Extend the code from Lab. Use the same UML as below and make extensions as necessary Circle -int x //x coord of the center -int y // y coord of the center -int radius -static int count // static variable to keep count of number of circles created + Circle() //default constructor that sets origin to (0,0) and radius to 1 +Circle(int x, int y, int radius) // regular constructor +getX(): int +getY(): int +getRadius(): int +setX(int newX: void +setY(int newY): void +setRadius(int newRadius):void +getArea(): double // returns the area using formula pi*r^2 +getCircumference // returns the circumference using the formula 2*pi*r +toString(): String // return the circle as a string in the form (x,y) : radius +getDistance(Circle other): double // * returns the distance between the center of this circle and the other circle +moveTo(int newX,int newY):void // * move the center of the circle to the new coordinates +intersects(Circle other): bool //* returns true if the center of the other circle lies inside this circle else returns false +resize(double scale):void// * multiply the radius by the scale +resize(int scale):Circle // * returns a new Circle with the same center as this circle but radius multiplied by scale +getCount():int //returns the number of circles created //note that the resize function is an overloaded function. The definitions have different signatures Write a brand new driver program that does the following: Creates a circle object circleOne at (0,0):5. Creates a shared pointer circleOnePtr to circleOne Creates a circle object circleTwo at (-2,-2): 10 Creates a shared pointer circleTwoPtr to circleTwo Use greaterThan to check if circleTwo is bigger than circleOne and display results // **** you will need to change the parameter to shared pointer Declare a vector of pointers to circles- circlePointerVector Call a function with signature inputData(circlePointerVector &, string filename) that reads data from a file called dataLab4.txt into the array The following h-k are done in this function // **** note how the & operator is used in the parameter list Use istringstream to create an input string stream called instream. Initialize it with each string that is read from the data file using the getline method. Read the coordinates for the center and the radius from instream to create the circles Include a try catch statement to take care of the exception that would occur if there was a file open error. Display the message “File Open Error” and exit if the exception occurs Display all the circles in this vector of shared pointers using the toString method Display the sum of the areas of all the circles in the array Switch the position of the second and fourth circles by swapping pointers to the circles Display this changed sequence of circles using toString method You need to go through it and learn how to use shared pointers to objects, create vectors of shared pointers to objects and pass these as parameters. You need to write one line of code. Declare circleTwoPtr. Watch for **** in comments. Data File: 0 0 4 0 0 12 - 2 -9 11 4 5 7 7 8 9 2 -5 11 the output need to look like screenshot
Extend the code from Lab. Use the same UML as below and make extensions as necessary
Circle |
-int x //x coord of the center -int y // y coord of the center -int radius -static int count // static variable to keep count of number of circles created |
+ Circle() //default constructor that sets origin to (0,0) and radius to 1 +Circle(int x, int y, int radius) // regular constructor +getX(): int +getY(): int +getRadius(): int +setX(int newX: void +setY(int newY): void +setRadius(int newRadius):void +getArea(): double // returns the area using formula pi*r^2 +getCircumference // returns the circumference using the formula 2*pi*r +toString(): String // return the circle as a string in the form (x,y) : radius +getDistance(Circle other): double // * returns the distance between the center of this circle and the other circle +moveTo(int newX,int newY):void // * move the center of the circle to the new coordinates +intersects(Circle other): bool //* returns true if the center of the other circle lies inside this circle else returns false +resize(double scale):void// * multiply the radius by the scale +resize(int scale):Circle // * returns a new Circle with the same center as this circle but radius multiplied by scale +getCount():int //returns the number of circles created //note that the resize function is an overloaded function. The definitions have different signatures
|
- Write a brand new driver program that does the following:
- Creates a circle object circleOne at (0,0):5.
- Creates a shared pointer circleOnePtr to circleOne
- Creates a circle object circleTwo at (-2,-2): 10
- Creates a shared pointer circleTwoPtr to circleTwo
- Use greaterThan to check if circleTwo is bigger than circleOne and display results // **** you will need to change the parameter to shared pointer
- Declare a vector of pointers to circles- circlePointerVector
- Call a function with signature inputData(circlePointerVector &, string filename) that reads data from a file called dataLab4.txt into the array The following h-k are done in this function
// **** note how the & operator is used in the parameter list
- Use istringstream to create an input string stream called instream. Initialize it with each string that is read from the data file using the getline method.
- Read the coordinates for the center and the radius from instream to create the circles
- Include a try catch statement to take care of the exception that would occur if there was a file open error. Display the message “File Open Error” and exit if the exception occurs
- Display all the circles in this vector of shared pointers using the toString method
- Display the sum of the areas of all the circles in the array
- Switch the position of the second and fourth circles by swapping pointers to the circles
- Display this changed sequence of circles using toString method
You need to go through it and learn how to use shared pointers to objects, create
You need to write one line of code. Declare circleTwoPtr. Watch for **** in comments.
Data File:
0 0 4
0 0 12
- 2 -9 11
4 5 7
7 8 9
2 -5 11
the output need to look like screenshot
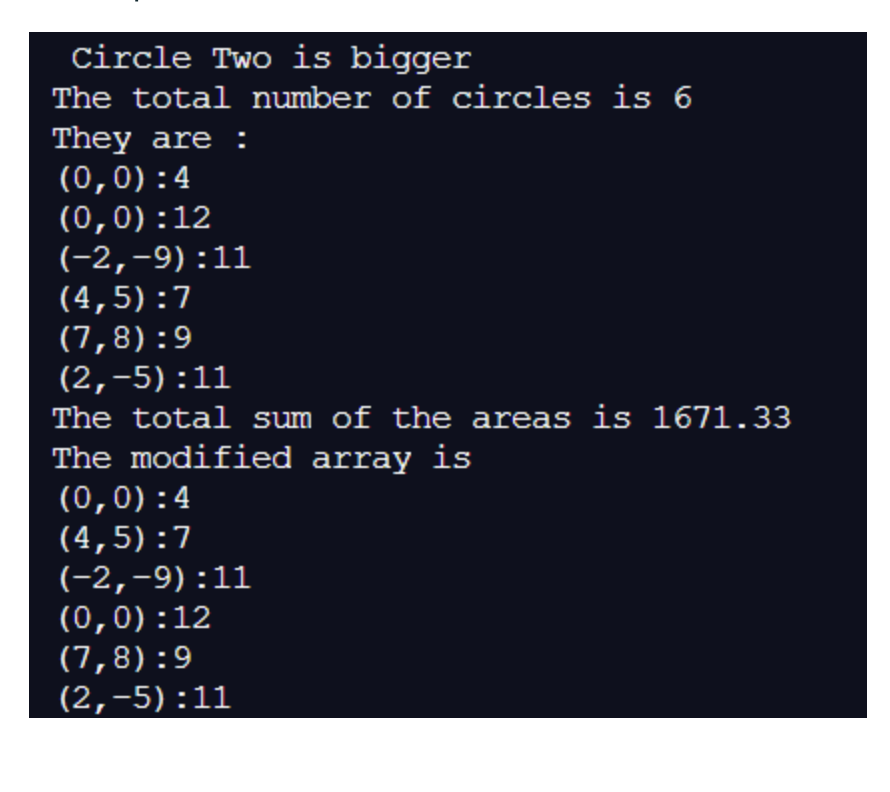

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 7 images

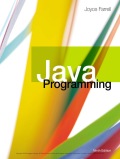
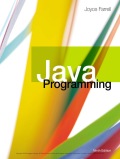