Exercise: Write a program to compute the result of dividing two positive integers and the remainder using only addition and subtraction operations (no / or %). The program should execute as in the examples below. Input: two positive integers Output example: Enter two integers: 7 3 7/3 = 2+1/3 Enter two integers: 9 0 9/0 = undefined Enter two integers: 15 3 15/3 = 5 Algorithm: 1. Define a function with two integer input parameters called top and bot. Inside the function: A. Define integers quot and rem, initialized to 0 and top, respectively. B. Build a loop that executes as long as bot is less than or equal to rem with the following: 1) Add 1 to quot 2) subtract bot from rem C. After the loop, write top, bot, quot and rem to the standard output as shown in the example. 2. In main, read the integers to be divided (see example) 3. Call the function to compute and print the division.
Exercise: Write a program to compute the result of dividing two positive integers and the remainder using only addition and subtraction operations (no / or %). The program should execute as in the examples below. Input: two positive integers Output example: Enter two integers: 7 3 7/3 = 2+1/3 Enter two integers: 9 0 9/0 = undefined Enter two integers: 15 3 15/3 = 5 Algorithm: 1. Define a function with two integer input parameters called top and bot. Inside the function: A. Define integers quot and rem, initialized to 0 and top, respectively. B. Build a loop that executes as long as bot is less than or equal to rem with the following: 1) Add 1 to quot 2) subtract bot from rem C. After the loop, write top, bot, quot and rem to the standard output as shown in the example. 2. In main, read the integers to be divided (see example) 3. Call the function to compute and print the division.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section6.4: A Case Study: Rectangular To Polar Coordinate Conversion
Problem 9E: (Numerical) Write a program that tests the effectiveness of the rand() library function. Start by...
Related questions
Question
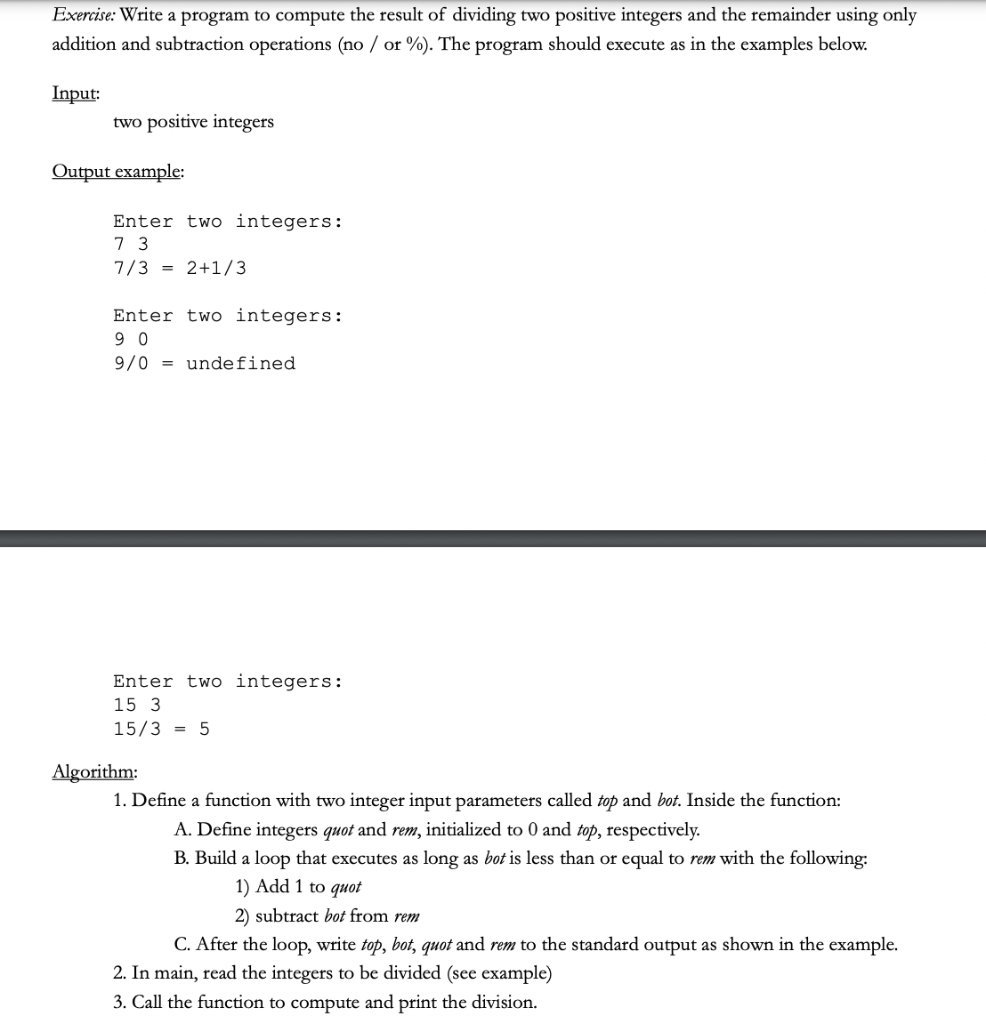
Transcribed Image Text:Exercise: Write a program to compute the result of dividing two positive integers and the remainder using only
addition and subtraction operations (no / or %). The program should execute as in the examples below.
Input:
two positive integers
Output example:
Enter two integers:
7 3
7/3 = 2+1/3
Enter two integers:
9 0
9/0 = undefined
Enter two integers:
15 3
15/3 = 5
Algorithm:
1. Define a function with two integer input parameters called top and bot. Inside the function:
A. Define integers quot and rem, initialized to 0 and top, respectively.
B. Build a loop that executes as long as bot is less than or equal to rem with the following:
1) Add 1 to quot
2) subtract bot from rem
C. After the loop, write top, bot, quot and rem to the standard output as shown in the example.
2. In main, read the integers to be divided (see example)
3. Call the function to compute and print the division.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
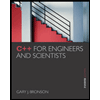
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
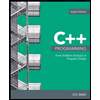
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
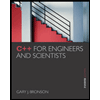
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
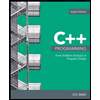
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning