Explain these codes import java.text.DecimalFormat; import java.util.Scanner; public class Main { private static DecimalFormat df2 = new DecimalFormat("#.00"); public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.println(); System.out.print("\tEnter Name : "); String emp_name =input.nextLine(); System.out.print("\tPress F for Full Time or P for Part Time : "); char job_criteria =input.next().charAt(0); char select = Character.toUpperCase(job_criteria); System.out.println(); if (select == 'F') { System.out.print("\t------ Full Time Employee ----- "); System.out.println(); System.out.print("\tEnter Basic Pay : "); double basic_pay = input.nextDouble(); FullTimeEmployee emp = new FullTimeEmployee(); emp.setName(emp_name); emp.setMonthlySalary(basic_pay); System.out.println("\n"); System.out.println("\tName : " + emp.getName()); System.out.println("\tWage : " + df2.format(emp.getMonthlySalary())); System.out.println("\n"); } else if (select == 'P') { System.out.print("\t------ Part Time Employee ----- "); System.out.println("\n"); System.out.print("\tEnter Rate Per Hour and No. of Hours Worked Seperated By Space : "); double rate_per_hour = input.nextDouble(); int no_hours_work2 = input.nextInt(); PartTimeEmployee emp = new PartTimeEmployee(); emp.setName(emp_name); emp.setRatePerHour(rate_per_hour); emp.setHoursWorked(no_hours_work2); System.out.println("\tName : " + emp.getName()); System.out.println("\tWage : " + df2.format(emp.getWage())); System.out.println("\n"); } else { System.out.println("\n"); System.out.print("\tInvalid Option. Please Try Again"); } System.out.print("\tEnd of Program"); System.out.println("\n"); input.close(); } } class Employee { private String name; public void setName(String name) { this.name = name; } public String getName() { return name; } } class PartTimeEmployee extends Employee { private double ratePerHour; private int hoursWorked; private double wage; public void setWage() { wage = hoursWorked * ratePerHour; } public double getWage() { setWage(); return wage; } public void setRatePerHour(double rate) { ratePerHour = rate; } public void setHoursWorked(int hours) { hoursWorked = hours; } } class FullTimeEmployee extends Employee { private double monthlySalary; public void setMonthlySalary(double salary) { monthlySalary = salary; } public double getMonthlySalary() { return monthlySalary; } }
Explain these codes
import java.text.DecimalFormat;
import java.util.Scanner;
public class Main
{
private static DecimalFormat df2 = new DecimalFormat("#.00");
public static void main(String[] args)
{
Scanner input = new Scanner(System.in);
System.out.println();
System.out.print("\tEnter Name : ");
String emp_name =input.nextLine();
System.out.print("\tPress F for Full Time or P for Part Time : ");
char job_criteria =input.next().charAt(0);
char select = Character.toUpperCase(job_criteria);
System.out.println();
if (select == 'F') {
System.out.print("\t------ Full Time Employee ----- ");
System.out.println();
System.out.print("\tEnter Basic Pay : ");
double basic_pay = input.nextDouble();
FullTimeEmployee emp = new FullTimeEmployee();
emp.setName(emp_name);
emp.setMonthlySalary(basic_pay);
System.out.println("\n");
System.out.println("\tName : " + emp.getName());
System.out.println("\tWage : " + df2.format(emp.getMonthlySalary()));
System.out.println("\n");
}
else if (select == 'P')
{
System.out.print("\t------ Part Time Employee ----- ");
System.out.println("\n");
System.out.print("\tEnter Rate Per Hour and No. of Hours Worked Seperated By Space : ");
double rate_per_hour = input.nextDouble();
int no_hours_work2 = input.nextInt();
PartTimeEmployee emp = new PartTimeEmployee();
emp.setName(emp_name);
emp.setRatePerHour(rate_per_hour);
emp.setHoursWorked(no_hours_work2);
System.out.println("\tName : " + emp.getName());
System.out.println("\tWage : " + df2.format(emp.getWage()));
System.out.println("\n");
} else {
System.out.println("\n");
System.out.print("\tInvalid Option. Please Try Again");
}
System.out.print("\tEnd of Program");
System.out.println("\n");
input.close();
}
}
class Employee
{
private String name;
public void setName(String name)
{
this.name = name;
}
public String getName()
{
return name;
}
}
class PartTimeEmployee extends Employee
{
private double ratePerHour;
private int hoursWorked;
private double wage;
public void setWage()
{
wage = hoursWorked * ratePerHour;
}
public double getWage()
{
setWage();
return wage;
}
public void setRatePerHour(double rate)
{
ratePerHour = rate;
}
public void setHoursWorked(int hours)
{
hoursWorked = hours;
}
}
class FullTimeEmployee extends Employee
{
private double monthlySalary;
public void setMonthlySalary(double salary)
{
monthlySalary = salary;
}
public double getMonthlySalary()
{
return monthlySalary;
}
}

Step by step
Solved in 4 steps with 1 images

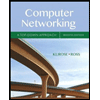
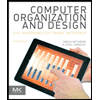
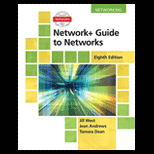
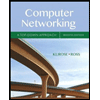
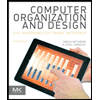
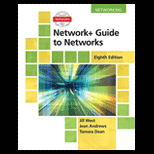
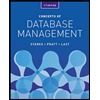
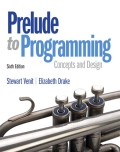
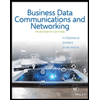