Exercise - Functions and Optionals If an app asks for a user's age, it may be because the app requires a user to be over a certain age to use some of the services it provides. Write a function called checkAge that takes one parameter of type String. The function should try to convert this parameter into an Int value and then check if the user is over 18 years old. If he/she is old enough, print "Welcome!", otherwise print "Sorry, but you aren't old enough to use our app." If the String parameter cannot be converted into an Int value, print "Sorry, something went wrong. Can you please re-enter your age?" Call the function and pass in userInputAge below as the single parameter. Then call the function and pass in a string that can be converted to an integer. let userInputAge: String = "34e" Go back and update your function to return the age as an integer. Will your function always return a value? Make sure your return type accurately reflects this. Call the function and print the return value. Imagine you are creating an app for making purchases. Write a function that will take in the name of an item for purchase as a String and will return the cost of that item as an optional Double. In the body of the function, check to see if the item is in stock by accessing it in the dictionary stock. If it is, return the price of the item by accessing it in the dictionary prices. If the item is out of stock, return nil. Call the function and pass in a String that exists in the dictionaries below. Print the return value. 3 var prices = ["Chips": 2.99, "Donuts": 1.89, "Juice": 3.99, "Apple": 0.50, "Banana": 0.25, "Broccoli": e.99] var stock = ["Chips": 4, "Donuts": 0, "Juice": 12, "Apple": 6, "Banana": 6, "Broccoli": 3]
Exercise - Functions and Optionals If an app asks for a user's age, it may be because the app requires a user to be over a certain age to use some of the services it provides. Write a function called checkAge that takes one parameter of type String. The function should try to convert this parameter into an Int value and then check if the user is over 18 years old. If he/she is old enough, print "Welcome!", otherwise print "Sorry, but you aren't old enough to use our app." If the String parameter cannot be converted into an Int value, print "Sorry, something went wrong. Can you please re-enter your age?" Call the function and pass in userInputAge below as the single parameter. Then call the function and pass in a string that can be converted to an integer. let userInputAge: String = "34e" Go back and update your function to return the age as an integer. Will your function always return a value? Make sure your return type accurately reflects this. Call the function and print the return value. Imagine you are creating an app for making purchases. Write a function that will take in the name of an item for purchase as a String and will return the cost of that item as an optional Double. In the body of the function, check to see if the item is in stock by accessing it in the dictionary stock. If it is, return the price of the item by accessing it in the dictionary prices. If the item is out of stock, return nil. Call the function and pass in a String that exists in the dictionaries below. Print the return value. 3 var prices = ["Chips": 2.99, "Donuts": 1.89, "Juice": 3.99, "Apple": 0.50, "Banana": 0.25, "Broccoli": e.99] var stock = ["Chips": 4, "Donuts": 0, "Juice": 12, "Apple": 6, "Banana": 6, "Broccoli": 3]
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Functions and Optionals - How do I do this practice exercise using Swift code?
![Exercise - Functions and Optionals
If an app asks for a user's age, it may be because the app requires a user to be over a certain age to use some of the services it provides. Write a function called checkAge that takes one parameter
of type String. The function should try to convert this parameter into an Int value and then check if the user is over 18 years old. If he/she is old enough, print "Welcome!", otherwise print
"Sorry, but you aren't old enough to use our app." If the String parameter cannot be converted into an Int value, print "Sorry, something went wrong. Can you please re-enter your age?" Call the
function and pass in userInputAge below as the single parameter. Then call the function and pass in a string that can be converted to an integer.
let userInputAge: String
= "34e"
7
8
Go back and update your function to return the age as an integer. Will your function always return a value? Make sure your return type accurately reflects this. Call the function and print the
return value.
10
11
Imagine you are creating an app for making purchases. Write a function that will take in the name of an item for purchase as a String and will return the cost of that item as an optional Double.
In the body of the function, check to see if the item is in stock by accessing it in the dictionary stock. If it is, return the price of the item by accessing it in the dictionary prices. If the item is
out of stock, return nil. Call the function and pass in a String that exists in the dictionaries below. Print the return value.
["Chips": 2.99, "Donuts": 1.89, "Juice": 3.99, "Apple": 0.50, "Banana": 0.25, "Broccoli": 0.99]
["Chips": 4, "Donuts": 0, "Juice": 12, "Apple": 6, "Banana": 6, "Broccoli": 3]
13
var prices
%3D
14
var stock =
15
16 |](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbea0901b-9b71-43dc-90d5-d9e10ed3de89%2Fe16d82af-6a6e-48f1-bc9c-5c6c25ad4628%2Fz7z65o_processed.png&w=3840&q=75)
Transcribed Image Text:Exercise - Functions and Optionals
If an app asks for a user's age, it may be because the app requires a user to be over a certain age to use some of the services it provides. Write a function called checkAge that takes one parameter
of type String. The function should try to convert this parameter into an Int value and then check if the user is over 18 years old. If he/she is old enough, print "Welcome!", otherwise print
"Sorry, but you aren't old enough to use our app." If the String parameter cannot be converted into an Int value, print "Sorry, something went wrong. Can you please re-enter your age?" Call the
function and pass in userInputAge below as the single parameter. Then call the function and pass in a string that can be converted to an integer.
let userInputAge: String
= "34e"
7
8
Go back and update your function to return the age as an integer. Will your function always return a value? Make sure your return type accurately reflects this. Call the function and print the
return value.
10
11
Imagine you are creating an app for making purchases. Write a function that will take in the name of an item for purchase as a String and will return the cost of that item as an optional Double.
In the body of the function, check to see if the item is in stock by accessing it in the dictionary stock. If it is, return the price of the item by accessing it in the dictionary prices. If the item is
out of stock, return nil. Call the function and pass in a String that exists in the dictionaries below. Print the return value.
["Chips": 2.99, "Donuts": 1.89, "Juice": 3.99, "Apple": 0.50, "Banana": 0.25, "Broccoli": 0.99]
["Chips": 4, "Donuts": 0, "Juice": 12, "Apple": 6, "Banana": 6, "Broccoli": 3]
13
var prices
%3D
14
var stock =
15
16 |
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
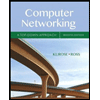
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
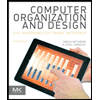
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
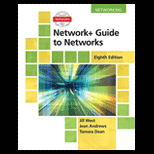
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
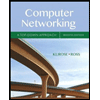
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
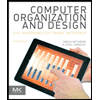
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
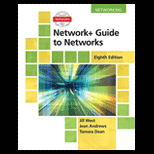
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
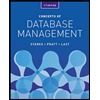
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
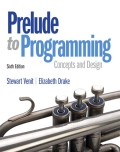
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
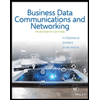
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY