but using a file will make for easier debugging) into a one dimensional array of type double named x_arr, declared to be 25 elements in length. Count the elements as you input them. Then in a second loop, a for loop this time, print out all the elements which were input. Make up your own data file for this problem. Extend your code by writing a for loop to find the largest value in the array. The largest value should go into a variable named xhigh. Your code should then print out xhigh. Extend your code by writing a for loop to find the smallest value in the array. The smallest value should go into a variable named xlow. Your code should then print out xlow. Extend your code to produce a second array by using a for loop to iterate through your x_arr array and produce another array x_second_arr where each element in x_second_arr is 3 times the value in the array x_arr. Print out both arrays, with brief labelling so you can tell them apart on your output. You will next "normalize" the numbers in the array. This takes one array of numbers (x_arr) and puts them into a second "normalized" array named norm_x_arr so that the original values collectively "stretch" or "contract" to fit into a different range. (Both x_arr and norm_x_arr should be declared to be the same length, for example 25.) An example of where this could be used would be to take test results between 0 and 30 ("out of 30") and produce marks between 0 and 100. To do this, you will first input the two new limiting values you choose into two variables named max and min - these will be your upper and lower limits of the new, normalized norm_x_arr (the 0 and 100 of the example). You then copy the code you wrote above to find the largest and the smallest values in x_arr, putting them into xhigh and xlow (these would be the 0 and 30 of the example). Then using the appropriate loop form, run through the elements of x_arr, putting each element in turn into norm_x_arr modified according to the formula normxi = min + ((xi - xlow) * (max - min)) / (xhigh - xlow) to produce the second array norm_x_arr. In this formula the terms xi and normxi, are referring to single elements of the arrays x_arr and norm_x_arr. The xi will be be turned into the normxi but in the process they are adjusted so that xhigh becomes max, the maximum of all the normalized numbers, and xlow becomes min, the minimum of all the normalized numbers ... with all elements in the xi array being adjusted proprotionally to fall between min and max. As a trivial example, if you had the numbers 2 3 4 and you wanted to "normalize" these to between 0 and 10, you would apply the formula so that 2 3 4 would become 0 5 10. Note that the spacing between the values is maintained proportionally: 2 and 3 and 4 are all 1 apart; 0 and 5 and 10 are all 5 apart. As another example, you could also normalize the same 2 3 4 to be between 14 and 18. 2 would become 14, 3 would become 16, and 4 would become 18. Try this with several different sets of data. Include various orderings of the numbers (not necessarily given in sorted order), put in duplicate values for some of the data elements, and ensure your code works with a mix of positive, zero, and negative values.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter8: I/o Streams And Data Files
Section: Chapter Questions
Problem 8PP: (Data processing) A bank’s customer records are to be stored in a file and read into a set of arrays...
Related questions
Question
Exercise #1:
Write a C program that contains the following steps. Read carefully each step as they are not only programming steps but also learning topics that explain how numeric arrays in C work.
- Write a while loop to input a series of numbers (either from a file or from the keyboard, but using a file will make for easier debugging) into a one dimensional array of type double named x_arr, declared to be 25 elements in length. Count the elements as you input them. Then in a second loop, a for loop this time, print out all the elements which were input. Make up your own data file for this problem.
- Extend your code by writing a for loop to find the largest value in the array. The largest value should go into a variable named xhigh. Your code should then print out xhigh.
- Extend your code by writing a for loop to find the smallest value in the array. The smallest value should go into a variable named xlow. Your code should then print out xlow.
- Extend your code to produce a second array by using a for loop to iterate through your x_arr array and produce another array x_second_arr where each element in x_second_arr is 3 times the value in the array x_arr. Print out both arrays, with brief labelling so you can tell them apart on your output.
- You will next "normalize" the numbers in the array. This takes one array of numbers (x_arr) and puts them into a second "normalized" array named norm_x_arr so that the original values collectively "stretch" or "contract" to fit into a different range. (Both x_arr and norm_x_arr should be declared to be the same length, for example 25.) An example of where this could be used would be to take test results between 0 and 30 ("out of 30") and produce marks between 0 and 100. To do this, you will first input the two new limiting values you choose into two variables named max and min - these will be your upper and lower limits of the new, normalized norm_x_arr (the 0 and 100 of the example). You then copy the code you wrote above to find the largest and the smallest values in x_arr, putting them into xhigh and xlow (these would be the 0 and 30 of the example). Then using the appropriate loop form, run through the elements of x_arr, putting each element in turn into norm_x_arr modified according to the formula
normxi = min + ((xi - xlow) * (max - min)) / (xhigh - xlow)
to produce the second array norm_x_arr. In this formula the terms xi and normxi, are referring to single elements of the arrays x_arr and norm_x_arr. The xi will be be turned into the normxi but in the process they are adjusted so that xhigh becomes max, the maximum of all the normalized numbers, and xlow becomes min, the minimum of all the normalized numbers ... with all elements in the xi array being adjusted proprotionally to fall between min and max. As a trivial example, if you had the numbers 2 3 4 and you wanted to "normalize" these to between 0 and 10, you would apply the formula so that 2 3 4 would become 0 5 10. Note that the spacing between the values is maintained proportionally: 2 and 3 and 4 are all 1 apart; 0 and 5 and 10 are all 5 apart. As another example, you could also normalize the same 2 3 4 to be between 14 and 18. 2 would become 14, 3 would become 16, and 4 would become 18. Try this with several different sets of data. Include various orderings of the numbers (not necessarily given in sorted order), put in duplicate values for some of the data elements, and ensure your code works with a mix of positive, zero, and negative values.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
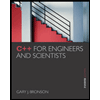
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
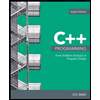
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
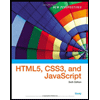
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
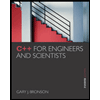
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
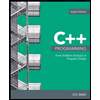
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
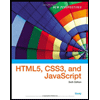
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning